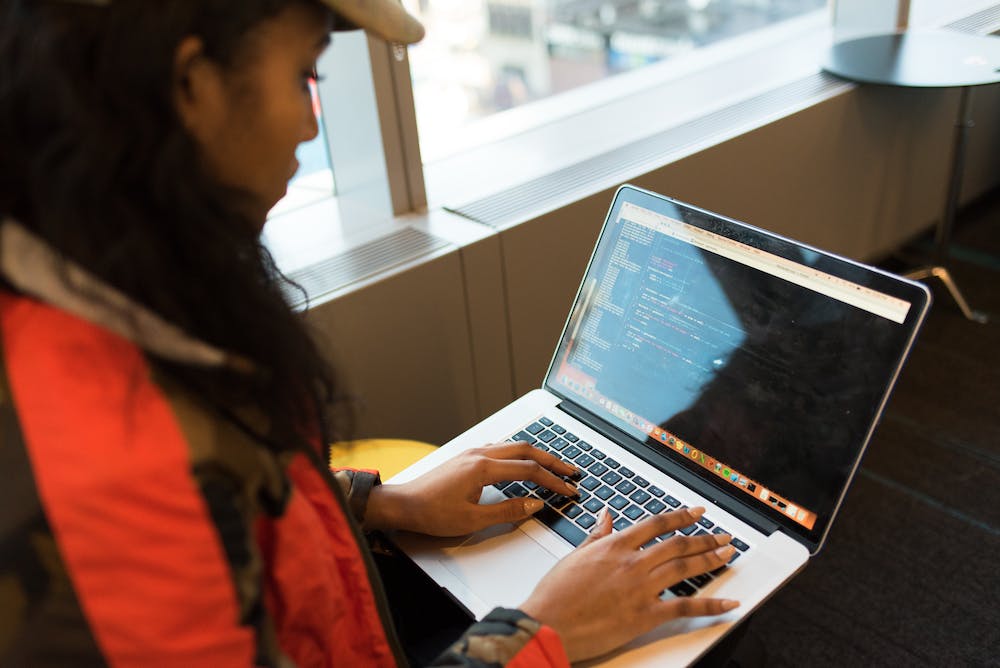
Sorting is a fundamental operation in computer science and is used in various applications such as searching, data analysis, and more. While there are many sorting algorithms available, the insertion sort algorithm stands out for its simplicity and efficiency. In this article, we’ll explore how you can revolutionize your sorting game with an insanely efficient insertion sort Python code.
What is Insertion Sort?
Insertion sort is a simple sorting algorithm that builds the final sorted array (or list) one item at a time. IT is much less efficient on large lists than more advanced algorithms such as quicksort, heapsort, or merge sort. However, insertion sort provides several advantages for small datasets or partially sorted datasets. It is also an excellent algorithm for teaching purposes.
Efficiency of Insertion Sort
The efficiency of insertion sort lies in its simplicity and adaptability to partially sorted datasets. The algorithm works by iterating through an array (or list) and comparing each element with its adjacent elements. If the element is smaller, it is moved to its correct position. This process continues until the entire list is sorted. The time complexity of insertion sort is O(n^2) for the worst-case scenario but can be O(n) for partially sorted arrays.
Python Implementation
Python is a versatile and powerful programming language that makes it easy to implement sorting algorithms. Below is an example of an efficient insertion sort Python code:
“`python
def insertion_sort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i – 1
while j >= 0 and key < arr[j]:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
“`
With just a few lines of code, you can implement an insertion sort algorithm in Python to sort any array or list efficiently.
Revolutionize Your Sorting Game
Now that you have an efficient insertion sort Python code at your disposal, you can revolutionize your sorting game in several ways:
- Improved Performance: By using insertion sort for small or partially sorted datasets, you can significantly improve sorting performance compared to more complex algorithms.
- Learning Experience: Implementing insertion sort in Python can be a valuable learning experience for beginners and intermediate programmers to understand sorting algorithms and their implementation.
- Code Optimization: The simplicity of the insertion sort algorithm makes it easier to optimize and adapt to specific requirements or constraints in your applications.
Conclusion
Insertion sort is a powerful and efficient sorting algorithm that can revolutionize your sorting game with its simplicity and adaptability. By implementing an insertion sort Python code, you can improve performance, gain valuable learning experience, and optimize your code for specific requirements. Take advantage of this insanely efficient sorting algorithm and enhance your programming skills today!
FAQs
Q: Can insertion sort be used for large datasets?
A: While insertion sort is less efficient on large datasets compared to more advanced algorithms, it can still be used for small or partially sorted datasets with good performance.
Q: Is insertion sort suitable for educational purposes?
A: Yes, insertion sort’s simplicity and ease of implementation make it an excellent algorithm for teaching sorting concepts to students and beginner programmers.
Q: Can insertion sort be optimized for specific requirements?
A: Yes, the simplicity of the insertion sort algorithm makes it easier to optimize and adapt to specific constraints or requirements in your applications.