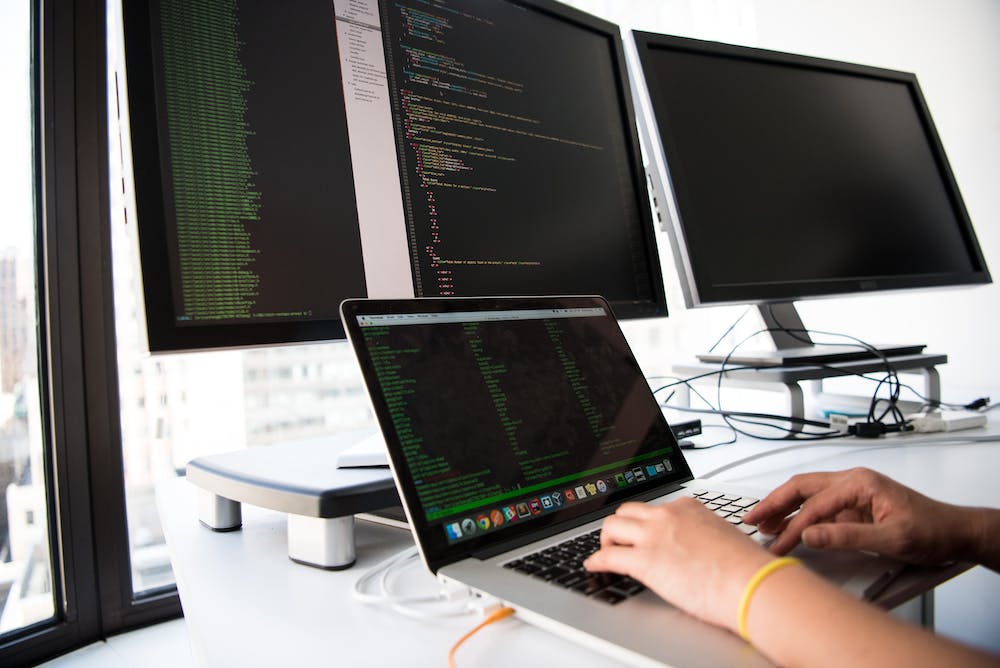
PHP is a popular programming language used to create dynamic web pages and applications. IT offers various features and functions to help developers write efficient and maintainable code. One such function is the require() statement, which allows you to include and execute external PHP files in your code. Mastering the require() statement is essential for writing modular and reusable code. In this article, we will explore best practices and tips for using the require() statement in PHP.
Understanding the require() Statement
The require() statement is used to include and execute an external PHP file in the current script. When using require(), if the specified file is not found or cannot be included for any reason, it will result in a fatal error, and the script execution will be halted. This is different from the include() statement, which generates a warning and allows the script to continue execution if the specified file is not found.
Here’s a basic example of using the require() statement in PHP:
“`php
require(‘config.php’);
// Other code goes here
?>
“`
In this example, the require() statement is used to include the ‘config.php’ file in the current script. If ‘config.php’ is not found, a fatal error will occur, and the script execution will stop.
Best Practices for Using require()
When working with the require() statement in PHP, there are several best practices to keep in mind to write clean and efficient code. Let’s explore some of these best practices:
1. Use require_once() for Non-Repeating Includes
If you need to include a file that contains functions, classes, or configuration settings, it’s best to use require_once() instead of require(). The require_once() statement ensures that the specified file is included only once, even if it’s called multiple times within the same script. This can prevent issues with redeclaring functions or classes and reduce unnecessary overhead.
2. Use Absolute Paths for Includes
When including files in your PHP scripts, it’s a good practice to use absolute paths instead of relative paths. Using absolute paths ensures that the included files are always found, regardless of the current working directory or file structure. You can use the $_SERVER[‘DOCUMENT_ROOT’] superglobal to construct absolute paths dynamically.
3. Organize Included Files in a Separate Directory
To improve code organization and maintainability, consider organizing your included files in a dedicated directory. This can help you easily locate and manage the files that are included in your scripts. It’s also a good practice to follow a naming convention for included files, such as prefixing them with ‘inc_’ or ‘include_’ to distinguish them from other files.
4. Validate Included Files
Before including a file in your PHP script, it’s important to validate the file path and ensure that the specified file exists. You can use conditional statements and file existence checks to handle possible errors and prevent fatal errors from occurring. Additionally, you can use built-in PHP functions like file_exists() and is_file() to perform file validations.
Tips for Using require()
In addition to best practices, there are some tips that can help you effectively use the require() statement in your PHP development. Let’s explore these tips:
1. Use Autoloading for Classes
If you are working with object-oriented PHP and have multiple class files, consider implementing an autoloader to automatically include class files when they are needed. PHP provides the spl_autoload_register() function, which allows you to register a custom autoloading function to handle class file inclusion on demand.
2. Optimize File Inclusion
When including files in your PHP scripts, it’s important to optimize file inclusion to minimize the impact on performance. You can use opcode caches like APC or OPcache to cache included files and improve script execution speed. Additionally, you can leverage the use of the realpath() function to determine the absolute path of included files and reduce the overhead of file system operations.
3. Monitor File Dependencies
As your codebase grows, it’s helpful to monitor and manage file dependencies to maintain a clear understanding of which files are being included in your scripts. You can use tools like PHP’s built-in get_included_files() function to retrieve a list of included files at runtime and identify any potential issues or redundancies.
Conclusion
Mastering the require() statement in PHP is crucial for writing modular, reusable, and efficient code. By following best practices and tips for using the require() statement, you can enhance the maintainability and performance of your PHP applications. Remember to use require_once() for non-repeating includes, use absolute paths for includes, organize included files in a separate directory, validate included files, use autoloading for classes, optimize file inclusion, and monitor file dependencies to achieve a well-structured and scalable codebase.
FAQs
Q: What is the difference between require() and include() in PHP?
A: The main difference between require() and include() in PHP is how they handle file inclusion errors. When using require(), a fatal error will occur if the specified file cannot be included. In contrast, include() generates a warning and allows the script to continue execution.
Q: When should I use require_once() instead of require()?
A: You should use require_once() when including files that contain functions, classes, or configuration settings that should not be redeclared. require_once() ensures that the specified file is included only once, even if it’s called multiple times within the same script.
Q: How can I optimize file inclusion in my PHP scripts?
A: To optimize file inclusion in your PHP scripts, you can use opcode caches like APC or OPcache to cache included files and improve script execution speed. Additionally, you can leverage the use of the realpath() function to determine the absolute path of included files and reduce the overhead of file system operations.