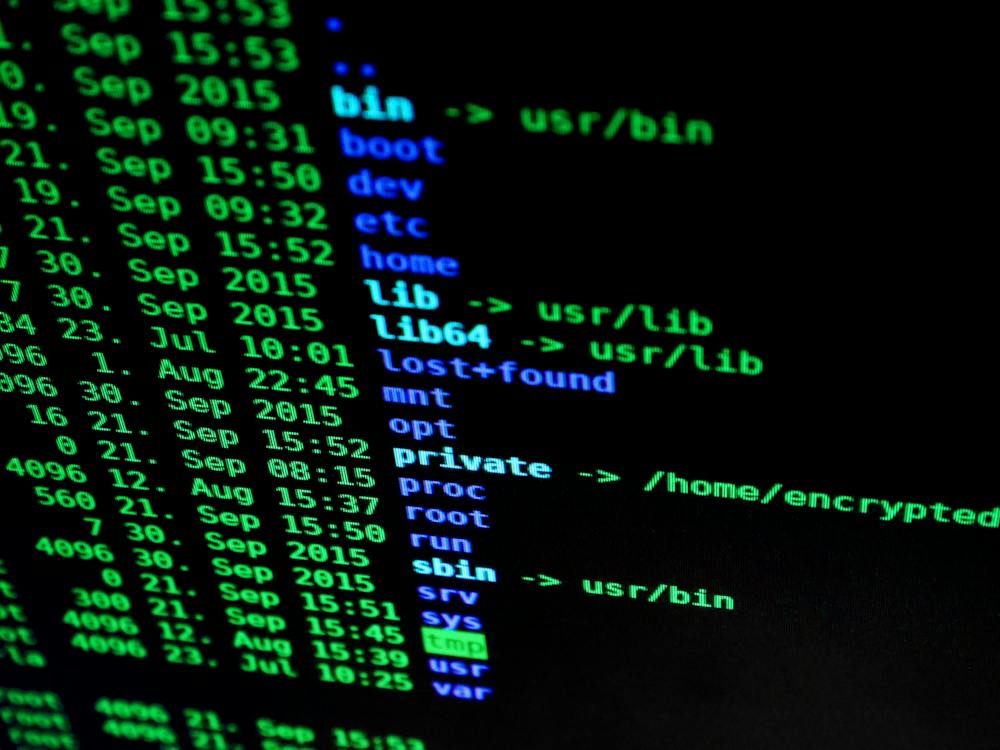
Understanding the confusion matrix is an important aspect of evaluating the performance of a machine learning model. IT provides a detailed breakdown of the model’s predictions and can help identify areas for improvement. In this article, we will explore the confusion matrix and how to use Python to analyze IT.
What is a confusion matrix?
A confusion matrix is a table that is used to describe the performance of a classification model. IT compares the actual values of the target variable with the values predicted by the model. The matrix is particularly useful for evaluating the performance of binary classification models, where there are only two possible outcomes (e.g., true/false, positive/negative).
The matrix itself is a 2×2 table, with four possible combinations of predicted and actual values:
- True Positive (TP): The model correctly predicts a positive outcome.
- True Negative (TN): The model correctly predicts a negative outcome.
- False Positive (FP): The model incorrectly predicts a positive outcome.
- False Negative (FN): The model incorrectly predicts a negative outcome.
Using Python to analyze the confusion matrix
Python provides a number of libraries for working with data and machine learning models. One of the most popular libraries for this purpose is scikit-learn, which provides tools for building and evaluating machine learning models. Let’s look at a simple example of using scikit-learn to create a confusion matrix.
“`python
# Importing the necessary libraries
from sklearn.metrics import confusion_matrix
import numpy as np
# Generating random sample data
y_true = np.array([1, 0, 1, 1, 0, 1, 0, 0, 1, 1])
y_pred = np.array([1, 1, 0, 1, 0, 1, 0, 0, 1, 0])
# Creating the confusion matrix
cm = confusion_matrix(y_true, y_pred)
print(cm)
“`
In this example, we first import the `confusion_matrix` function from scikit-learn and the `numpy` library, which we will use to create the sample data. We then create two arrays, `y_true` and `y_pred`, which represent the actual and predicted values, respectively. Finally, we use the `confusion_matrix` function to create the confusion matrix and print the result.
The output of the code will be:
“`
[[4 1]
[2 3]]
“`
This output represents the four possible combinations of predicted and actual values. From the matrix, we can see that there are 4 true positives, 1 false positive, 2 false negatives, and 3 true negatives.
Interpreting the confusion matrix
Once the confusion matrix has been generated, IT can be used to calculate a number of metrics that provide insight into the performance of the model. These metrics include:
- Accuracy: The proportion of correct predictions out of the total number of predictions.
- Precision: The proportion of true positive predictions out of the total number of positive predictions.
- Recall: The proportion of true positive predictions out of the total number of actual positive values.
- F1 Score: The harmonic mean of precision and recall, which provides a balanced measure of a model’s performance.
These metrics can be calculated using the values in the confusion matrix, and can provide valuable insights into the strengths and weaknesses of the model.
Conclusion
The confusion matrix is an important tool for evaluating the performance of a classification model. By using Python and libraries like scikit-learn, IT is possible to quickly and easily generate a confusion matrix and use IT to calculate key performance metrics. Understanding and mastering the confusion matrix can help data scientists and machine learning practitioners to identify areas for improvement and make informed decisions about model performance.
FAQs
What is the purpose of a confusion matrix?
The confusion matrix is used to evaluate the performance of a classification model by comparing the actual values of the target variable with the values predicted by the model. IT provides a detailed breakdown of the model’s predictions and can help identify areas for improvement.
What are some common metrics derived from the confusion matrix?
Some common metrics derived from the confusion matrix include accuracy, precision, recall, and F1 score. These metrics provide insight into the performance of the model and can help identify areas for improvement.
How can Python be used to analyze the confusion matrix?
Python provides a number of libraries, such as scikit-learn, for working with data and machine learning models. These libraries can be used to quickly and easily generate a confusion matrix and calculate key performance metrics.