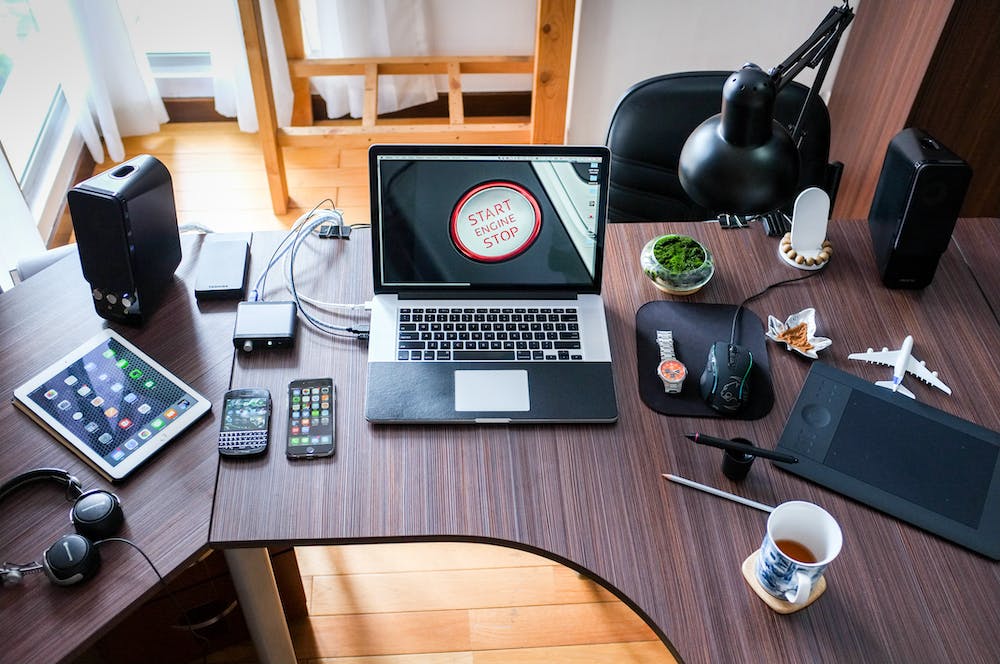
Mastering the PHP substr Function for String Manipulation
PHP is a powerful scripting language widely used for web development. One of the fundamental tasks in web development is manipulating strings – whether IT‘s extracting a portion of a string or simply finding a specific character. The PHP substr function comes to the rescue in such cases. This article will help you understand and master the substr function for efficient string manipulation.
What is the substr Function?
The PHP substr function allows you to extract a portion of a string based on a given starting position and length. Its syntax is as follows:
substr($string, $start, $length)
Where:
$string
is the input string from which you want to extract a portion$start
is the position in the string where the extraction should begin. IT can be zero-based or negative (from the end of the string)$length
is the number of characters to extract. If not specified, the function will extract everything from the starting position to the end of the string
Examples
Let’s dive into some practical examples to illustrate the powers of the substr function.
Example 1: Basic Usage
To extract a portion of a string, simply provide the starting position and length parameters:
$string = "Hello, World!";
$substring = substr($string, 7, 5);
The result, in this case, will be the substring “World”.
Example 2: Negative Start Position
You can also use a negative starting position to count from the end of the string:
$string = "The quick brown fox jumps over the lazy dog";
$substring = substr($string, -12);
In this example, the resulting substring will be “the lazy dog”.
Example 3: Omitting the Length Parameter
If you don’t specify the length parameter, the substr function will return everything from the starting position to the end of the string:
$string = "I love PHP!";
$substring = substr($string, 7);
The result here will be “PHP!” because we didn’t specify the length.
Conclusion
The substr function is a powerful tool for string manipulation in PHP. By mastering its usage, you can easily extract specific portions of strings based on your needs. Whether you want to obtain a substring, count characters from the end of a string, or simply extract everything after a specific position, the substr function has got you covered.
FAQs
Q1: Can I modify the original string with the substr function?
No, the substr function only extracts a substring from the original string. IT does not modify the original string in any way.
Q2: What happens if the start position is out of range?
If the start position you provide is out of range (either greater than or less than the length of the string), the substr function will return an empty string.
Q3: Can I use variables as arguments for the substr function?
Yes, the substr function allows you to use variables as arguments. This enables dynamic string manipulation based on user input or other factors.
Q4: Can I use substr to replace a portion of a string?
No, the substr function is only for extracting a portion of a string. If you want to replace a substring, you should use the str_replace or str_ireplace functions instead.
With the PHP substr function in your toolkit, you can easily manipulate strings to suit your needs. Understanding its usage and capabilities will greatly enhance your ability to work with strings in PHP.