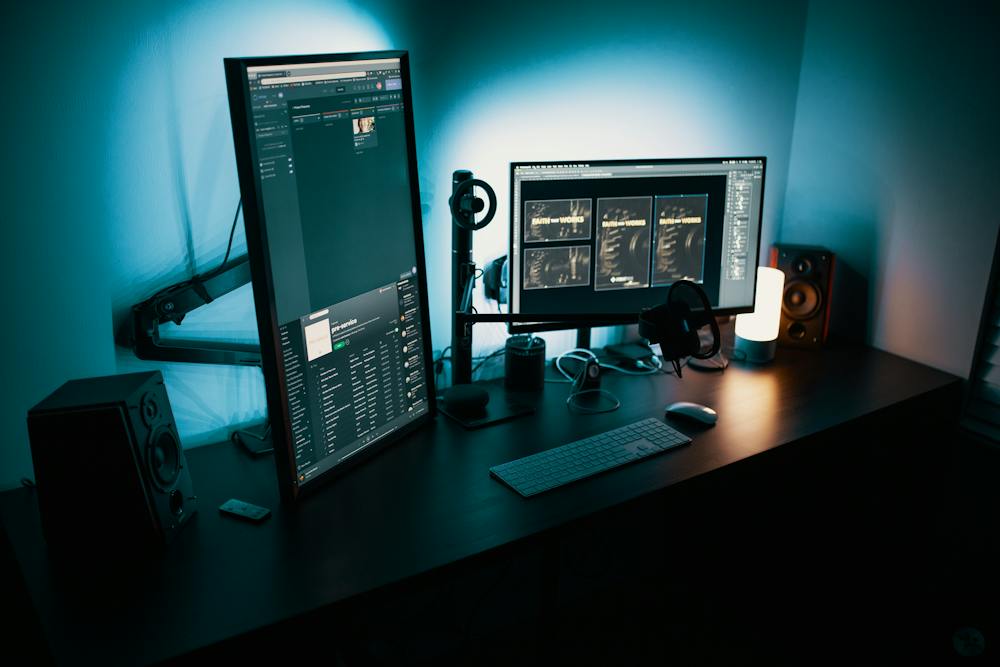
Rock Paper Scissors is a classic hand game that is played between two people. The game has simple rules: Rock beats Scissors, Scissors beats Paper, and Paper beats Rock. In this article, we will explore how to master the logic of the Rock Paper Scissors game using Python programming language.
Understanding the Logic
The first step in mastering the Rock Paper Scissors game in Python is to understand the logic behind IT. The game is essentially a combination of conditional statements and random selection. In Python, we can use the ‘if-elif-else’ statements to implement the game logic. We will also utilize the ‘random’ module to generate the computer‘s choice.
Implementing the Game Logic
Let’s start by implementing the game logic in Python. We will create a function that takes the player’s choice as input and compares it with the computer’s choice to determine the winner. Here’s a simple implementation:
def rock_paper_scissors(player_choice, computer_choice):
if player_choice == computer_choice:
return "It's a tie!"
elif (player_choice == "rock" and computer_choice == "scissors") or (player_choice == "scissors" and computer_choice == "paper") or (player_choice == "paper" and computer_choice == "rock"):
return "You win!"
else:
return "Computer wins!"
Integrating Random Selection
Now, let’s integrate the random selection of the computer’s choice using the ‘random’ module. We will create a list of choices (rock, paper, scissors) and use the ‘choice’ function to select a random item from the list. Here’s the updated implementation:
import random
def get_computer_choice():
choices = ["rock", "paper", "scissors"]
return random.choice(choices)
player_choice = input("Enter your choice (rock, paper, scissors): ").lower()
computer_choice = get_computer_choice()
result = rock_paper_scissors(player_choice, computer_choice)
print("Computer chose:", computer_choice)
print(result)
Optimizing the Game
While the basic implementation works, we can optimize the game by adding input validation and error handling. We can also create a simple user interface to make the game more interactive. By enhancing the user experience, we can elevate the overall gameplay.
Input Validation and Error Handling
To ensure that the player enters a valid choice, we can implement input validation and error handling in the game. We can prompt the player to re-enter their choice if it is not valid. Here’s an example of how to do this:
def get_player_choice():
valid_choices = ["rock", "paper", "scissors"]
while True:
player_choice = input("Enter your choice (rock, paper, scissors): ").lower()
if player_choice in valid_choices:
return player_choice
else:
print("Invalid choice. Please enter a valid choice.")
continue
Creating a User Interface
To enhance the user experience, we can create a simple user interface using the ‘tkinter’ module in Python. We can design a basic window with buttons for the player to make their choice and display the result of the game. This will make the game more visually appealing and engaging for the player.
Conclusion
Mastering the logic of the Rock Paper Scissors game in Python is an excellent exercise for beginners to learn about conditional statements, random selection, and user input handling. By implementing the game logic and optimizing the game with input validation and a user interface, we can create a fun and interactive game experience for players.
FAQs
Q: Can I use this implementation for a web-based version of the game?
A: Yes, you can adapt the Python implementation for a web-based version using frameworks such as Flask or Django. You can create an API for the game logic and integrate it into a web application.
Q: Are there other variations of the game that I can explore?
A: Yes, there are many variations of the Rock Paper Scissors game, such as Rock Paper Scissors Lizard Spock, which adds two more choices to the game. You can also create your own custom variations to make the game more interesting.
Q: How can I improve the game further?
A: You can improve the game further by adding score tracking, multiplayer support, and advanced game modes. You can also explore incorporating machine learning to create a more challenging computer opponent.