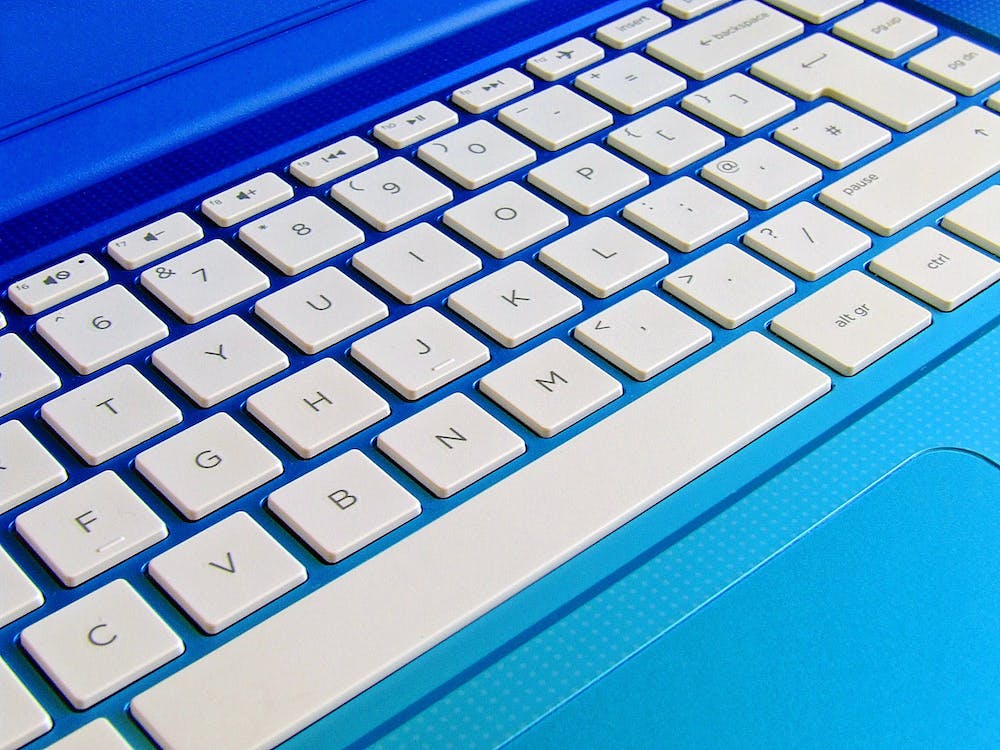
PHP is a popular programming language for web development, and IT is commonly used to create dynamic web pages. When working with PHP, you may need to retrieve usernames from a database to display on your Website. In this step-by-step guide, we will show you how to get usernames from a PHP database.
Step 1: Connecting to the Database
The first step is to establish a connection to the database using PHP. You will need to provide the database host, username, password, and database name to create the connection. Here is an example of how to connect to a MySQL database using PHP:
<?php
$host = "localhost";
$username = "username";
$password = "password";
$database = "database_name";
$connection = new mysqli($host, $username, $password, $database);
if ($connection->connect_error) {
die("Connection failed: " . $connection->connect_error);
}
?>
Step 2: Retrieving Usernames from the Database
Once the connection to the database is established, you can retrieve the usernames from the database using SQL queries. You can use the SELECT statement to fetch the usernames from a specific table in the database. Here is an example of how to retrieve usernames from a table named `users`:
<?php
$query = "SELECT username FROM users";
$result = $connection->query($query);
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo "Username: " . $row["username"] . "<br>";
}
} else {
echo "0 results";
}
$connection->close();
?>
Step 3: Displaying the Usernames on a Web Page
Once the usernames are retrieved from the database, you can display them on a web page using PHP. You can use the echo statement to output the usernames in a list or a table. Here is an example of how to display the usernames in an unordered list:
<?php
echo "<ul>";
$query = "SELECT username FROM users";
$result = $connection->query($query);
if ($result->num_rows > 0) {
while($row = $result->fetch_assoc()) {
echo "<li>" . $row["username"] . "</li>";
}
} else {
echo "0 results";
}
echo "</ul>";
$connection->close();
?>
Conclusion
In conclusion, retrieving usernames from a PHP database involves connecting to the database, retrieving the usernames using SQL queries, and displaying the usernames on a web page. By following the step-by-step guide provided in this article, you can easily get usernames from a PHP database and incorporate them into your web development projects.
FAQs
Q: Can I use this method to retrieve other data from the database?
A: Yes, you can use similar techniques to retrieve other data such as email addresses, user IDs, and more from a PHP database.
Q: Is it important to close the database connection after retrieving the data?
A: Yes, it is good practice to close the database connection after you have finished retrieving the data to free up resources and avoid potential issues with the database.
Q: What are the security considerations when retrieving data from a database?
A: When retrieving data from a database, it is important to sanitize user input to prevent SQL injection attacks. You can use prepared statements to help prevent these types of attacks.