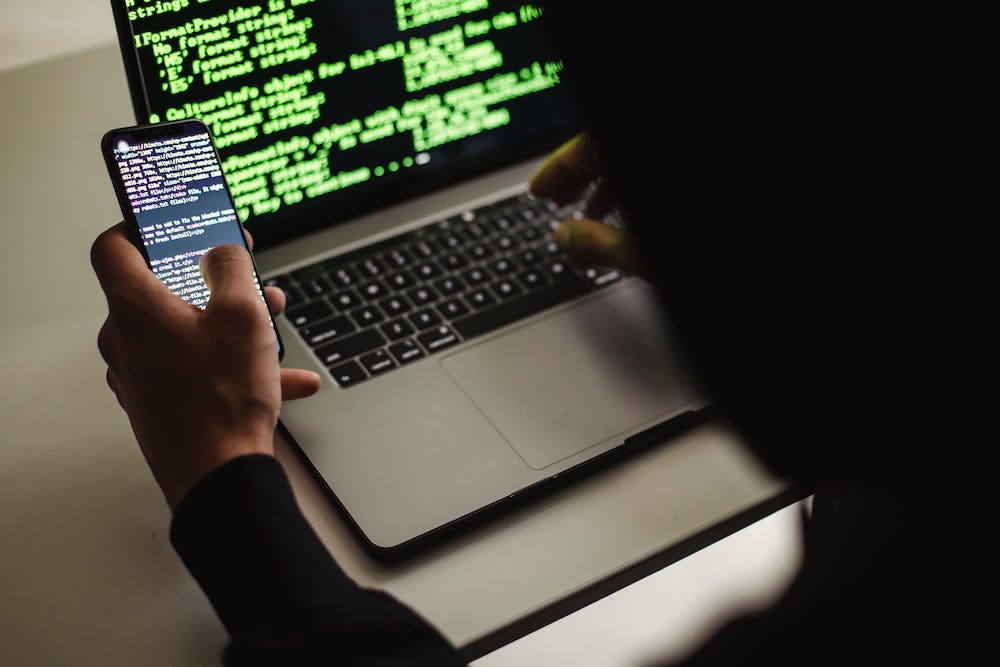
If you’re a web developer, chances are you’ve heard of PHP. IT‘s one of the most popular server-side scripting languages used for creating dynamic web pages. But what you may not know is that PHP has a powerful tool called IMAP that can revolutionize the way you handle email in your web applications.
IMAP, which stands for internet Message Access Protocol, is a standard protocol used by email clients to retrieve messages from a mail server. With PHP’s IMAP extension, you can easily integrate email functionality into your web applications, allowing you to read, search, and manipulate emails directly from your PHP code.
Getting Started with PHP IMAP
Before we dive into the details of PHP IMAP, let’s first understand how to get started with it. The IMAP extension is bundled with PHP, so there’s no need to install any additional software. You can simply enable the extension in your PHP configuration file and start using it right away.
To enable the IMAP extension, open your php.ini file and uncomment the following line:
extension=imap
Once you’ve done that, restart your web server, and the IMAP extension will be ready to use. You can now start writing code to interact with email servers using PHP.
Reading Emails with PHP IMAP
One of the most common use cases for PHP IMAP is reading emails from a mail server. With just a few lines of code, you can connect to an email server, retrieve messages, and process them in your PHP application.
Let’s take a look at a simple example of how to use PHP IMAP to read emails:
<?php
// Server settings
$server = '{mail.example.com:993/imap/ssl}INBOX';
$username = '[email protected]';
$password = 'password';
// Connect to the IMAP server
$inbox = imap_open($server, $username, $password);
// Get the list of emails in the inbox
$emails = imap_search($inbox, 'ALL');
// Loop through all the emails and display the subject
foreach ($emails as $email_number) {
echo "Subject: " . imap_utf8(imap_headerinfo($inbox, $email_number)->subject) . "<br>";
}
// Close the connection
imap_close($inbox);
?>
In this example, we first specify the server settings, including the server address, username, and password. We then use the imap_open function to connect to the IMAP server and retrieve the list of emails in the inbox using imap_search. Finally, we loop through the emails and display the subject of each email.
Searching and Filtering Emails
PHP IMAP also allows you to search and filter emails based on various criteria, such as sender, subject, date, or message content. This is particularly useful when you need to process a large number of emails and only want to retrieve those that meet specific criteria.
Here’s an example of how to search for emails with a specific subject using PHP IMAP:
<?php
// Connect to the IMAP server
$inbox = imap_open($server, $username, $password);
// Search for emails with a specific subject
$emails = imap_search($inbox, 'SUBJECT "Your Subject Here"');
// Loop through the matching emails and process them
foreach ($emails as $email_number) {
// Process the email
}
// Close the connection
imap_close($inbox);
?>
In this example, we use the imap_search function to search for emails with the subject “Your Subject Here”. The function returns an array of email numbers that match the search criteria, which we can then process in our PHP code.
Manipulating Emails
PHP IMAP also provides a set of functions for manipulating emails, such as marking emails as read or unread, moving emails to different folders, deleting emails, and more. This allows you to automate the management of emails in your web applications and streamline your workflow.
Let’s see an example of how to mark an email as read using PHP IMAP:
<?php
// Connect to the IMAP server
$inbox = imap_open($server, $username, $password);
// Get the list of unread emails in the inbox
$unread_emails = imap_search($inbox, 'UNSEEN');
// Mark each unread email as read
foreach ($unread_emails as $email_number) {
imap_setflag_full($inbox, $email_number, "\\Seen");
}
// Close the connection
imap_close($inbox);
?>
In this example, we use imap_search to retrieve the list of unread emails in the inbox and then use imap_setflag_full to mark each email as read by adding the “\\Seen” flag.
Conclusion
PHP IMAP is a powerful tool that every web developer should be familiar with. It allows you to seamlessly integrate email functionality into your PHP applications, from reading and searching emails to manipulating and managing them. By leveraging the capabilities of PHP IMAP, you can enhance the functionality of your web applications and provide a more seamless user experience for handling email communications.
So the next time you’re building a web application that involves email, consider using PHP IMAP to streamline your email handling process and take your web development skills to the next level.
FAQs
What is PHP IMAP?
PHP IMAP is a PHP extension that allows developers to interact with mail servers using the IMAP protocol. It provides functions for reading, searching, and manipulating emails directly from PHP code.
Is PHP IMAP compatible with all mail servers?
PHP IMAP is compatible with most standard mail servers that support the IMAP protocol. However, there may be specific configurations or limitations with certain mail servers, so it’s important to test and verify compatibility with your target mail server.
Can PHP IMAP be used for sending emails?
No, PHP IMAP is designed specifically for retrieving and manipulating emails from mail servers. For sending emails, you can use the built-in mail function in PHP or a dedicated email library such as PHPMailer.
Are there any security considerations when using PHP IMAP?
When using PHP IMAP, it’s important to handle user credentials and sensitive information securely to prevent unauthorized access to email accounts. Always use secure connections (e.g., SSL) when connecting to mail servers and validate user input to prevent potential security vulnerabilities.
Overall, PHP IMAP is a versatile and indispensable tool for any web developer looking to enhance their email handling capabilities in web applications. By familiarizing yourself with PHP IMAP and leveraging its powerful features, you can take your web development skills to new heights and provide a seamless email experience for your users.