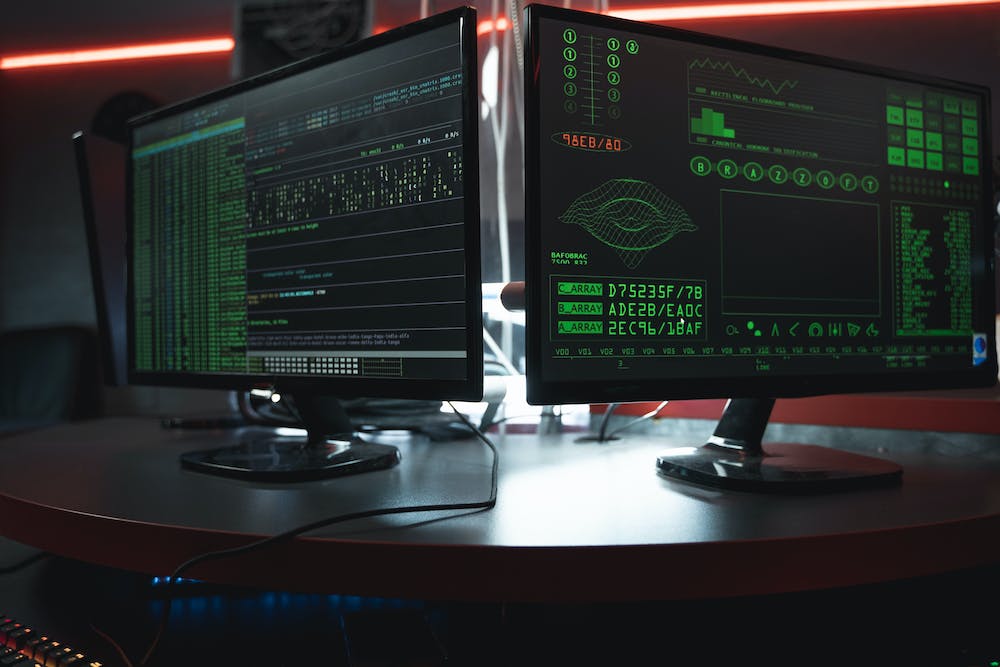
Python is a powerful programming language that is widely used for various applications, including data analysis, web development, and automation. One of the key aspects of programming in Python is working with time code. Time code manipulation is essential for tasks such as scheduling, logging, and data analysis. In this article, we will explore 5 essential tips that will help you master time code in Python.
1. Use the datetime Module
The datetime module in Python provides classes for manipulating dates and times. IT includes the datetime class, which allows you to create, manipulate, and format dates and times. The datetime module also provides functions for performing arithmetic operations on dates and times, such as adding or subtracting days, months, or years.
Here is an example of how to use the datetime module to work with dates and times:
“`python
import datetime
# Create a datetime object
now = datetime.datetime.now()
print(now)
# Format the datetime object
formatted_date = now.strftime(“%Y-%m-%d %H:%M:%S”)
print(formatted_date)
# Perform arithmetic operations
tomorrow = now + datetime.timedelta(days=1)
print(tomorrow)
“`
By using the datetime module, you can easily create, manipulate, and format dates and times in your Python programs.
2. Understand Time Zones and Daylight Saving Time
When working with time code in Python, it is important to understand time zones and daylight saving time. The pytz module provides an easy way to work with time zones in Python. It includes a timezone class that allows you to convert datetimes between different time zones.
Here is an example of how to use the pytz module to work with time zones:
“`python
import pytz
import datetime
# Create a timezone object
tz = pytz.timezone(‘America/New_York’)
# Convert a datetime to a different time zone
utc_now = datetime.datetime.now(pytz.utc)
eastern_now = utc_now.astimezone(tz)
print(eastern_now)
“`
By understanding time zones and daylight saving time, you can ensure that your Python programs are able to handle time code correctly, regardless of the user’s location.
3. Handling Time Code Formats
Python provides the strftime and strptime functions for formatting and parsing dates and times. The strftime function allows you to format a datetime object as a string, while the strptime function allows you to parse a string into a datetime object.
Here is an example of how to use the strftime and strptime functions:
“`python
import datetime
# Format a datetime object as a string
now = datetime.datetime.now()
formatted_date = now.strftime(“%Y-%m-%d %H:%M:%S”)
print(formatted_date)
# Parse a string into a datetime object
date_string = “2022-01-01 12:00:00”
parsed_date = datetime.datetime.strptime(date_string, “%Y-%m-%d %H:%M:%S”)
print(parsed_date)
“`
By leveraging the strftime and strptime functions, you can easily convert between datetime objects and string representations of dates and times in your Python programs.
4. Utilize Time Code Libraries
There are several third-party libraries available for working with time code in Python. These libraries provide additional functionality and convenience for handling dates and times in your programs. One such library is Pendulum, which offers a cleaner and more intuitive interface for working with dates and times compared to the standard datetime module.
Here is an example of how to use the Pendulum library to work with dates and times:
“`python
import pendulum
# Create a datetime object
now = pendulum.now()
print(now)
# Add or subtract time
tomorrow = now.add(days=1)
print(tomorrow)
# Convert to a different time zone
utc_now = now.in_timezone(‘UTC’)
print(utc_now)
“`
By utilizing time code libraries such as Pendulum, you can simplify and enhance the handling of dates and times in your Python programs.
5. Error Handling and Edge Cases
When working with time code in Python, it is important to consider error handling and edge cases. For example, certain operations such as adding or subtracting months or years from a date can result in unexpected behavior, especially when dealing with leap years and daylight saving time transitions.
It is essential to thoroughly test your time code logic and incorporate error handling for edge cases to ensure the reliability and correctness of your Python programs.
Conclusion
Mastering time code in Python is crucial for various applications, and the 5 essential tips covered in this article will help you efficiently work with dates and times in your programs. By utilizing the datetime module, understanding time zones, handling time code formats, leveraging time code libraries, and addressing error handling and edge cases, you can ensure the accuracy and reliability of your time-related operations in Python.
FAQs
What is the best way to handle time zones in Python?
The pytz module provides a convenient way to work with time zones in Python. It allows you to convert datetimes between different time zones and handle daylight saving time transitions.
Are there any recommended time code libraries for Python?
Yes, there are several recommended time code libraries for Python, including Pendulum and Arrow. These libraries offer enhanced functionality and convenience for working with dates and times compared to the standard datetime module.
How important is error handling when working with time code in Python?
Error handling is crucial when working with time code in Python, especially when dealing with edge cases such as leap years and daylight saving time transitions. Thorough testing and error handling can ensure the reliability and correctness of your time-related operations.