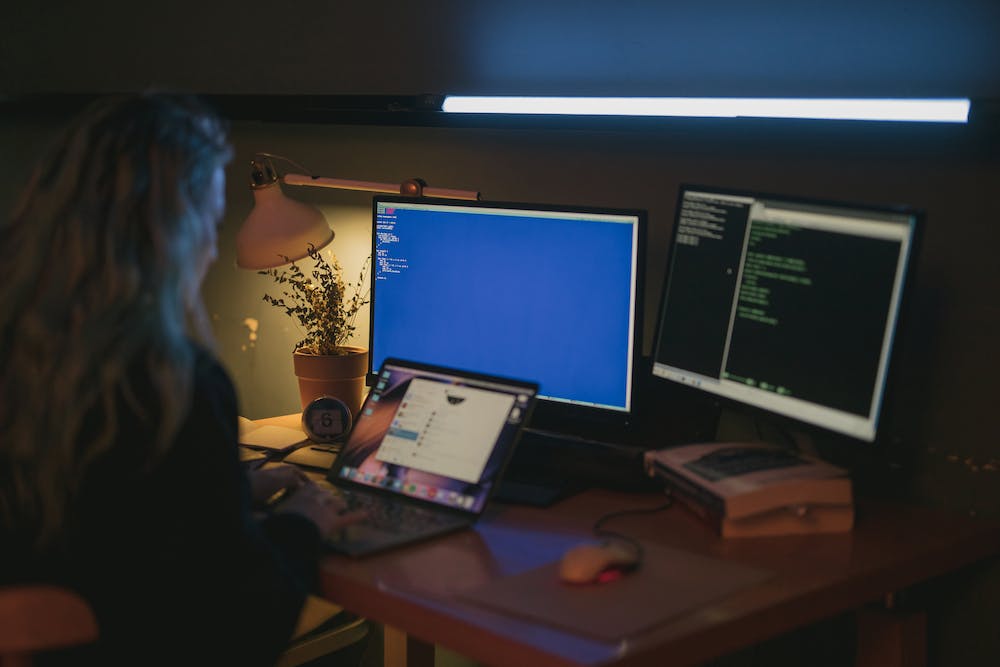
PHP, which stands for Hypertext Preprocessor, is a popular general-purpose scripting language that is especially suited for web development. IT is widely used and versatile, making it an essential language to master for anyone interested in web development. One of the first things you learn in any programming language is how to output the famous “Hello, World!” message. In this article, we will explore the basics of PHP and how to create a “Hello, World!” program.
Getting Started with PHP
Before we dive into creating our “Hello, World!” program, let’s first make sure we have PHP installed on our system. PHP is a server-side scripting language, so it needs to be installed on a web server to function. If you don’t have a web server set up, you can use a local development environment like XAMPP, WAMP, or MAMP to run PHP on your computer. Once you have PHP installed, you can create and run PHP scripts from a text editor and a web browser.
To check if PHP is installed on your system, open a command prompt or terminal and type the following command:
php -v
If PHP is installed, you should see the version number of the installed PHP along with other details. If PHP is not installed, you can download it from the official PHP Website (https://www.php.net/downloads) and follow the installation instructions for your operating system.
Creating a “Hello, World!” Program
Now that we have PHP installed, let’s create a simple “Hello, World!” program. Open a text editor and create a new file with a .php extension, for example, hello.php. In this file, we will write a few lines of code to output the “Hello, World!” message to the browser.
<?php
echo "Hello, World!";
?>
Save the file and open it in a web browser by entering the URL to the file in the address bar. If you are using a local development environment, the URL might look like http://localhost/hello.php. When you open the file in the browser, you should see the “Hello, World!” message displayed on the page.
Congratulations! You have just created and executed your first PHP program. It may seem simple, but understanding the “Hello, World!” program is the first step in mastering the basics of PHP.
Understanding the “Hello, World!” Program
Let’s break down the code we just wrote to understand what each part does:
- <?php: This is the opening tag for PHP code. It tells the web server to start interpreting and executing PHP code.
- echo: This is a PHP language construct used to output text. In this case, we are using it to output the “Hello, World!” message to the browser.
- “Hello, World!”: This is the text we want to output to the browser. It is enclosed in double quotes to indicate that it is a string.
- ?>: This is the closing tag for PHP code. It tells the web server to stop interpreting and executing PHP code.
That’s it! The “Hello, World!” program may seem straightforward, but it lays the foundation for more complex PHP programs that you will write in the future.
Enhancing the “Hello, World!” Program
Now that you have a basic understanding of how to create a “Hello, World!” program in PHP, let’s explore some ways to enhance it. You can add HTML markup, variables, and even create functions to make your program more dynamic and interactive.
For example, you can combine PHP with HTML to create a more structured and visually appealing “Hello, World!” program:
<html>
<body>
<?php
$message = "Hello, World!";
echo "<h1>" . $message . "</h1>";
?>
</body>
</html>
In this example, we have stored the “Hello, World!” message in a variable called $message and used it to dynamically create an HTML heading element. This demonstrates how PHP can be integrated with HTML to create dynamic and customizable web pages.
Conclusion
Mastering the basics of PHP starts with understanding how to create a “Hello, World!” program. By following the steps in this article, you have learned how to set up a PHP environment, create a simple PHP script, and execute it in a web browser. Understanding the fundamentals of PHP is essential for anyone looking to pursue a career in web development or programming.
As you continue your journey with PHP, you will discover its vast capabilities and features that make it a powerful scripting language for web development. From dynamic web applications to e-commerce platforms, PHP is a versatile language that can handle a wide range of tasks and challenges.
FAQs
Q: Can I run PHP without a web server?
A: No, PHP is a server-side scripting language, so it needs to be run on a web server to function properly. However, you can use a local development environment like XAMPP, WAMP, or MAMP to run PHP on your computer for testing and development purposes.
Q: What is the purpose of the “Hello, World!” program?
A: The “Hello, World!” program is a traditional introductory program that is used to demonstrate the basic syntax of a programming language. It serves as a simple example to help beginners understand how to write, execute, and troubleshoot code in a new language.
Q: Can I use PHP for more than just web development?
A: Yes, PHP was originally designed for web development, but it has since evolved to support a wide range of applications, including command-line scripting, desktop applications, and even mobile app development.
Thank you for reading our article on mastering the basics of PHP and creating a “Hello, World!” program. We hope you found the information valuable and that it has given you a solid foundation for further exploration of PHP and web development. Happy coding!