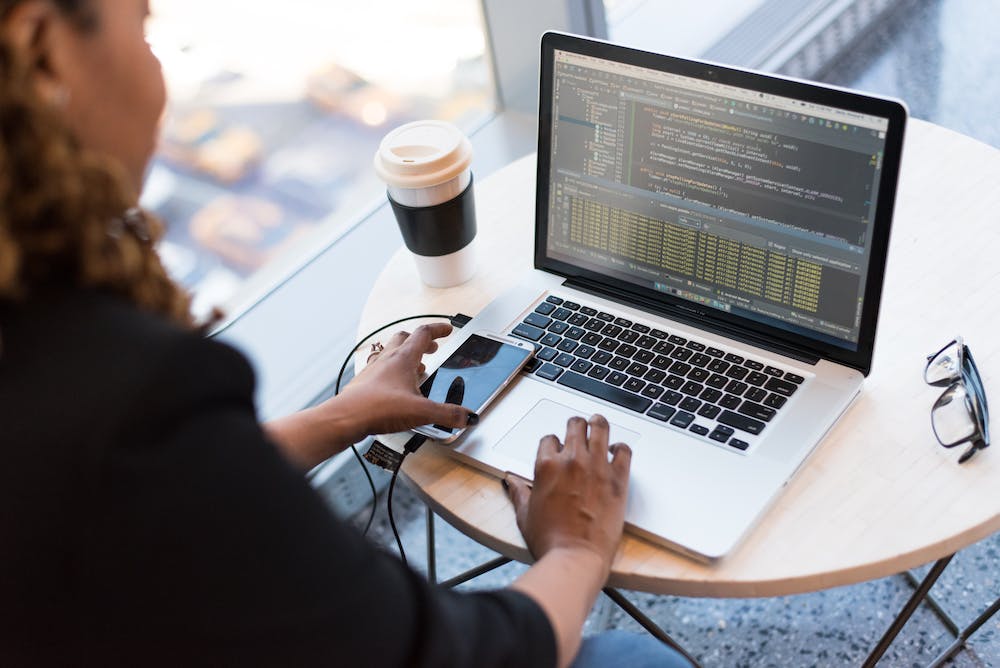
One of the most common tasks when working with PHP is converting a string into an array. This can be especially useful when you have a string that contains multiple values separated by a specific character or delimiter. By mastering the string to array conversion in PHP, you’ll be able to leverage the power of arrays to easily manipulate and work with data in your applications. In this article, we’ll explore different ways to convert a string into an array in PHP.
Using the explode() Function
One of the simplest and most effective ways to convert a string into an array in PHP is by using the explode() function. This function allows you to split a string into an array based on a specified delimiter character. Here’s how you can use the explode() function:
$string = "apple,banana,orange";
$array = explode(",", $string);
In this example, the explode() function takes two parameters: the delimiter (in this case, a comma) and the string that you want to convert into an array. The result is an array containing each value separated by the delimiter.
Converting a String with Multiple Delimiters
What if your string contains multiple delimiters? In that case, you can use the preg_split() function, which allows you to split a string into an array using a regular expression pattern. Here’s an example:
$string = "apple,banana;orange";
$array = preg_split("/[,;]/", $string);
In this example, the regular expression pattern “/[,;]/” matches either a comma or a semicolon. The preg_split() function splits the string into an array using this pattern as the delimiter.
Converting a String with Key-Value Pairs
Sometimes, you may have a string that contains key-value pairs, such as “name=John&age=25&city=NewYork”. To convert this string into an associative array, you can use the parse_str() function. Here’s an example:
$string = "name=John&age=25&city=NewYork";
parse_str($string, $array);
After executing this code, the $array variable will contain the following associative array:
[
'name' => 'John',
'age' => '25',
'city' => 'NewYork'
]
Frequently Asked Questions
Q: Can I use a different character as the delimiter?
A: Yes, you can use any character as the delimiter. Simply change the delimiter parameter in the explode() or preg_split() function to your desired character.
Q: What if my string contains leading or trailing spaces?
A: If your string contains leading or trailing spaces, you can use the trim() function to remove them before converting the string into an array.
Q: How do I handle empty values?
A: By default, if a value is empty between two delimiters, an empty string will be included in the resulting array. However, you can use the array_filter() function to remove empty values from the array.
Q: Can I convert an array back into a string?
A: Yes, you can convert an array back into a string by using the implode() function. This function takes an array and concatenates its values into a string using a specified delimiter.
With these techniques and tips, you can now master the art of converting a string into an array in PHP. Whether you’re dealing with simple comma-separated values or complex key-value pairs, understanding how to convert strings into arrays will greatly enhance your PHP programming skills.