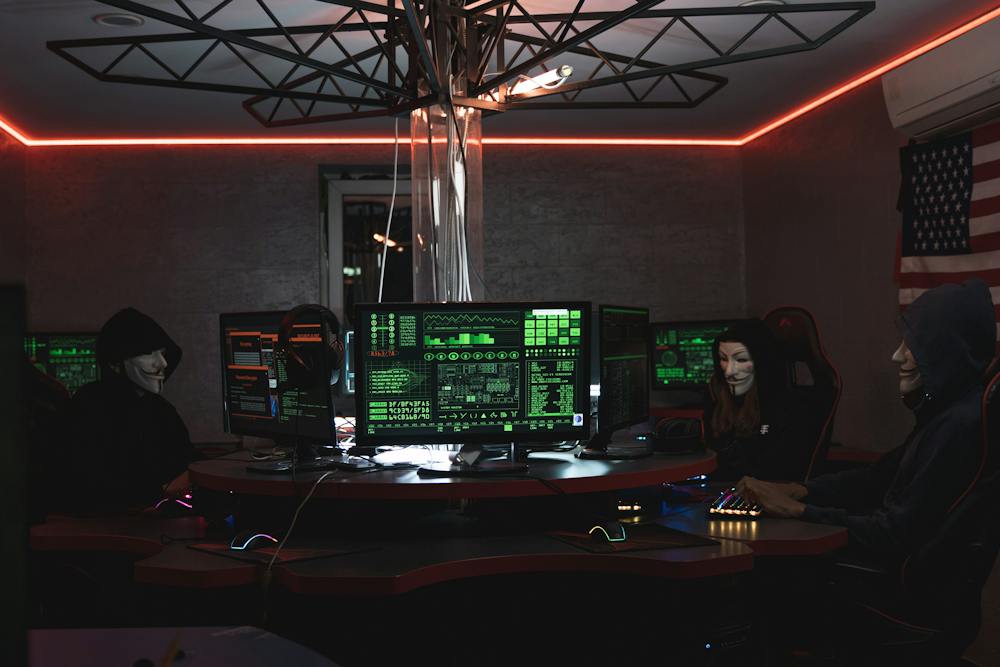
Handling HTTP requests is a crucial part of web development. One popular way to send HTTP requests is by using cURL in PHP. cURL is a powerful library that allows you to interact with various types of servers using various protocols. In this tutorial, we will walk you through the process of making cURL requests in PHP.
Prerequisites
Before we get started, make sure you have the following:
- Basic knowledge of PHP
- A local development environment such as XAMPP or WAMP
Step 1: Setting up your Environment
First, ensure that you have PHP and cURL installed on your local development environment. If not, you can easily install cURL using the following command:
sudo apt-get install php-curl
Once you have cURL installed, you can verify IT by running the following command in your terminal or command prompt:
php -m | grep curl
Step 2: Making a Simple cURL Request
Now that your environment is set up, let’s make a simple cURL request to an external API. Open your text editor and create a new PHP file. Add the following code:
<?php
// create a new cURL resource
$ch = curl_init();
// set the cURL options
curl_setopt($ch, CURLOPT_URL, 'https://api.example.com/data');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// execute the cURL request
$response = curl_exec($ch);
// close cURL resource
curl_close($ch);
// handle the response
if ($response) {
$data = json_decode($response, true);
var_dump($data);
} else {
echo 'Error: ' . curl_error($ch);
}
Step 3: Sending POST Request with cURL
cURL also allows you to send POST requests to a server. Let’s modify our previous example to send a POST request to an API endpoint.
<?php
$ch = curl_init();
$data = array('username' => 'john', 'password' => 'secret');
curl_setopt($ch, CURLOPT_URL, 'https://api.example.com/login');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query($data));
$response = curl_exec($ch);
curl_close($ch);
if ($response) {
$result = json_decode($response, true);
var_dump($result);
} else {
echo 'Error: ' . curl_error($ch);
}
Step 4: Handling cURL Errors
cURL requests may sometimes fail due to various reasons such as network issues or server errors. It’s important to handle these errors gracefully to provide a better user experience. Here’s how you can handle cURL errors:
<?php
$ch = curl_init();
// ...
$response = curl_exec($ch);
if ($response) {
// handle successful response
} else {
// handle cURL error
$error_message = curl_error($ch);
if ($error_message) {
echo 'Error: ' . $error_message;
} else {
echo 'Error: Unknown cURL error';
}
}
// ...
Conclusion
Now you have learned how to make cURL requests in PHP. cURL is a versatile tool that allows you to interact with various web services and APIs. We have covered the basics of making simple GET and POST requests and handling cURL errors. With this knowledge, you can start integrating cURL into your PHP projects and enhance their capabilities.
FAQs
Q: What is cURL?
A: cURL is a library and command-line tool for transferring data with URLs. It supports various protocols such as HTTP, HTTPS, FTP, and more.
Q: Can I use cURL in other programming languages?
A: Yes, cURL is a popular library and is available in various programming languages including PHP, Python, and Node.js.
Q: How to handle authentication with cURL?
A: You can handle authentication with cURL by using the CURLOPT_USERPWD
option to set the username and password for the request.
Q: Is cURL secure?
A: cURL itself is not inherently secure, but it provides the capability to make secure connections using protocols like HTTPS. It’s important to use cURL securely by validating SSL certificates and handling sensitive data appropriately.
Q: Can I make asynchronous requests with cURL?
A: cURL itself doesn’t support asynchronous requests, but you can achieve asynchronous behavior by using techniques such as multi_curl or by using cURL in combination with other asynchronous libraries or frameworks.