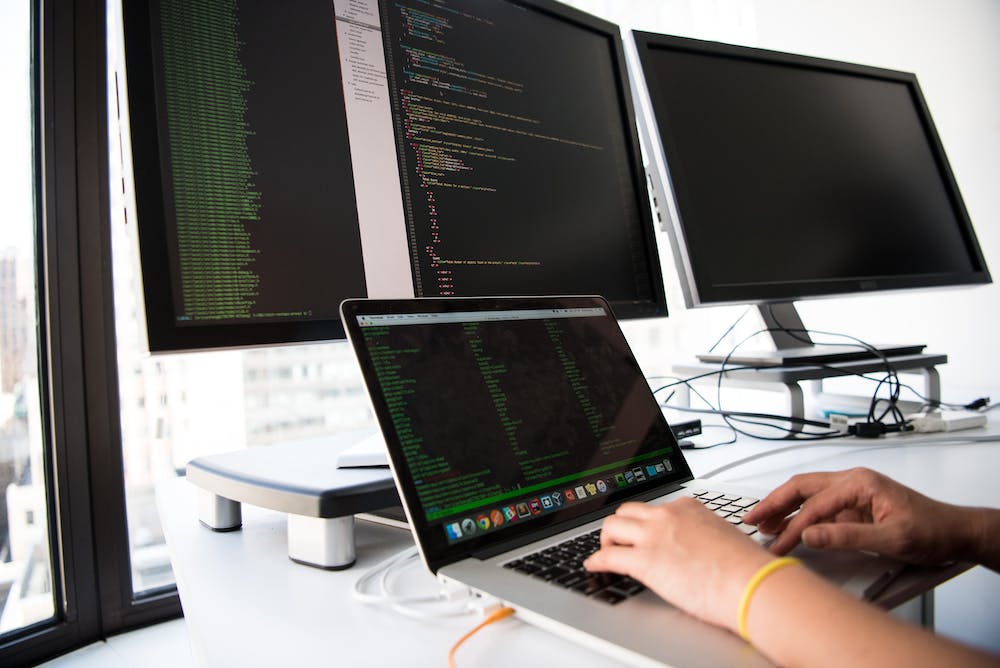
In today’s fast-paced world, performance is a critical aspect of software development. When IT comes to Python programming, optimizing code for faster execution can make a significant difference in the overall performance of the application. In this article, we will explore various techniques for optimizing Python code to improve its speed and efficiency.
1. Use Built-in Functions and Libraries
One of the easiest ways to improve the performance of your Python code is to leverage built-in functions and libraries. Python provides a rich set of libraries and modules that offer efficient implementations of common tasks. For example, instead of writing custom code for sorting a list, you can use the built-in sorted()
function, which is optimized for performance.
2. Avoid Using Global Variables
Global variables can slow down the execution of your code, especially in larger applications. Every time a global variable is accessed or modified, Python has to perform a lookup to find the variable in the global scope, which can be time-consuming. By minimizing the use of global variables and instead passing values as function parameters or using local variables, you can improve the performance of your code.
3. Use List Comprehensions
List comprehensions are a concise and efficient way to generate lists in Python. They provide a more readable and faster alternative to traditional for loops for creating lists. By using list comprehensions, you can reduce the number of lines of code and improve the performance of your application.
4. Optimize Loops
Loops are an essential part of Python programming, but they can also be a performance bottleneck if not used efficiently. One way to optimize loops is to minimize the number of iterations by using techniques such as early exit conditions or iterating over smaller data sets. Additionally, using built-in functions like map()
and filter()
can often be more efficient than writing custom loops.
5. Use Generators
Generators are a powerful feature in Python that allow you to create iterators without the need to store the entire sequence in memory. By using generators, you can improve the memory efficiency of your code and reduce the overhead of creating and managing large data sets.
6. Profile Your Code
Profiling is a technique used to analyze the performance of a program and identify areas where optimization is needed. Python provides built-in modules like profile
and timeit
to help you measure the performance of your code. By profiling your code, you can pinpoint the parts that need optimization and focus your efforts on improving those areas.
7. Use External Libraries and Modules
There are several external libraries and modules available for Python that offer optimized implementations of common algorithms and data structures. For example, the NumPy
library provides efficient multi-dimensional arrays and mathematical functions, while the pandas
library offers high-performance data manipulation and analysis tools. By leveraging these external libraries, you can take advantage of their optimized code for improved performance.
8. Avoid Unnecessary Recalculation
In some cases, a piece of code may perform the same calculation multiple times, even though the result remains constant. By caching or memoizing the results of expensive computations, you can avoid unnecessary recalculation and improve the overall performance of your code.
Conclusion
Optimizing Python code for faster execution is essential for building high-performance applications. By following the techniques outlined in this article, you can improve the speed and efficiency of your Python code and deliver a better user experience. From using built-in functions and libraries to profiling your code for performance analysis, there are numerous optimization techniques available to Python developers.
FAQs
Q: How important is code optimization in Python?
A: Code optimization is crucial in Python as it can significantly impact the performance and efficiency of your applications. Optimized code can lead to faster execution, reduced memory consumption, and improved user experience.
Q: Are there any drawbacks to code optimization in Python?
A: While code optimization can improve the performance of your Python applications, it can also make the code more complex and harder to maintain. It’s important to find a balance between optimization and readability to ensure that your code remains maintainable in the long run.
Q: Should I always prioritize speed over readability in Python code?
A: While speed is important, readability should not be sacrificed for the sake of optimization. It’s essential to write clean and understandable code that can be easily maintained and extended. Prioritize optimization only when necessary and where performance improvements are significant.
Q: Can external libraries and modules help in code optimization?
A: Yes, external libraries and modules often provide optimized implementations of common tasks, such as mathematical operations, data manipulation, and algorithmic functions. By leveraging these external resources, you can improve the performance of your Python code without having to reinvent the wheel.
Q: How can I measure the performance of my Python code?
A: Python provides built-in modules like profile
and timeit
to help you measure the performance of your code. Additionally, there are third-party tools and profilers available that can provide more detailed insights into the execution of your code.