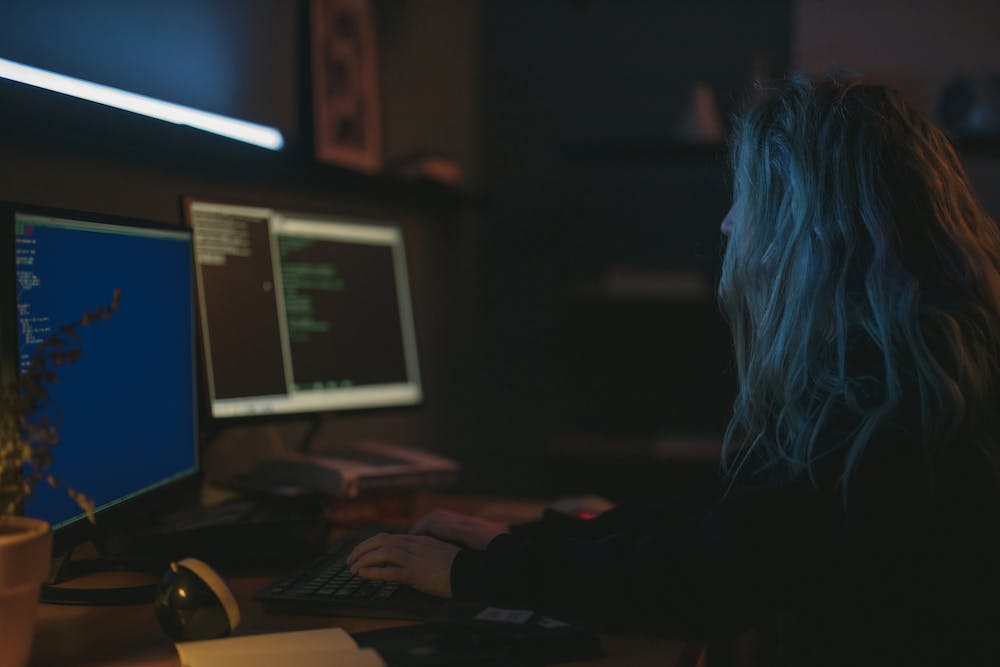
Python is one of the most popular programming languages in the world. IT is widely used for web development, data analysis, artificial intelligence, and more. If you’re looking to land a job as a Python developer or want to advance your career, mastering the basics of Python is essential. In this ultimate guide, we’ll cover everything you need to know to nail your Python interview and excel in your career.
The Basics of Python
Python is known for its simplicity and readability, making it a great language for beginners. It is an interpreted language, which means that the code is executed line by line. Python uses indentation to delimit blocks of code, making it easy to read and understand.
One of the key features of Python is its extensive standard library, which includes modules and packages for tasks such as string manipulation, file I/O, and more. Python also has a large and active community, which means that there are plenty of resources available for learning and problem-solving.
Variables and Data Types
In Python, variables are used to store data. Variables can store different types of data, such as numbers, strings, and lists. Python has several built-in data types, including integers, floats, strings, and booleans. Understanding how to work with these data types is essential for writing effective Python code.
# Example of variables and data types
age = 25
name = "John"
height = 6.2
is_student = True
hobbies = ["hiking", "reading", "coding"]
Control Flow and Loops
Python supports various control flow statements, such as if, else, and elif, for making decisions in your code. Loops, such as for and while loops, are used to iterate over sequences of data. Understanding how to use control flow and loops is crucial for writing efficient and readable Python code.
# Example of control flow and loops
if age >= 18:
print("You are an adult")
else:
print("You are a minor")
for hobby in hobbies:
print("I enjoy " + hobby)
Functions and Modules
Functions are essential for organizing and reusing code in Python. They allow you to break your code into smaller, more manageable pieces. Python also supports the use of modules, which are files containing Python code that can be imported into your programs. Understanding how to create and use functions and modules is crucial for writing modular and maintainable Python code.
# Example of functions and modules
def greet(name):
print("Hello, " + name)
import math
print(math.sqrt(16))
Exception Handling
Python supports exception handling, which allows you to gracefully handle errors that may occur in your code. Understanding how to use try, except, and finally blocks is essential for writing robust Python code that can handle unexpected situations.
# Example of exception handling
try:
result = 10 / 0
except ZeroDivisionError:
print("Cannot divide by zero")
finally:
print("Exiting the program")
Advanced Topics
Once you have mastered the basics of Python, there are several advanced topics that you can explore to further enhance your skills. These topics include object-oriented programming, data structures, file handling, and more. The key to mastering these topics is practice and continuous learning.
Preparing for Your Python Interview
Now that you have a solid understanding of the basics of Python, it’s time to prepare for your interview. Here are some tips to help you nail your Python interview:
Understand the Job Requirements
Before your interview, make sure to carefully review the job requirements and understand the specific skills and experiences that the employer is looking for. This will help you tailor your responses to demonstrate how your skills and experiences align with the job requirements.
Practice Coding Problems
Many Python interviews include coding problems or challenges. Practicing coding problems on platforms like LeetCode, HackerRank, or backlink works can help you sharpen your problem-solving skills and become more comfortable with coding under pressure.
Prepare for Behavioral Questions
In addition to technical questions, Python interviews may also include behavioral questions to assess your personality, work ethic, and problem-solving approach. Prepare for these types of questions by thinking of examples from your past experiences that demonstrate your ability to work in a team, handle conflicts, or overcome challenges.
Ask Questions
At the end of the interview, you will likely have the opportunity to ask the interviewer questions. Prepare a list of thoughtful questions about the company, the team, or the role to show your interest in the position and gain a better understanding of the company culture and expectations.
Conclusion
Mastering the basics of Python is essential for anyone looking to excel in a Python-related role. By understanding variables and data types, control flow and loops, functions and modules, and exception handling, you will have a solid foundation to build upon. Additionally, preparing for your Python interview is crucial to demonstrate your skills and experiences effectively. With the right preparation and practice, you can confidently showcase your Python skills and nail your interview!
FAQs
1. What is Python?
Python is a high-level, interpreted programming language known for its simplicity and readability. It is widely used for web development, data analysis, artificial intelligence, and more.
2. What are the basic data types in Python?
Python has several built-in data types, including integers, floats, strings, and booleans.
3. How can I prepare for a Python interview?
To prepare for a Python interview, make sure to review the job requirements, practice coding problems, prepare for behavioral questions, and ask thoughtful questions at the end of the interview.
By following the advice and tips outlined in this ultimate guide, you can confidently nail your Python interview and advance your career in the exciting world of Python development!