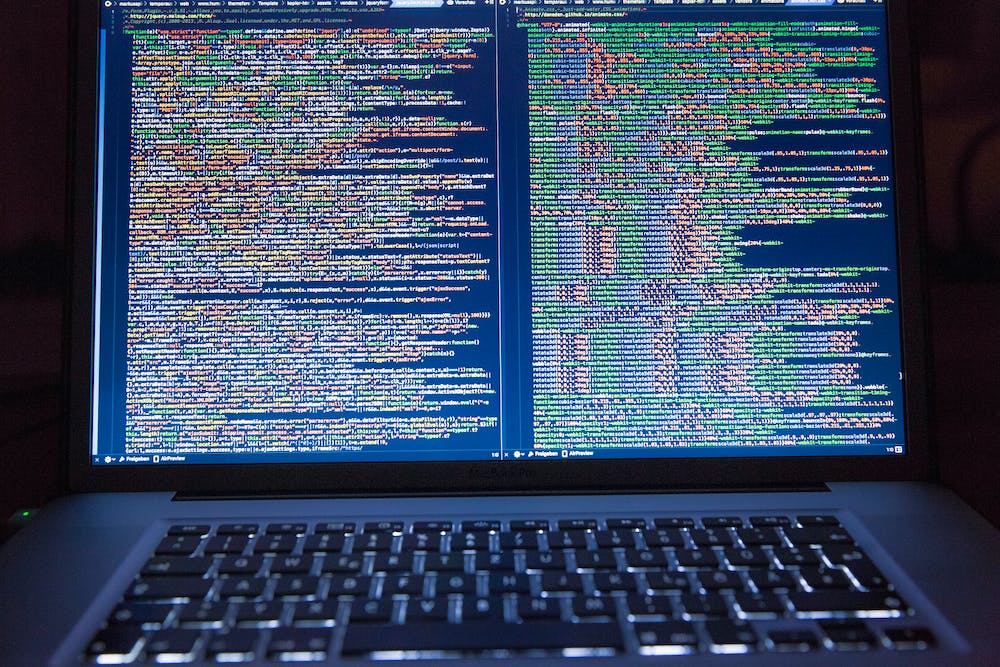
In the world of web development, Laravel has emerged as one of the most popular PHP frameworks due to its elegant syntax, comprehensive features, and seamless database integration. Efficient database insertion is a critical aspect of any web application and Laravel offers an array of techniques that can revolutionize this process.
1. Eloquent ORM
Laravel’s Eloquent ORM (Object-Relational Mapping) provides a simple and intuitive way to interact with the database. IT allows developers to work with database objects as if they were regular PHP objects, making the process of database insertion seamless and efficient.
For example, let’s consider a scenario where we want to insert a new user into the database. With Laravel’s Eloquent ORM, the process becomes as simple as creating a new instance of the User model and setting its properties:
$user = new User;
$user->name = 'John Doe';
$user->email = '[email protected]';
$user->save();
Behind the scenes, Laravel handles the database insertion, ensuring that the data is properly escaped and sanitized. This not only saves development time but also helps prevent common security vulnerabilities like SQL injection.
2. Mass Assignment
Laravel’s mass assignment feature allows developers to insert multiple records into the database with a single line of code, significantly improving efficiency. IT is particularly useful when dealing with bulk data insertion or during data seeding for testing purposes.
Using mass assignment, you can define an array of attributes and pass IT to the `create` method of your model, as shown below:
$users = [
[
'name' => 'John Doe',
'email' => '[email protected]'
],
[
'name' => 'Jane Smith',
'email' => '[email protected]'
]
];
User::create($users);
This simple approach eliminates the need for repetitive database operations, resulting in faster and more efficient insertion of data.
3. Database Transactions
Database transactions are indispensable when IT comes to ensuring data integrity and consistency. Laravel provides a built-in mechanism for managing database transactions, offering an efficient way to handle complex operations.
For example, consider a scenario where you need to insert a user record and update another table simultaneously. If any error occurs during this process, you want to ensure that the changes are rolled back to maintain data consistency.
Laravel’s database transactions simplify this process. By encapsulating the database operations within a transaction, Laravel automatically rolls back any changes if an exception is thrown:
DB::transaction(function () {
$user = new User;
$user->name = 'John Doe';
$user->email = '[email protected]';
$user->save();
$profile = new Profile;
$profile->user_id = $user->id;
$profile->bio = 'Lorem ipsum dolor sit amet.';
$profile->save();
});
If an exception occurs during the transaction, both the user and the associated profile records will not be inserted into the database, maintaining data consistency.
Conclusion
Laravel offers a wide range of revolutionary techniques for seamless database insertion, empowering developers to efficiently interact with the database.
The Eloquent ORM simplifies the process of database insertion, treating database objects as regular PHP objects. Mass assignment allows for bulk data insertion, saving time and effort. Database transactions ensure data integrity and consistency, making IT easier to handle complex operations.
By leveraging these techniques, developers can streamline the development process, improve application performance, and enhance overall efficiency.
FAQs
1. Are these techniques limited to Laravel?
Yes, these techniques are specific to Laravel. Laravel provides a robust database integration layer, making the insertion process seamless and efficient.
2. Can I use these techniques for other databases?
While these techniques are optimized for Laravel’s default database implementation (MySQL), Laravel also supports multiple databases, including PostgreSQL, SQLite, and SQL Server. You can leverage these techniques with other database systems, but some adjustments may be required.
3. Are there any performance considerations when using these techniques?
Laravel has been extensively optimized for performance, and these techniques have been designed to work efficiently. However, IT is always recommended to benchmark and monitor performance to ensure optimal results for your specific application and database environment.