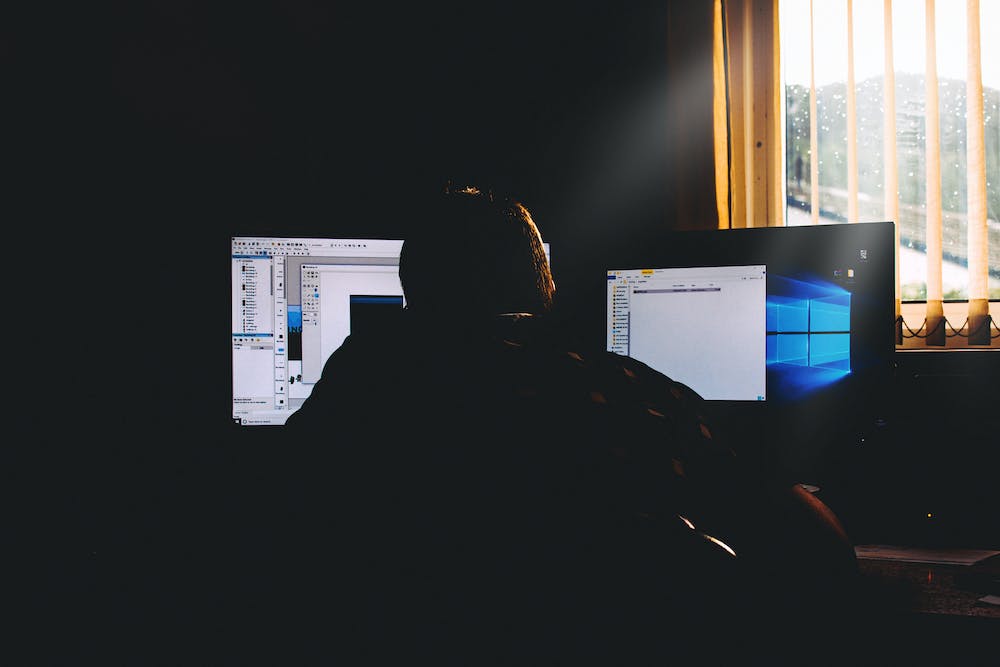
In the world of programming, writing clean and readable code is of utmost importance. The way code is written can greatly impact not only the developer who works on IT but also the overall functionality and efficiency of the application. Python, being a versatile and popular programming language, requires a specific coding style guide to ensure that the code remains clean, readable, and maintainable. In this article, we will explore the essential aspects of Python coding style and how to write clean and readable code that adheres to these guidelines.
Importance of Clean and Readable Code
Clean and readable code is essential for several reasons. Firstly, it makes the code easy to understand and maintain. When multiple developers work on the same project, having clean and readable code ensures that everyone can understand the codebase and make changes without introducing errors or bugs. Additionally, clean code reduces the likelihood of introducing technical debt, which can be costly to address in the long run. Furthermore, readable code promotes collaboration and makes it easier for new developers to onboard and contribute to the project.
Python Coding Style Guidelines
Python has its own specific coding style guidelines, often referred to as PEP 8 (Python Enhancement Proposal 8). PEP 8 provides a set of recommendations for writing clean and readable code in Python. Some of the key guidelines include:
- Use 4-space indentation
- Avoid mixing tabs and spaces for indentation
- Limit line length to 79 characters
- Use descriptive variable and function names
- Use whitespace to improve readability
- Follow naming conventions for variables, functions, and classes
- Use docstrings to document modules, functions, classes, and methods
Writing Clean and Readable Code in Python
Now that we understand the importance of clean and readable code and the coding style guidelines for Python, let’s delve into some best practices for writing clean and readable code in Python.
Consistent Indentation
Python uses indentation to define the scope of code blocks, such as loops, conditional statements, and function definitions. It is essential to use consistent indentation throughout the codebase to improve readability and maintainability. PEP 8 recommends using 4-space indentation, which is the standard in the Python community.
Descriptive Variable and Function Names
Choosing descriptive and meaningful names for variables and functions can significantly improve the readability of the code. Instead of using vague or single-letter variable names, opt for names that reflect the purpose or role of the variable in the code. The same applies to function names – they should be descriptive and indicative of their functionality.
Limit Line Length
PEP 8 recommends limiting the line length to 79 characters to ensure that the code remains readable without the need for excessive horizontal scrolling. This guideline promotes concise and clear code, as well as better visibility when comparing different parts of the code.
Whitespace for Readability
Using whitespace effectively can significantly improve the readability of the code. It is important to use whitespace to separate logical sections of the code, such as functions, classes, and loops. Additionally, adding whitespace around operators and after commas can enhance the overall clarity of the code.
Follow Naming Conventions
Python has specific naming conventions for variables, functions, and classes. For example, variable names should be in lowercase with words separated by underscores (e.g., my_variable), while class names should follow the CapWords convention (e.g., MyClass). Adhering to these naming conventions can make the code more consistent and readable across the entire codebase.
Documenting Code with Docstrings
Docstrings are used to document modules, functions, classes, and methods in Python. They provide a clear and concise way to explain the purpose and usage of the code. Writing informative docstrings can greatly enhance the readability of the code and make it easier for other developers to understand and utilize the functionality.
Conclusion
Writing clean and readable code in Python is essential for maintaining a high-quality codebase, promoting collaboration, and ensuring the long-term maintainability of the project. By following the coding style guidelines outlined in PEP 8 and adopting best practices for clean code, developers can create code that is easy to understand, maintain, and extend. Ultimately, clean and readable code contributes to the overall success and efficiency of a Python project.
FAQs
What are the benefits of writing clean and readable code?
Writing clean and readable code promotes collaboration, reduces technical debt, and ensures the maintainability of the codebase. It also makes it easier for new developers to understand and contribute to the project.
Why is consistent indentation important in Python?
Consistent indentation improves the readability and maintainability of the code, as it clearly defines the scope of code blocks and logical sections.
How can I improve the documentation of my Python code?
Using informative docstrings and following the PEP 257 guidelines can help improve the documentation of your Python code, making it easier for other developers to understand and utilize the functionality.