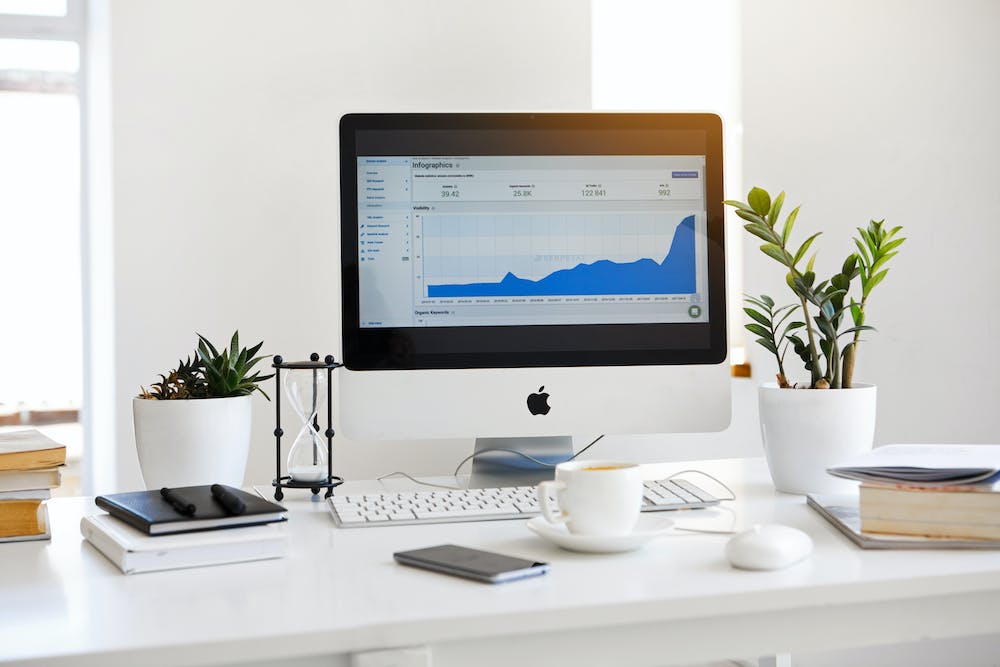
PHP, which stands for Hypertext Preprocessor, is a popular server-side scripting language used for web development. IT powers millions of websites and web applications on the internet. The release of PHP 8 brings with it a host of new features and upgrades, aimed at boosting performance and productivity for developers. In this article, we’ll take a closer look at some of the key features of PHP 8 and how they can benefit developers.
New Features of PHP 8
Named Arguments
Named arguments allow developers to specify function arguments by name, rather than by position. This can make function calls more readable and self-documenting, especially for functions with a large number of parameters. For example:
// Without named arguments
$result = calculateTotal(10, 5, true, 0.2);
// With named arguments
$result = calculateTotal(quantity: 10, price: 5, taxIncluded: true, taxRate: 0.2);
Union Types
Union types allow developers to specify that a parameter or return type can be one of several types. This can be useful when a function can accept multiple types of input, or when a function can return different types of values. For example:
function processValue(int|float $value): string|bool {
// function body
}
Attributes
Attributes provide a way to add metadata to classes, methods, and properties. They can be used for a variety of purposes, such as specifying validation rules, documenting code, or adding functionality to classes. For example:
#[Route('/products', methods: ['GET'])]
class ProductController {
#[Authorize]
public function index() {
// method body
}
}
Match Expression
The match expression is a more concise and readable alternative to the switch statement. It allows developers to compare a value against multiple possible options and return a result based on the first matching option. For example:
$result = match($status) {
'success' => 'Operation successful',
'fail' => 'Operation failed',
default => 'Operation status unknown',
};
Upgrades in PHP 8
Performance Improvements
PHP 8 introduces several performance improvements, including a new JIT (Just-In-Time) compiler. The JIT compiler converts PHP code into machine code at runtime, which can lead to significant performance gains for certain types of applications. In addition, PHP 8 includes optimizations for memory usage and garbage collection, further enhancing performance.
Constructor Property Promotion
Constructor property promotion is a new feature that allows developers to declare and initialize class properties directly in the constructor parameters. This can reduce boilerplate code and make class definitions more concise and readable. For example:
class Product {
public function __construct(
public string $name,
public float $price,
public int $quantity = 0,
) {}
}
Weak Maps
Weak maps are a new data structure in PHP 8 that allow developers to associate arbitrary data with objects without preventing the objects from being garbage collected. This can be useful for caching or memoization, where developers need to store additional information about objects without affecting their lifecycle.
Stricter Type Checking
PHP 8 introduces stricter type checking and more robust type inference, which can help developers catch type-related errors at compile time rather than at runtime. This can lead to more reliable and maintainable code, as well as improved IDE support for type-related features.
Conclusion
PHP 8 brings with it a wide range of new features and upgrades that can significantly enhance the performance and productivity of developers. From named arguments and union types to attributes and the match expression, PHP 8 offers a more modern and expressive syntax for writing clean and maintainable code. In addition, the performance improvements and stricter type checking in PHP 8 can lead to faster and more reliable applications. Developers are encouraged to upgrade to PHP 8 and take advantage of these new features to build better and more efficient web applications.
FAQs
Q: What is the release date for PHP 8?
A: PHP 8 was officially released on November 26, 2020.
Q: Is PHP 8 backward compatible with previous versions?
A: While PHP 8 includes many new features and improvements, it is not entirely backward compatible with previous versions. Some deprecated features have been removed, and certain backward incompatible changes have been introduced. Developers are advised to carefully review the migration guide before upgrading to PHP 8.
Q: Are there any performance benchmarks comparing PHP 8 to previous versions?
A: Yes, several performance benchmarks have been conducted to compare the performance of PHP 8 with previous versions. The results show significant performance improvements, particularly in CPU-intensive workloads, thanks to the new JIT compiler and other optimizations in PHP 8.
Q: Can I use PHP 8 for all my existing projects?
A: While PHP 8 offers many benefits, it’s important to consider the compatibility of existing projects before upgrading. Some third-party libraries and frameworks may not yet fully support PHP 8, so it’s essential to verify compatibility and conduct thorough testing before migrating existing projects to PHP 8.
Q: Where can I find more information about PHP 8 and its features?
A: The official PHP Website and documentation provide comprehensive information about PHP 8, including a migration guide, release notes, and detailed descriptions of new features and upgrades. Additionally, community forums and developer resources can offer valuable insights and best practices for leveraging PHP 8 in web development projects.