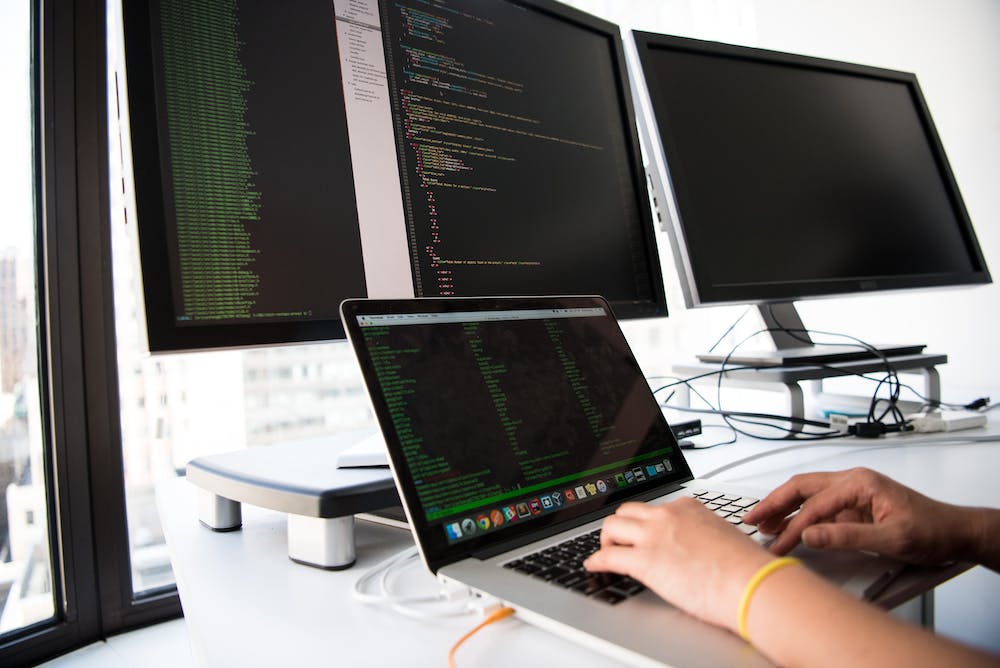
Python is a versatile and powerful programming language that is widely used in web development, data analysis, artificial intelligence, and more. However, like any other programming language, Python has its own set of challenges that can frustrate even the most experienced developers. In this article, we will explore some common coding hurdles that developers face when working with Python and provide solutions to overcome them.
1. Handling Errors and Exceptions
One of the most common challenges that Python developers face is dealing with errors and exceptions. When writing code, IT is inevitable that you will encounter errors, such as syntax errors, logic errors, and runtime errors. In Python, you can use the try-except block to handle exceptions and gracefully manage errors in your code.
For example:
try:
# Your code here
except Exception as e:
# Handle the exception here
By using the try-except block, you can catch and handle exceptions without crashing your program. It is important to use specific exception types (e.g., ValueError, TypeError) when catching exceptions to ensure proper error handling.
2. Managing Dependencies and packages
Another common challenge in Python development is managing dependencies and packages. Python’s package manager, pip, allows you to easily install, upgrade, and remove packages from your project. However, it can be challenging to manage different package versions and dependencies, especially in complex projects.
To overcome this challenge, you can use virtual environments to isolate your project’s dependencies. Virtual environments allow you to create isolated Python environments for each project, which helps to avoid conflicts between different package versions.
For example:
# Create a new virtual environment
python -m venv myenv
# Activate the virtual environment
source myenv/bin/activate
# Install packages within the virtual environment
pip install package_name
By using virtual environments, you can easily manage dependencies and package versions for each of your projects.
3. Optimizing Code Performance
Python is a high-level language known for its simplicity and readability. However, it is not always the fastest language when it comes to performance. In some cases, Python code can be slow, especially when dealing with large datasets or complex algorithms.
To optimize code performance in Python, you can use various techniques such as caching, algorithm optimization, and using built-in data structures and functions. Additionally, you can leverage external libraries such as NumPy and pandas for efficient data processing and manipulation.
4. Writing Clean and Maintainable Code
Writing clean and maintainable code is essential for any software project, and Python is no exception. One of the common challenges developers face is maintaining code quality and readability, especially in collaborative projects.
To overcome this challenge, you can follow best practices and coding standards, such as PEP 8, which provides guidelines for writing clean and readable Python code. Additionally, you can use code linters and static analysis tools to identify and fix code quality issues.
Conclusion
In conclusion, Python is a powerful programming language that comes with its own set of challenges. However, with the right approach and mindset, you can overcome common coding hurdles and become a more proficient Python developer. By effectively handling errors and exceptions, managing dependencies, optimizing code performance, and writing clean and maintainable code, you can take your Python programming skills to the next level.
FAQs
Q: What are some common errors in Python programming?
A: Some common errors in Python programming include syntax errors, logic errors, and runtime errors.
Q: How can I optimize code performance in Python?
A: You can optimize code performance in Python by using techniques such as caching, algorithm optimization, and leveraging external libraries for efficient data processing.