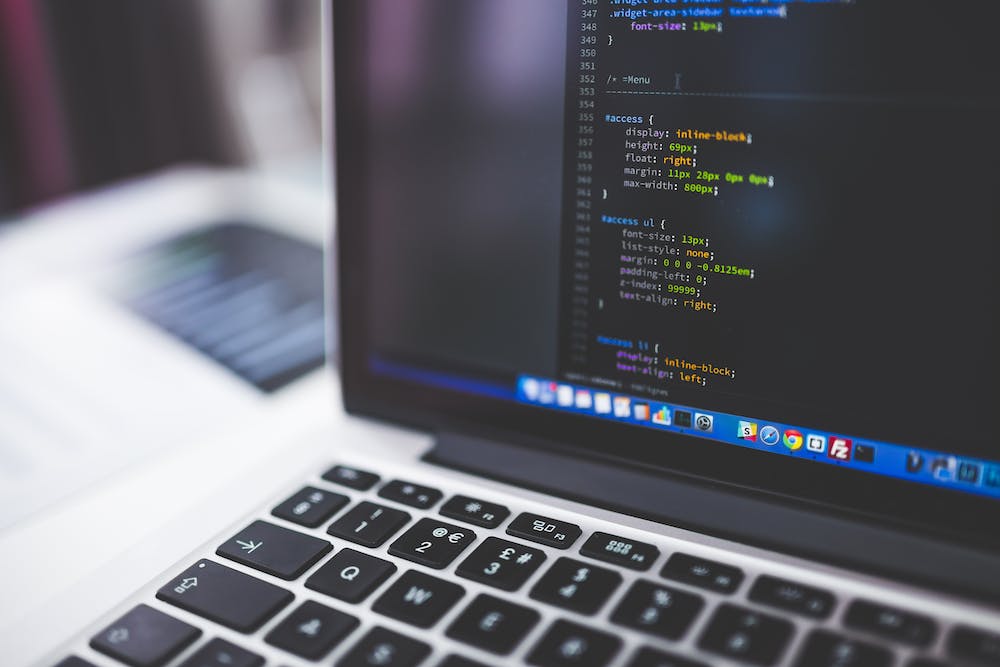
PHP is a popular server-side scripting language that is widely used for web development. One of the key data structures in PHP is the associative array. In this article, we will delve into the intricacies of associative arrays in PHP, understand their usage, and explore examples to demonstrate their functionality.
Understanding Associative Arrays
An associative array is a data structure that stores key-value pairs. Unlike numeric arrays, where the keys are automatically assigned by the system, associative arrays allow you to define your own keys and associate them with specific values. This provides a convenient way to organize and access data based on custom identifiers.
In PHP, associative arrays are created using the array() function or the shorthand square bracket notation []. The key-value pairs are specified using the => operator, with the key on the left and the value on the right. For example:
$student = array(
'name' => 'John Doe',
'age' => 20,
'grade' => 'A'
);
In this example, the keys are ‘name’, ‘age’, and ‘grade’, and the corresponding values are ‘John Doe’, 20, and ‘A’ respectively. This allows us to access specific information about the student using the associated keys.
Usage of Associative Arrays
Associative arrays are widely used in PHP for various purposes. Some common use cases include:
- Storing User Information: When building a user authentication system, associative arrays are used to store user data such as username, email, password, etc.
- Configuration Settings: Configuration settings for a web application can be stored in an associative array, allowing easy access and modification.
- Database Results: When retrieving data from a database, the results can be organized into an associative array for easy manipulation.
Accessing Associative Array Elements
Once an associative array is created, you can access its elements using the corresponding keys. This is done by specifying the key within square brackets after the array variable. For example:
echo $student['name']; // Output: John Doe
echo $student['age']; // Output: 20
Here, we are accessing the ‘name’ and ‘age’ elements of the $student array using their respective keys.
Adding and Modifying Elements
Associative arrays in PHP are dynamic, which means you can add new elements or modify existing ones at any point in the script. This can be achieved by simply assigning a value to a new key or an existing key. For example:
$student['grade'] = 'B'; // Modify the 'grade' value
$student['city'] = 'New York'; // Add a new 'city' key
After these operations, the $student array will have the updated ‘grade’ value and a new ‘city’ key-value pair.
Looping Through Associative Arrays
Iterating through an associative array is a common requirement in PHP. This can be accomplished using the foreach loop, which allows you to traverse all the key-value pairs in the array. For example:
foreach ($student as $key => $value) {
echo "$key: $value
";
}
This loop will output each key-value pair of the $student array in the format “key: value”.
Sorting Associative Arrays
PHP provides functions to sort associative arrays based on their keys or values. The ksort() function is used to sort an array by its keys in ascending order, while krsort() sorts the keys in descending order. Similarly, asort() and arsort() are used to sort the array by its values in ascending and descending order respectively.
For example:
ksort($student); // Sort by keys
asort($student); // Sort by values
Conclusion
Associative arrays are a powerful feature of PHP that allow developers to organize and manipulate data in a flexible and efficient manner. By using custom keys to access values, associative arrays offer a convenient way to represent complex data structures. Understanding how to create, access, modify, and loop through associative arrays is essential for any PHP developer, and mastering these skills can greatly improve the efficiency and readability of your code.
FAQs
Q: Can we have nested associative arrays in PHP?
A: Yes, you can have nested associative arrays in PHP. This allows you to create multi-dimensional data structures for organizing complex data.
Q: What is the difference between numeric and associative arrays?
A: In numeric arrays, the keys are automatically assigned as sequential numbers starting from 0, while in associative arrays, you can define your own custom keys to access the values.
Q: Are associative arrays memory intensive?
A: Associative arrays do require more memory compared to numeric arrays due to the storage of keys along with values. However, the difference is generally negligible for small to medium-sized arrays.