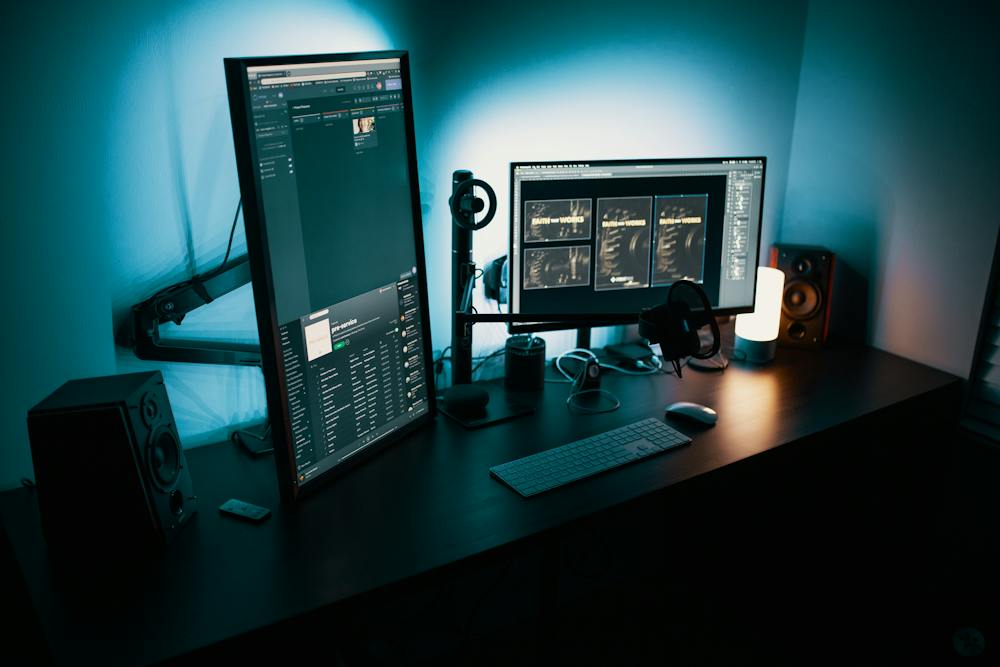
In today’s digital age, email communication is an essential part of every business’s marketing and customer engagement strategy. With the rise of personalized marketing, dynamic emails have become increasingly popular as they provide a more tailored and engaging experience for recipients. In this tutorial, we will explore how to send dynamic emails using Laravel Mail, a powerful email sending library for Laravel, a popular PHP framework.
What are Dynamic Emails?
Dynamic emails, also known as personalized emails, are emails that are customized and tailored to the recipient based on their preferences, behavior, or demographic information. These emails are designed to be more relevant and engaging, ultimately leading to higher open and click-through rates. Dynamic emails can include personalized greetings, product recommendations, personalized offers, and more. By leveraging dynamic emails, businesses can improve their email marketing performance and customer engagement.
Why Use Laravel Mail for Sending Dynamic Emails?
Laravel Mail is a robust and feature-rich library that makes sending emails in Laravel a breeze. IT provides a clean and expressive API for sending various types of emails, including dynamic emails. Laravel Mail offers built-in support for sending HTML emails, attachments, queuing emails for background processing, and more. With its intuitive syntax and powerful features, Laravel Mail is the perfect choice for sending dynamic emails in Laravel applications.
Step-by-Step Tutorial: Sending Dynamic Emails with Laravel Mail
Now, let’s dive into the step-by-step tutorial for sending dynamic emails with Laravel Mail. We will walk through the process of setting up Laravel Mail, creating dynamic email templates, and sending personalized emails to recipients.
Step 1: Install Laravel Mail
If you haven’t already installed Laravel Mail in your Laravel application, you can do so using composer. Simply run the following command in your terminal:
composer require intervention/image
This will install Laravel Mail and all of its dependencies in your Laravel project.
Step 2: Configure Mail Settings
Next, you’ll need to configure your mail settings in the .env
file of your Laravel application. Update the following variables with your mail server’s information:
MAIL_MAILER=smtp
MAIL_HOST=your_smtp_host
MAIL_PORT=your_smtp_port
MAIL_USERNAME=your_smtp_username
MAIL_PASSWORD=your_smtp_password
MAIL_ENCRYPTION=your_smtp_encryption
MAIL_FROM_ADDRESS=your_email_address
MAIL_FROM_NAME="${APP_NAME}"
Make sure to replace your_smtp_host
, your_smtp_port
, your_smtp_username
, your_smtp_password
, and your_email_address
with the actual credentials for your mail server.
Step 3: Create Dynamic Email Templates
Now, let’s create dynamic email templates for our personalized emails. Laravel Mail provides a convenient way to define email templates using Blade, Laravel’s templating engine. Create a new Blade template for your email in the resources/views/emails
directory of your Laravel project. Here’s an example of a dynamic email template:
@component('mail::message')
# Hello, {{ $user->name }}!
You have a new personalized offer just for you.
@component('mail::button', ['url' => $offer->url])
Check it out
@endcomponent
Thanks,
{{ config('app.name') }}
@endcomponent
In this example, we’re using Blade templating to personalize the email content with the recipient’s name and a personalized offer URL. You can customize the email template to include any dynamic content based on the recipient’s information.
Step 4: Send Dynamic Emails
With our email template in place, we can now use Laravel Mail to send personalized emails to recipients. Here’s an example of how to send a dynamic email using Laravel Mail:
use Illuminate\Support\Facades\Mail;
use App\Mail\PersonalizedOffer;
use App\User;
use App\Offer;
$user = User::find(1);
$offer = Offer::find(1);
Mail::to($user->email)->send(new PersonalizedOffer($user, $offer));
In this example, we’re fetching a user and an offer from our database and passing them to the PersonalizedOffer
mail class. This mail class is responsible for sending the dynamic email using the email template we created earlier.
Conclusion
Dynamic emails are a powerful tool for engaging with your audience and driving better results from your email marketing campaigns. By leveraging Laravel Mail, you can easily send personalized and targeted emails to your recipients, leading to higher open and click-through rates. Follow the step-by-step tutorial in this article to get started with sending dynamic emails using Laravel Mail, and take your email marketing to the next level.
FAQs
What is the difference between static and dynamic emails?
Static emails have the same content for all recipients, while dynamic emails are personalized and tailored to each recipient based on their preferences, behavior, or demographic information.
Can I use Laravel Mail to send transactional emails?
Yes, Laravel Mail is suitable for sending transactional emails, such as order confirmations, password reset emails, and more. Its intuitive syntax and powerful features make it a great choice for all types of email communications.
How can I test my dynamic emails before sending them to my recipients?
You can use Laravel’s built-in mail testing features to preview and test your dynamic emails before sending them to your recipients. By using the Mail::fake()
method, you can prevent emails from being sent and instead capture them for inspection and testing.
References
1. “Laravel Mail – Official Documentation” – https://laravel.com/docs/8.x/mail
2. “Sending Email – Laravel Official Documentation” – https://laravel.com/docs/8.x/mail#sending-mail