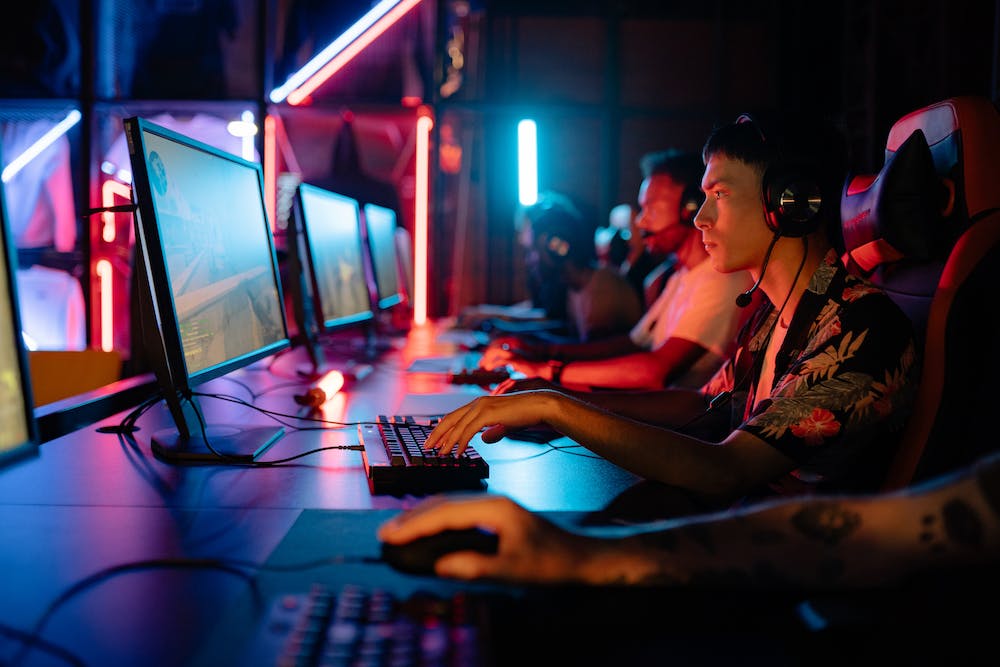
Binary search is a fundamental algorithm in computer science that allows you to efficiently search for a specific element in a sorted array. With the right implementation, IT can drastically reduce the time complexity of search operations, making it a valuable tool for any programmer.
Understanding Binary Search
Before we dive into the Python program that implements binary search, let’s first understand how binary search works. The basic idea behind binary search is to divide the array into two halves and then repeatedly narrow down the search interval until the desired element is found. This approach takes advantage of the fact that the array is sorted, allowing us to eliminate half of the remaining elements in each step.
Here’s a high-level overview of the binary search algorithm:
- Initialize two pointers,
left
andright
, to mark the beginning and end of the search interval. - While
left
is less than or equal toright
, calculate the midpoint of the interval asmid = (left + right) / 2
. - If the midpoint element is equal to the target element, return its index.
- If the midpoint element is less than the target element, update
left = mid + 1
to search the right half of the interval. - If the midpoint element is greater than the target element, update
right = mid - 1
to search the left half of the interval.
By following these steps, binary search can quickly locate the desired element in the array with a time complexity of O(log n), where n is the number of elements in the array.
Implementing Binary Search in Python
Now that we have a solid understanding of how binary search works, let’s take a look at a Python program that implements the binary search algorithm. This program will accept a sorted array and a target element as input, and return the index of the target element if it exists in the array.
“`python
def binary_search(arr, target):
left, right = 0, len(arr) – 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid – 1
return -1
“`
In this Python program, the binary_search
function takes two parameters: arr
, which is the sorted array, and target
, which is the element we want to search for. The function then uses the binary search algorithm to locate the target element and return its index if found, or -1 if not found.
Testing the Program
Let’s test the binary_search
function with a sample input to see how it performs. We’ll use the following sorted array and target element:
“`python
arr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
target = 5
result = binary_search(arr, target)
print(result) # Output: 4
“`
As expected, the program correctly returns the index of the target element, which is 4. This demonstrates the efficiency and accuracy of the binary search algorithm when implemented in Python.
Optimizing the Program
While the basic binary search algorithm works well for most cases, there are opportunities for optimization to further improve its performance. For example, you can enhance the program to handle edge cases, such as when the array is empty or when the target element is not present in the array. Additionally, you can refine the implementation to make it more readable and maintainable by using helper functions and error handling.
Here’s an optimized version of the binary_search
function that includes error handling and edge case checks:
“`python
def binary_search(arr, target):
if not arr:
return -1
left, right = 0, len(arr) – 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid – 1
return -1
“`
By incorporating these optimizations, the program becomes more robust and can handle a wider range of input scenarios, further solidifying its efficiency and reliability.
Conclusion
Mastering the art of binary search is a valuable skill for any programmer, and with the right Python program, you can harness the efficiency and power of this algorithm in your own projects. By understanding the principles behind binary search and implementing it in Python, you can effectively search for elements in sorted arrays with minimal time complexity, making your code faster and more efficient.
FAQs
1. What is the time complexity of binary search?
The time complexity of binary search is O(log n), where n is the number of elements in the array. This makes binary search significantly more efficient than linear search algorithms, especially for large datasets.
2. Can binary search be used for non-numeric data?
Yes, binary search can be used for non-numeric data as long as the elements are comparable and the array is sorted according to a defined order. This allows binary search to quickly locate specific elements, regardless of their data type.
3. Are there any drawbacks to using binary search?
While binary search offers exceptional efficiency, it does require the array to be sorted beforehand. This means that any modifications to the array, such as insertions or deletions, will necessitate re-sorting before performing binary search operations, which can incur additional overhead.