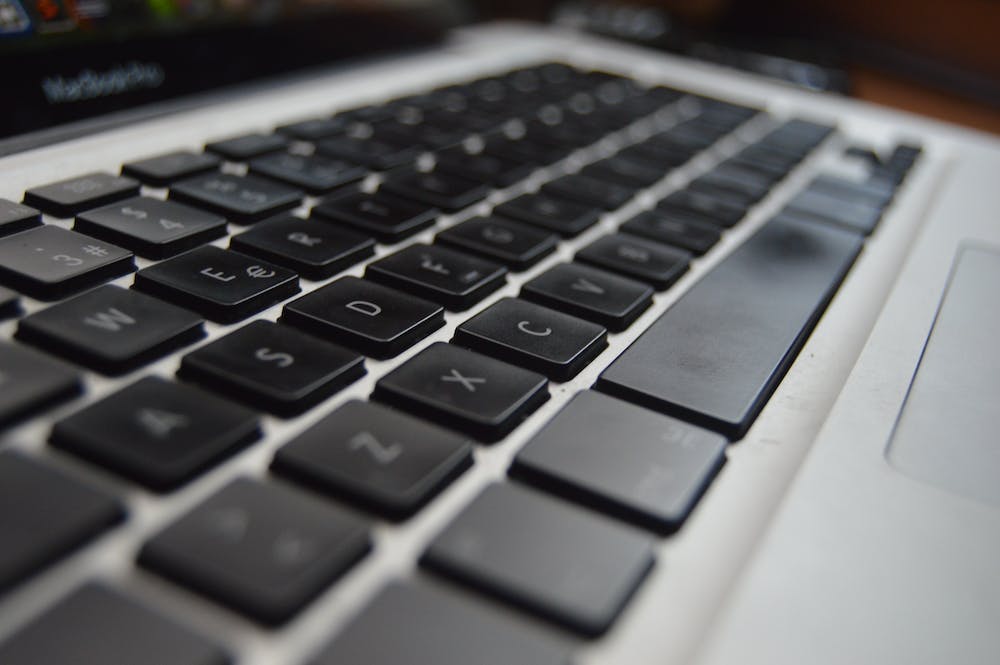
PHP is a powerful scripting language that is widely used for web development. IT provides various features and functions to create dynamic and interactive web pages. One of the essential features of PHP is the ability to send emails directly from a web application. In this article, we will explore how to send mail with just a few simple steps using PHP.
Setting Up the Environment
Before we start sending emails using PHP, we need to ensure that our environment is set up correctly. PHP comes with a built-in mail function that allows us to send emails directly from the server. However, to use this function, we need to have access to a mail server. Most web hosting providers offer SMTP (Simple Mail Transfer Protocol) servers that allow you to send emails using PHP.
To set up the environment, you need to have a web server with PHP installed. You also need to have access to an SMTP server. If you are using a web hosting service, you can usually find the SMTP server details in the hosting dashboard. If you are setting up a local development environment, you can use tools like Mailtrap or Mailhog to simulate an SMTP server on your local machine.
Sending Emails with PHP
Once the environment is set up, we can start sending emails using the built-in mail function in PHP. The mail function takes several parameters, including the recipient’s email address, the subject of the email, the body of the email, and optional headers such as CC, BCC, and additional headers like From, Reply-To, etc.
Here’s a simple example of how to send an email using PHP:
<?php
$to = "[email protected]";
$subject = "Test Email";
$message = "This is a test email sent from PHP.";
$headers = "From: [email protected]";
if(mail($to, $subject, $message, $headers)) {
echo "Email sent successfully";
} else {
echo "Email sending failed";
}
?>
In this example, we specify the recipient’s email address, the subject of the email, the body of the email, and the sender’s email address in the headers. We then use the mail function to send the email. If the email is sent successfully, we display a success message. Otherwise, we display an error message.
Handling Email Attachments
In addition to sending plain text emails, PHP also allows us to send email attachments. This can be useful when you want to send files such as documents, images, or audio files through email. To send an email with an attachment, we need to use the MIME (Multipurpose internet Mail Extensions) type when constructing the email message.
Here’s an example of how to send an email with an attachment using PHP:
<?php
$to = "[email protected]";
$subject = "Test Email with Attachment";
$message = "This is a test email with an attachment sent from PHP.";
$file = "path/to/attachment.pdf";
$filename = "attachment.pdf";
$file_content = file_get_contents($file);
$file_content = chunk_split(base64_encode($file_content));
$boundary = md5(uniqid(time()));
$headers = "From: [email protected]\r\n";
$headers .= "MIME-Version: 1.0\r\n";
$headers .= "content-Type: multipart/mixed; boundary=\"{$boundary}\"";
$body = "--{$boundary}\r\n";
$body .= "Content-Type: text/plain; charset=\"utf-8\"\r\n";
$body .= "Content-Transfer-Encoding: 7bit\r\n";
$body .= "\r\n";
$body .= $message."\r\n";
$body .= "--{$boundary}\r\n";
$body .= "Content-Type: application/octet-stream; name=\"{$filename}\"\r\n";
$body .= "Content-Transfer-Encoding: base64\r\n";
$body .= "Content-Disposition: attachment; filename=\"{$filename}\"\r\n";
$body .= "\r\n";
$body .= $file_content."\r\n";
$body .= "--{$boundary}--";
if(mail($to, $subject, $body, $headers)) {
echo "Email with attachment sent successfully";
} else {
echo "Email with attachment sending failed";
}
?>
In this example, we first read the file content and encode it using base64. We then construct the email message with the attachment using the MIME type. Finally, we use the mail function to send the email.
Handling HTML Emails
PHP also allows us to send HTML-formatted emails. This can be useful when you want to send rich-content emails with images, links, and styling. To send an HTML email, we need to include the appropriate headers and construct the email message using HTML tags.
Here’s an example of how to send an HTML-formatted email using PHP:
<?php
$to = "[email protected]";
$subject = "Test HTML Email";
$message = "<html><body><h1>This is a test email with HTML content sent from PHP</h1><p>This email includes images, links, and styling.</p></body></html>";
$headers = "From: [email protected]\r\n";
$headers .= "MIME-Version: 1.0\r\n";
$headers .= "Content-Type: text/html; charset=UTF-8\r\n";
if(mail($to, $subject, $message, $headers)) {
echo "HTML Email sent successfully";
} else {
echo "HTML Email sending failed";
}
?>
In this example, we include the appropriate headers for sending HTML content and construct the email message using HTML tags. We then use the mail function to send the HTML-formatted email.
Conclusion
Sending emails using PHP is a valuable skill for web developers. Whether you need to send transactional emails, newsletters, or notifications, mastering the art of PHP mail function can greatly enhance your web development capabilities. By following the simple steps outlined in this article, you can learn how to send plain text emails, handle email attachments, and send HTML-formatted emails with ease. With a solid understanding of PHP, you can harness the power of email communication in your web applications.
FAQs
Q: Do I need a mail server to send emails using PHP?
A: Yes, you need access to an SMTP server to send emails using PHP. Most web hosting providers offer SMTP servers as part of their hosting services.
Q: Can I send email attachments using PHP mail function?
A: Yes, you can send email attachments using PHP mail function by utilizing the MIME type and constructing the email message accordingly.
Q: Is it possible to send HTML-formatted emails with PHP?
A: Yes, you can send HTML-formatted emails with PHP by including the appropriate headers and constructing the email message using HTML tags.