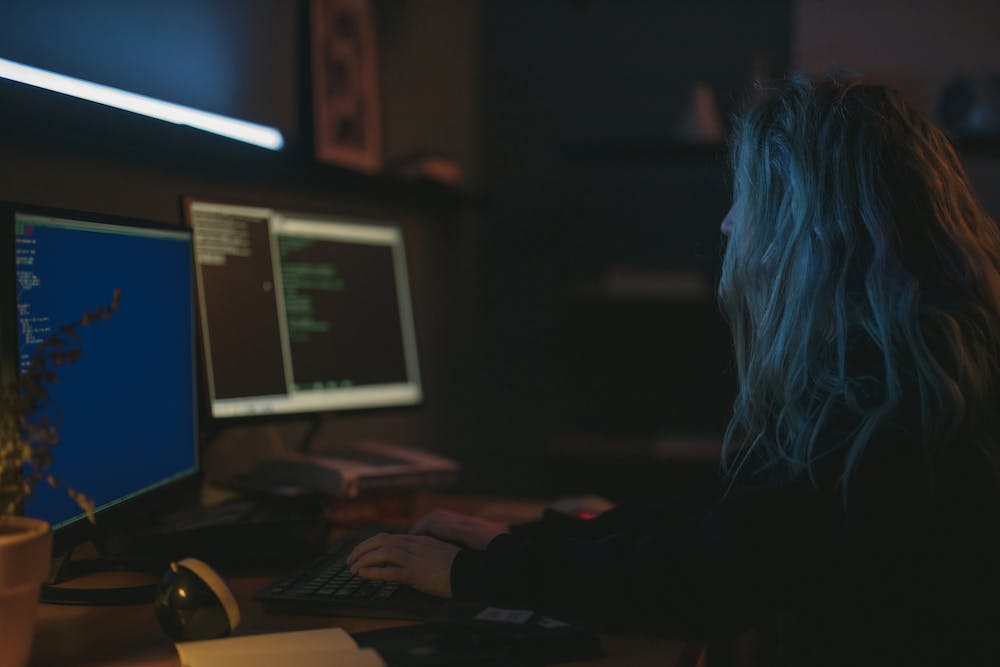
PHP cURL is a powerful library that allows developers to send and receive HTTP requests programmatically. IT provides a wide range of features and options, making IT a popular choice for interacting with APIs and retrieving data from remote servers. In this article, we will walk through a step-by-step guide on how to use PHP cURL effectively, along with some frequently asked questions to help you get started.
Step 1: Installing and Enabling cURL Extension
Before we can start using PHP cURL, we need to verify if cURL extension is installed and enabled in PHP. Usually, IT comes bundled with PHP, but IT might be disabled in some cases. To check if cURL is enabled, create a PHP file and add the following code snippet:
<?php
if(function_exists('curl_version')) {
echo 'cURL is enabled on this server.';
} else {
echo 'cURL is not enabled on this server.';
}
?>
If cURL is enabled, you will see the message “cURL is enabled on this server.” Otherwise, you will see the message “cURL is not enabled on this server.” If cURL is not enabled, you can follow the PHP documentation or reach out to your hosting provider to enable IT.
Step 2: Making GET Requests
One of the most common use cases for PHP cURL is making HTTP GET requests. To make a GET request, you need to initialize a cURL session using the curl_init() function, set the URL of the request using curl_setopt(), execute the request using curl_exec(), and finally, close the cURL session using curl_close(). Here’s an example:
<?php
// Initialize cURL session
$curl = curl_init();
// Set URL
curl_setopt($curl, CURLOPT_URL, 'https://api.example.com/data');
// Execute the GET request
$response = curl_exec($curl);
// Close the cURL session
curl_close($curl);
// Handle the response
echo $response;
?>
In this example, we initialize a cURL session, set the URL to ‘https://api.example.com/data’, execute the GET request, and store the response in the $response variable. Finally, we close the cURL session and echo the response.
Step 3: Making POST Requests
Besides GET requests, PHP cURL also allows us to make POST requests to send data to a remote server. To make a POST request, you need to set the CURLOPT_POST option to true, and specify the POST data using the CURLOPT_POSTFIELDS option. Here’s an example:
<?php
// Initialize cURL session
$curl = curl_init();
// Set URL
curl_setopt($curl, CURLOPT_URL, 'https://api.example.com/data');
// Set the request method to POST
curl_setopt($curl, CURLOPT_POST, true);
// Set the POST data
curl_setopt($curl, CURLOPT_POSTFIELDS, [
'key1' => 'value1',
'key2' => 'value2'
]);
// Execute the POST request
$response = curl_exec($curl);
// Close the cURL session
curl_close($curl);
// Handle the response
echo $response;
?>
In this example, we set the CURLOPT_POST option to true, and we pass an array of key-value pairs as the POST data using the CURLOPT_POSTFIELDS option. When the POST request is executed, the server will receive the specified data.
Frequently Asked Questions
-
Q: What is cURL?
A: cURL is a library and command-line tool used for making HTTP requests. In PHP, cURL is commonly used to interact with APIs and retrieve data from remote servers.
-
Q: Can I send custom headers with cURL?
A: Yes, you can send custom headers using the CURLOPT_HTTPHEADER option in PHP cURL. IT allows you to set headers such as Authorization, content-Type, User-Agent, and more.
-
Q: How can I handle errors when using cURL?
A: cURL provides error handling capabilities through the curl_errno() and curl_error() functions. curl_errno() returns the error number, while curl_error() returns the error message associated with the last cURL request.
-
Q: Is IT possible to upload files with cURL?
A: Yes, you can upload files with cURL by setting the CURLOPT_UPLOAD option to true and specifying the file path using the CURLOPT_POSTFIELDS option. Additionally, you can use the @ symbol before the file path to upload files.