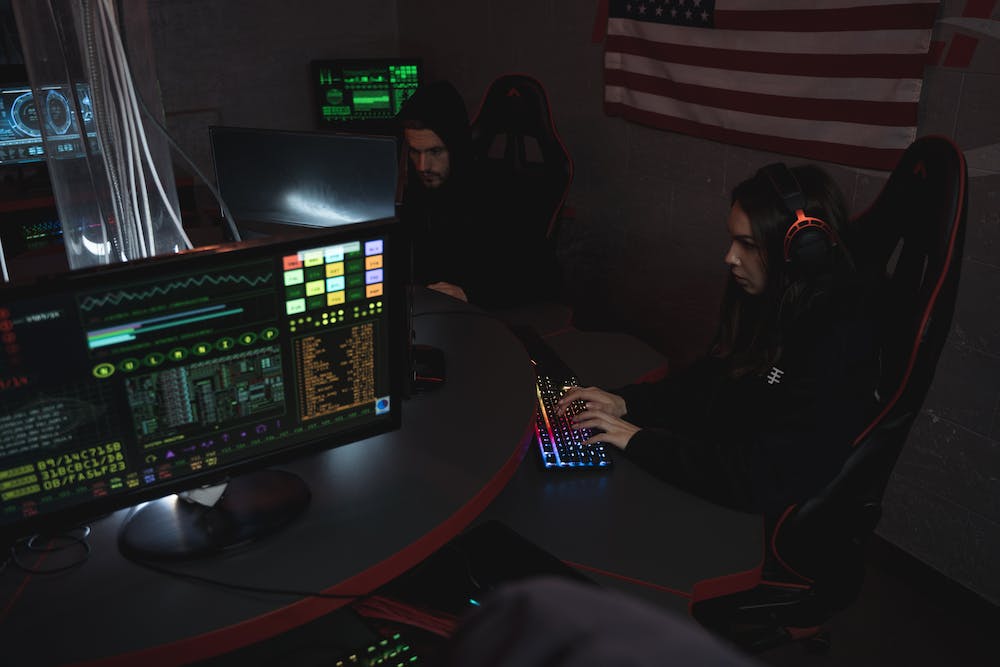
Mastering str_replace: A Comprehensive Guide
Introduction
In the world of programming, string manipulation is a common task that developers encounter on a regular basis. Whether you need to replace a specific word or character within a string, or you want to insert new content at a particular position, having a solid understanding of the str_replace
function can greatly simplify your coding process. This comprehensive guide will take you through everything you need to know about mastering str_replace
.
What is str_replace?
str_replace
is a useful PHP function that allows you to find and replace specific occurrences of a substring within a string. IT provides a straightforward way to manipulate strings by replacing target content with new content. The function takes three arguments: the target substring, the replacement substring, and the original string that needs to be modified.
Implementing str_replace
The syntax for using str_replace
is as follows:
str_replace(target, replacement, originalString)
Here is a simple example to illustrate how str_replace
works:
$originalString = "Hello, World!";
$target = "World";
$replacement = "Universe";
$newString = str_replace($target, $replacement, $originalString);
echo $newString; // Output: Hello, Universe!
Advanced Usage
While the basic functionality of str_replace
is relatively straightforward, there are a few advanced techniques that can be employed to enhance its usage.
Case Insensitivity: By default, str_replace
is case-sensitive. However, you can make IT case-insensitive by using the str_ireplace
function instead. This is particularly useful when you want to replace a substring regardless of its case.
Multiple Replacements: str_replace
can handle multiple replacements at once. You can pass arrays as arguments to make multiple replacements in one go, which is more efficient than calling str_replace
multiple times. Here’s an example:
$originalString = "The quick brown fox jumps over the lazy dog.";
$targets = array("quick", "brown", "fox");
$replacements = array("slow", "red", "cat");
$newString = str_replace($targets, $replacements, $originalString);
echo $newString; // Output: The slow red cat jumps over the lazy dog.
Conclusion
Mastering str_replace
can significantly streamline your string manipulation process in PHP. Whether you need to replace a single word or make multiple replacements, this powerful function provides you with the necessary tools to accomplish your tasks efficiently.
FAQs
Q: Can str_replace be used with arrays?
A: Yes, str_replace
can be used with arrays. By passing arrays as arguments, you can make multiple replacements simultaneously.
Q: Is str_replace case-sensitive?
A: By default, str_replace
is case-sensitive. However, if you need case-insensitivity, you can use the str_ireplace
function instead.
Q: What happens if the target substring is not present in the original string?
A: If the target substring is not found in the original string, str_replace
will simply return the original string without any modifications.
Q: Are there any alternatives to str_replace in PHP?
A: Yes, there are other string manipulation functions available in PHP, such as strtr
and preg_replace
, which provide different features and functionalities.