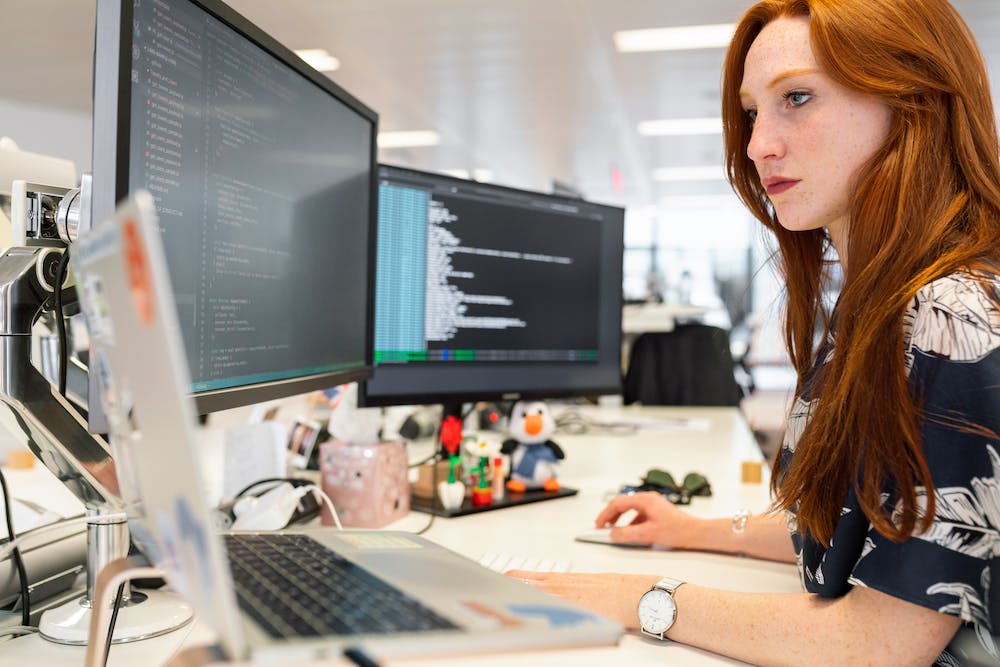
PHP Mailer is a powerful library that allows developers to send emails using the PHP programming language. IT provides a complete solution for managing and sending emails, making IT an essential tool for any web application that requires email communication. In this article, we will explore how to send emails with PHP Mailer, covering everything from installation to advanced usage.
Installation
The first step to start using PHP Mailer is to download and install IT. The library can be easily installed using Composer, a dependency manager for PHP. Open your command line interface and move to your project’s root directory. Run the following command to install PHP Mailer:
composer require phpmailer/phpmailer
This command will download PHP Mailer and its dependencies into your project’s vendor directory. Once the installation is complete, you can start using PHP Mailer in your project.
Configuration
To configure PHP Mailer, you need to initialize an instance of the PHPMailer class and set up its properties. Here’s an example of a basic configuration:
$mail = new PHPMailer\PHPMailer\PHPMailer();
$mail->isSMTP();
$mail->Host = 'smtp.gmail.com';
$mail->Port = 587;
$mail->SMTPAuth = true;
$mail->Username = '[email protected]';
$mail->Password = 'your-password';
In this example, we’re configuring PHP Mailer to use SMTP (Simple Mail Transfer Protocol) to send emails. We specify the SMTP server (in this case, Gmail’s SMTP server), the port, and enable SMTP authentication by providing our email credentials.
Sending Emails
Once you have configured PHP Mailer, you can start sending emails. Here’s an example of sending a simple text email:
$mail->setFrom('[email protected]', 'Your Name');
$mail->addAddress('[email protected]', 'Recipient Name');
$mail->Subject = 'Hello from PHP Mailer!';
$mail->Body = 'This is the body of the email.';
$mail->send();
In this example, we set the sender’s email address and name using the setFrom()
method, and the recipient’s email address and name using the addAddress()
method. We also set the subject and body of the email. Finally, we call the send()
method to send the email.
Advanced Usage
PHP Mailer offers many advanced features for sending emails, such as attaching files, embedding images, and sending emails in HTML format. Here are a few examples:
Attaching Files:
$mail->addAttachment('/path/to/file.pdf');
Embedding Images:
$mail->addEmbeddedImage('/path/to/image.png', 'logo');
Sending HTML Email:
$mail->isHTML(true);
$mail->Body = '<h1>HTML Email</h1><p>This is an HTML email.</p>';
With these additional features, you can create engaging and interactive emails to enhance your communication with users.
FAQs (Frequently Asked Questions)
- Q: Can I use PHP Mailer with other email providers?
A: Yes, you can use PHP Mailer with any SMTP server that supports SMTP authentication. - Q: How can I handle email errors?
A: PHP Mailer provides error handling mechanisms through exceptions. You can catch exceptions and handle them accordingly. - Q: Can I send bulk emails with PHP Mailer?
A: Yes, PHP Mailer supports sending multiple emails in a loop. However, be cautious with spam regulations and the limitations of your email provider. - Q: Is PHP Mailer secure?
A: PHP Mailer provides security features such as secure socket layer (SSL) and transport layer security (TLS) for secure email communication.
By following this guide, you can harness the power of PHP Mailer to efficiently send emails from your PHP applications. Whether IT‘s transactional emails, newsletters, or notifications, PHP Mailer simplifies the process and provides a reliable solution for email communication.