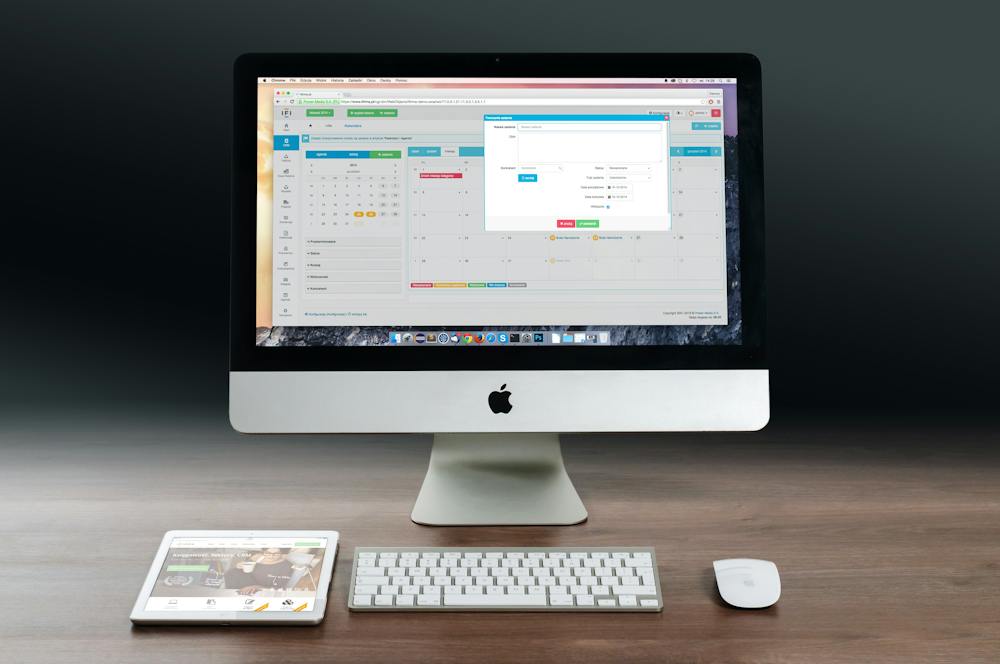
Python is one of the most popular and versatile programming languages in the world. IT is known for its simplicity and ease of learning, making it an ideal language for beginners. Whether you are new to programming or have some experience with other languages, mastering Python can open up a world of opportunities for you. In this article, we will take you through the basics of Python and provide you with the knowledge and resources you need to master the language from scratch.
Getting Started with Python
If you are completely new to programming, Python is a great language to start with. Its syntax is clear and easy to read, making it a great language for beginners to learn. To get started, you will need to download and install Python on your computer. You can do this by visiting the official Python Website and following the installation instructions for your operating system.
Basic Syntax and Data Types
Once you have Python installed, it’s time to start learning the basics. Python uses a simple and clean syntax, which makes it easy to read and write code. The first thing you will need to understand is the basic data types in Python, such as integers, floats, strings, and booleans. You will also need to learn about variables and how to use them to store and manipulate data in your programs.
Here’s an example of a simple Python program that prints “Hello, World!”:
print("Hello, World!")
Control Flow and Loops
Control flow and loops are essential concepts in programming. Python provides several ways to control the flow of your program, such as if statements and loops. Understanding how to use these constructs will allow you to write more complex and powerful programs. Here’s an example of a simple if statement in Python:
x = 10
if x > 5:
print("x is greater than 5")
Functions and Modules
Functions allow you to break down your code into smaller, reusable chunks. Python comes with a wide range of built-in functions, but you can also create your own custom functions. Modules are Python files that contain collections of related functions and variables. Understanding how to work with functions and modules will help you write more organized and maintainable code.
Intermediate Python
Once you have a good grasp of the basics, it’s time to move on to more intermediate topics. This includes working with data structures, handling exceptions, and file input/output. Python has a rich standard library that provides many powerful tools for working with data, files, and more.
Data Structures
Python provides several built-in data structures, such as lists, tuples, dictionaries, and sets. Understanding how to work with these data structures will allow you to store and manipulate data in your programs more effectively. Here’s an example of creating and manipulating a list in Python:
my_list = [1, 2, 3, 4, 5]
print(my_list[2])
Exception Handling
Errors and exceptions are a normal part of programming. Python provides a simple and powerful mechanism for handling errors through the use of try and except blocks. Learning how to handle exceptions will allow you to write more robust and reliable code.
File Input/Output
Working with files is a common task in programming. Python provides a simple and elegant way to read from and write to files. Understanding file input/output will allow you to work with external data and store the results of your programs.
Advanced Python
Once you have mastered the basics and intermediate topics, you can move on to more advanced aspects of Python. This includes topics such as object-oriented programming, regular expressions, and working with databases.
Object-Oriented Programming
Python is an object-oriented language, which means that it provides support for classes and objects. Understanding object-oriented programming will allow you to write more organized and modular code. Here’s an example of creating a simple class in Python:
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
print(f"{self.name} says woof!")
Regular Expressions
Regular expressions are a powerful tool for matching and manipulating text. Python provides support for regular expressions through the built-in re module. Understanding how to work with regular expressions will allow you to perform complex text processing tasks in your programs.
Working with Databases
Python provides support for working with a wide range of databases, such as SQLite, MySQL, and PostgreSQL. Understanding how to work with databases will allow you to store and retrieve data from external sources in your programs.
Conclusion
Python is a powerful and versatile programming language that is ideal for beginners. By mastering Python, you can unlock a world of opportunities in the world of programming and software development. Whether you are interested in web development, data science, or machine learning, Python has something to offer. By following the steps outlined in this article and practicing regularly, you can become proficient in Python and take your programming skills to the next level.
FAQs
What is Python?
Python is a high-level, interpreted programming language known for its simplicity and ease of learning.
Is Python a good language for beginners?
Yes, Python is a great language for beginners due to its clear and simple syntax.
How can I practice Python?
You can practice Python by working on coding challenges, building small projects, and contributing to open-source projects.
Are there any resources for learning Python?
There are many resources available for learning Python, including online tutorials, books, and interactive coding platforms.
How long does it take to master Python?
The time it takes to master Python varies from person to person, but regular practice and dedication are key to success.