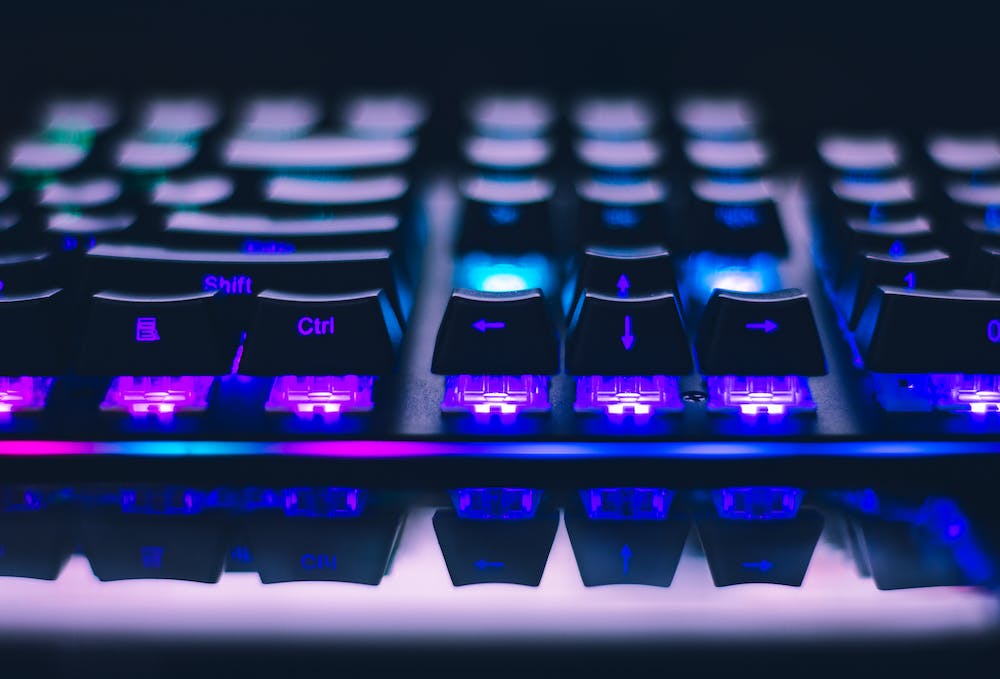
Random Forest is a powerful machine learning algorithm that is widely used for classification and regression tasks. IT is an ensemble learning method that operates by constructing a multitude of decision trees during training and outputting the class that is the mode of the classes (classification) or mean prediction (regression) of the individual trees. In this article, we will explore the magic of Random Forests and learn how to master IT with Python code.
Understanding Random Forests
Random Forest is an ensemble learning method that combines multiple decision trees to create a more robust and accurate model. The “random” in Random Forest comes from the fact that each tree is trained on a random subset of the training data, and at each split in the tree, a random subset of features is considered. This randomness helps to reduce overfitting and improve the generalization of the model.
The algorithm works by building multiple decision trees, each trained on a different subset of the data, and then combining the predictions of all the trees to make a final prediction. This approach helps to capture the variability and complex relationships in the data, making Random Forests a powerful and versatile algorithm.
Mastering Random Forests with Python
Python is a popular programming language for machine learning, and IT provides several libraries and tools for working with Random Forests. One of the most widely used libraries for building Random Forest models is scikit-learn.
Below is a simple example of how to train a Random Forest classifier using scikit-learn:
“`python
from sklearn.ensemble import RandomForestClassifier
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# Create a synthetic dataset for classification
X, y = make_classification(n_samples=1000, n_features=20, random_state=42)
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Initialize the Random Forest classifier
clf = RandomForestClassifier(n_estimators=100, random_state=42)
# Train the classifier on the training data
clf.fit(X_train, y_train)
# Make predictions on the testing data
y_pred = clf.predict(X_test)
# Evaluate the accuracy of the model
accuracy = accuracy_score(y_test, y_pred)
print(“Accuracy:”, accuracy)
“`
In this example, we first create a synthetic dataset for classification using the `make_classification` function from scikit-learn. We then split the dataset into training and testing sets using the `train_test_split` function. Next, we initialize a Random Forest classifier with 100 trees using the `RandomForestClassifier` class, and train the classifier on the training data. Finally, we make predictions on the testing data and evaluate the accuracy of the model.
Conclusion
Random Forests are a powerful and versatile machine learning algorithm that can be used for a wide range of tasks, including classification and regression. By combining multiple decision trees and leveraging the power of ensemble learning, Random Forests can capture complex relationships in the data and provide robust predictions. With the right tools and techniques, such as the scikit-learn library in Python, mastering the magic of Random Forests is within reach for any machine learning practitioner.
FAQs
What is the difference between a decision tree and a Random Forest?
A decision tree is a single tree-based model that makes predictions by recursively splitting the data into subsets based on the value of features. On the other hand, a Random Forest is an ensemble of multiple decision trees, where each tree is trained on a random subset of the data and a random subset of features. The predictions of all the trees are then combined to make a final prediction.
How does Random Forest prevent overfitting?
Random Forest prevents overfitting by training each tree on a random subset of the training data and by considering a random subset of features at each split. This introduces randomness and diversity into the trees, which helps to reduce overfitting and improve the generalization of the model.
Can Random Forest handle missing values?
Yes, Random Forest can handle missing values in the dataset. During the training process, the algorithm can use the available features to make decisions, even if some values are missing. However, IT is important to properly handle missing values before training the model to ensure optimal performance.
What are the key hyperparameters of Random Forest?
Some key hyperparameters of Random Forest include the number of trees in the forest (`n_estimators`), the maximum depth of the trees (`max_depth`), the minimum number of samples required to split a node (`min_samples_split`), and the maximum number of features to consider when looking for the best split (`max_features`). These hyperparameters can be tuned to optimize the performance of the model.
Is Random Forest suitable for high-dimensional data?
Yes, Random Forest can handle high-dimensional data effectively. The algorithm is able to capture complex relationships in the data, even when the number of features is high. However, IT is important to properly preprocess the data and tune the model’s hyperparameters to ensure good performance on high-dimensional datasets.