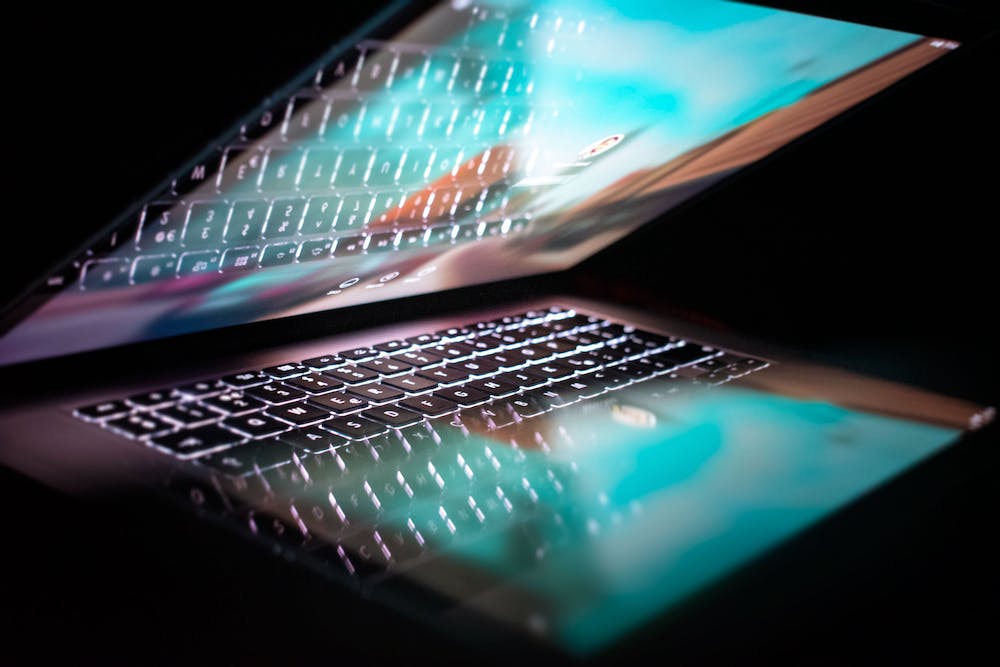
PHP is a widely-used and powerful server-side scripting language that is especially suited for web development. IT is used to build dynamic and interactive websites. In PHP, variables have different scopes, which determines where they can be accessed and used within a PHP script. In this article, we will explore the various variable scopes in PHP, including local, global, and static scopes.
Local Scope
In PHP, a variable declared within a function has a local scope. This means that it can only be accessed and used within that particular function. Once the function finishes executing, the variable is destroyed and its value is no longer available.
Here’s an example of a local variable in PHP:
<?php
function myFunction() {
$x = 10; // $x is a local variable
echo $x;
}
myFunction();
// echo $x; // This will throw an error as $x is not accessible outside the function
?>
In this example, the variable $x is declared inside the myFunction() function, and it can only be accessed within that function. If we try to access $x outside the function, it will result in an error.
Global Scope
Unlike local variables, global variables in PHP can be accessed and used throughout the entire script, including within functions. To declare a global variable, we use the global
keyword within a function.
Here’s an example of a global variable in PHP:
<?php
$y = 20; // $y is a global variable
function myFunction() {
global $y; // Accessing global variable $y inside the function
echo $y;
}
myFunction();
echo $y; // This will output 20
?>
In this example, the variable $y is declared outside the function, making it a global variable. We use the global
keyword to access this global variable inside the myFunction() function, and then we can use it to perform operations within the function as well as outside of it.
Static Scope
In addition to local and global scopes, PHP also has a static scope for variables. A static variable in PHP retains its value between function calls. This means that the variable is not destroyed when the function finishes executing, and its value is maintained and updated across multiple function calls.
Here’s an example of a static variable in PHP:
<?php
function myFunction() {
static $z = 30; // $z is a static variable
echo $z;
$z++; // Incrementing the static variable $z
}
myFunction(); // This will output 30
myFunction(); // This will output 31
myFunction(); // This will output 32
?>
In this example, the variable $z is declared as a static variable inside the myFunction() function. When the function is called multiple times, the value of $z is maintained between calls and is updated each time the function is executed.
Conclusion
In conclusion, PHP has three main variable scopes: local, global, and static. Understanding these variable scopes is crucial for writing efficient and maintainable PHP code. Local variables are limited to the function in which they are declared, global variables are accessible throughout the entire script, and static variables retain their value between function calls. By using the appropriate variable scope, developers can ensure that their code is well-organized and functions as intended.
FAQs
Q: Can I mix variable scopes in PHP?
A: Yes, you can mix variable scopes in PHP. For example, you can have a global variable and a local variable with the same name, without any conflicts.
Q: What is the best practice for using variable scopes in PHP?
A: It’s generally a good practice to use local variables within functions whenever possible, and limit the use of global variables to only when they are absolutely necessary. Using static variables sparingly can help maintain state across function calls without cluttering the global scope.
Q: Are there any performance implications of using different variable scopes in PHP?
A: Local variables are typically more efficient than global variables, as they have a smaller scope and are destroyed once a function finishes executing. Using global variables excessively can lead to unnecessary memory consumption and potential conflicts in larger codebases. Static variables have a minimal performance impact and can be useful for maintaining state across function calls.
Q: How can I ensure that my code is optimized for variable scopes?
A: Writing modular and well-organized code with clear variable scopes can help optimize the performance and maintainability of your PHP code. Avoiding excessive use of global variables and relying on local variables within functions can make your code more predictable and easier to debug.