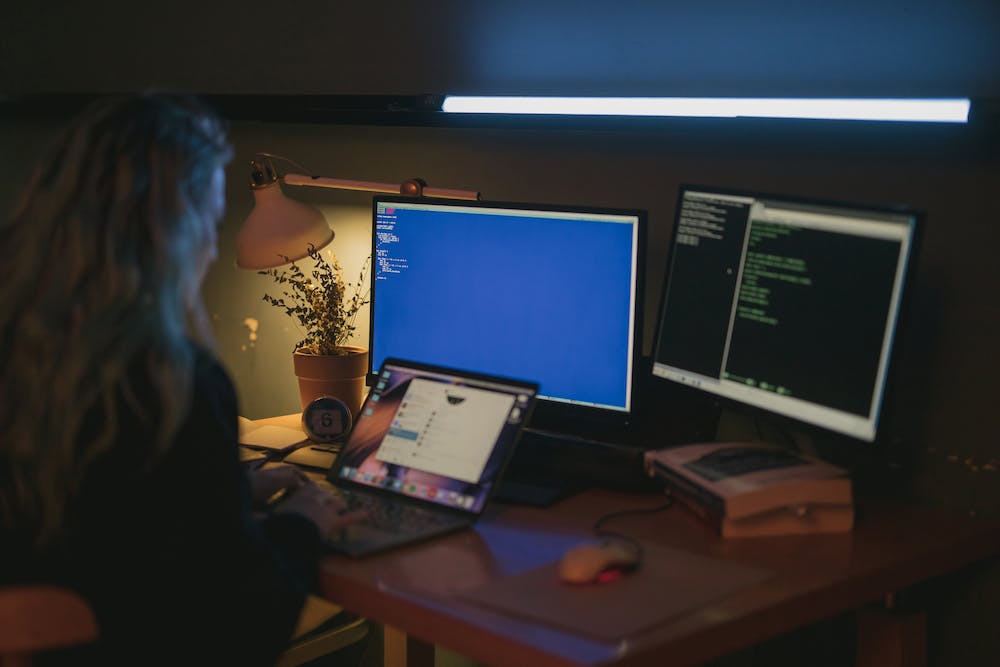
Introduction
Arrays are essential data structures in PHP that allow developers to store and manipulate sets of values efficiently. As PHP developers, IT‘s important to optimize our code to achieve better performance. In this article, we will explore some mind-blowing tricks and techniques to optimize your new arrays in PHP.
1. Use Short Array Syntax
PHP 5.4 introduced the short array syntax, which allows you to define arrays using square brackets: $array = [1, 2, 3];
. This syntax is not only more concise but also improves readability and maintenance of your code.
2. Preallocate Array Sizes
If you know the size of your array in advance, IT‘s recommended to preallocate the necessary memory by setting the size explicitly. This can be done using the array_fill
function or by initializing the array with a specific size and default values.
$array = array_fill(0, 1000, 'default_value');
3. Use Associative Arrays Instead of Search Functions
When you need to map values to keys and perform lookup operations, associative arrays can be a great alternative to search functions like in_array
. Associative arrays provide faster lookup times as the value can be directly accessed using the associated key.
$array = ['key_1' => 'value_1', 'key_2' => 'value_2'];
4. Take Advantage of Array Functions
PHP offers a wide range of array functions that can significantly simplify your code and improve performance. Functions like array_map
, array_filter
, and array_reduce
can eliminate the need for loops and help optimize your array operations.
5. Use SPL Data Structures
The Standard PHP Library (SPL) provides various data structures that can offer better performance and functionality compared to regular arrays. Classes like SplFixedArray
and SplDoublyLinkedList
can be utilized based on your specific requirements.
Conclusion
Optimizing arrays in PHP is crucial for achieving better performance in your applications. By using the aforementioned tricks and techniques, you can drastically improve both the efficiency and readability of your code. Embrace these PHP wizardry tricks and witness the mind-blowing transformations in your array handling!
FAQs
Q1: Are these tricks applicable to all PHP versions?
Most of these tricks are applicable to PHP versions 5.4 and above. However, IT‘s always recommended to check the compatibility with your specific PHP version.
Q2: How much performance improvement can I expect from these optimizations?
The actual performance improvement depends on various factors like array size, operations performed, and server configuration. However, implementing these optimizations correctly can result in significant performance gains.
Q3: Can these tricks be used in conjunction with database operations?
Absolutely! These tricks primarily focus on optimizing array operations within PHP. Database operations can benefit from these optimizations as well, especially when retrieving and manipulating data in arrays.
Q4: Are there any caveats or potential drawbacks with these techniques?
While these tricks generally result in performance improvements, IT‘s essential to consider the trade-offs in terms of code readability and maintainability. Ensure that you and your team fully understand the implemented techniques to avoid any potential drawbacks.