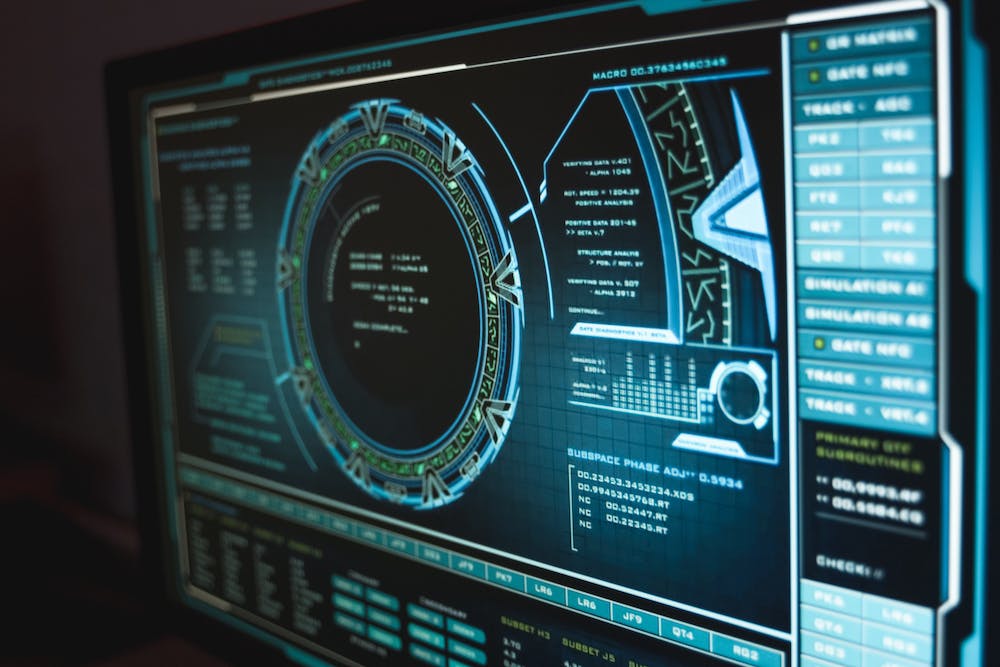
Python is a widely-used, high-level programming language known for its simplicity and readability. IT is an excellent language for beginners to learn and for experienced developers to quickly prototype applications. In this article, we will cover some basic concepts and provide code examples to help you master Python.
Variables and Data Types
One of the fundamental concepts in Python is variables. A variable is a name that refers to a value. In Python, variables are dynamically typed, which means you can assign any type of data to a variable without declaring the type. Here’s an example:
x = 5
y = "Hello, World!"
z = 3.14
print(x, y, z)
In this example, we have assigned an integer, a string, and a float to variables x
, y
, and z
respectively.
Control Flow
Python supports various control flow statements such as if
, for
, and while
to control the flow of a program. Here’s an example of an if
statement:
age = 20
if age < 18:
print("You are a minor")
else:
print("You are an adult")
In this example, we use the if-else
statement to check if the variable age
is less than 18.
Functions
Functions are a block of reusable code that performs a specific task. In Python, you can define a function using the def
keyword. Here’s an example:
def greet(name):
print("Hello, " + name + "!")
greet("Alice")
This example defines a function called greet
that takes a parameter name
and prints a greeting using that name.
Lists and Dictionaries
Python provides built-in data structures such as lists and dictionaries to store collections of data. Here’s an example of a list:
colors = ["red", "green", "blue"]
print(colors[0])
In this example, we create a list of colors and print the first element of the list using its index.
And here’s an example of a dictionary:
person = {"name": "Alice", "age": 25}
print(person["name"])
In this example, we create a dictionary to store information about a person and access the value associated with the key name
.
Conclusion
Python is a versatile and powerful programming language with a vast ecosystem of libraries and tools. Mastering the basic concepts of Python is essential for building a strong foundation in programming. In this article, we covered variables, control flow, functions, and data structures with code examples to help you understand these concepts better.
FAQs
Q: Can I use Python for web development?
A: Yes, Python can be used for web development with frameworks like Django and Flask.
Q: Is Python a good language for beginners?
A: Yes, Python’s simple syntax and readability make IT an excellent language for beginners to learn.
Q: Where can I learn more about Python?
A: You can find numerous online resources, tutorials, and books to learn more about Python programming.
Q: Is Python open-source?
A: Yes, Python is an open-source programming language with a large and active community.