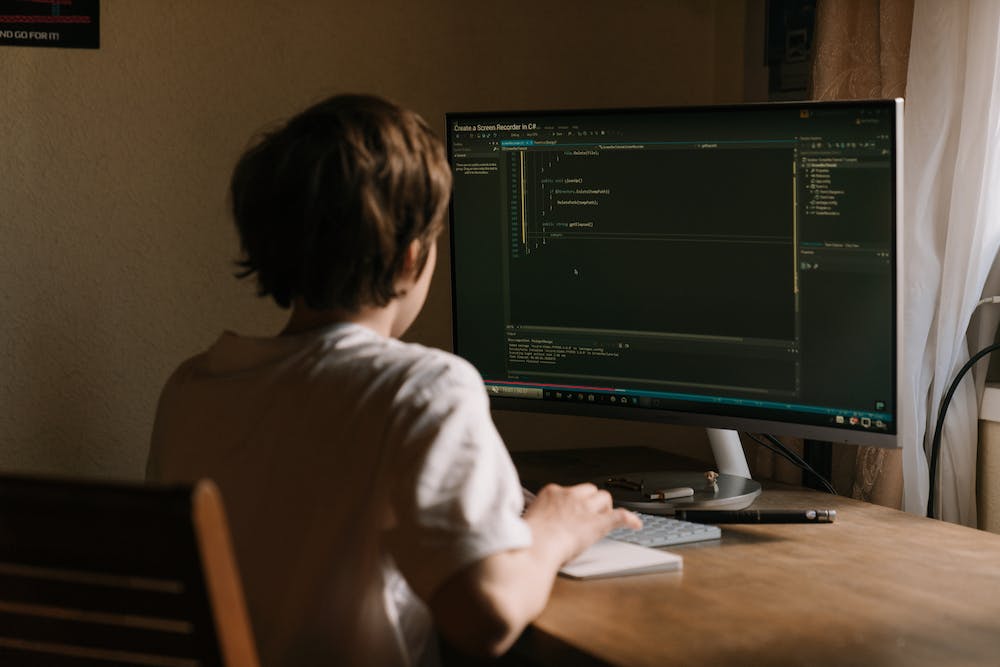
Python pseudocode is a powerful tool that allows developers to plan and visualize their code before actually writing IT in Python. By using pseudocode, developers can break down complex problems into smaller, more manageable pieces, making it easier to design and implement algorithms. In this article, we will explore five mind-blowing examples of Python pseudocode that can help you master this powerful tool.
Example 1: Finding the Maximum Number in a List
One of the most common tasks in programming is to find the maximum number in a list. With Python pseudocode, this task becomes much simpler. Here’s an example of how you can use Python pseudocode to find the maximum number in a list:
START
Set max_num to the first element in the list
For each number in the list
If the number is greater than max_num
Set max_num to the number
End For
Print max_num
END
By using this pseudocode, you can easily understand the logic behind finding the maximum number in a list before implementing it in Python. This can save you time and effort in writing and debugging your code.
Example 2: Calculating the Fibonacci Sequence
The Fibonacci sequence is a series of numbers in which each number is the sum of the two preceding ones, usually starting with 0 and 1. Using pseudocode, you can visualize the logic behind generating the Fibonacci sequence before writing the actual Python code. Here’s an example of Python pseudocode for calculating the Fibonacci sequence:
START
Set n1 to 0
Set n2 to 1
For i from 0 to n
Print n1
Set sum to n1 + n2
Set n1 to n2
Set n2 to sum
End For
END
By writing pseudocode for the Fibonacci sequence, you can clearly see the iterative logic involved in generating the sequence. This can be extremely helpful when you move on to writing the actual Python code.
Example 3: Checking for Prime Numbers
Another common programming task is checking whether a number is prime. You can use Python pseudocode to outline the logical steps for determining whether a number is prime before implementing it in Python. Here’s an example of Python pseudocode for checking prime numbers:
START
If the number is less than 2
Print “Not a prime number”
Else
Set is_prime to True
For i from 2 to the square root of the number
If the number is divisible by i
Set is_prime to False
End For
If is_prime is True
Print “Prime number”
Else
Print “Not a prime number”
End If
END
By using pseudocode, you can break down the logic for checking prime numbers into simple steps, making it easier to implement in Python. This approach can also help in identifying potential edge cases and optimizing the code for better performance.
Example 4: Sorting a List of Numbers
Sorting a list of numbers is a fundamental programming task, and Python pseudocode can help you understand the logic behind different sorting algorithms. Here’s an example of Python pseudocode for sorting a list of numbers using the bubble sort algorithm:
START
Set swapped to True
While swapped is True
Set swapped to False
For i from 0 to n-1
If the i-th element is greater than the i+1-th element
Swap the i-th and i+1-th elements
Set swapped to True
End For
End While
Print the sorted list
END
By using pseudocode, you can clearly see the iterative process involved in the bubble sort algorithm. This can be a great way to understand sorting algorithms before implementing them in Python.
Example 5: Implementing a Simple Binary Search
The binary search algorithm is an efficient way to search for a specific element in a sorted list. By using Python pseudocode, you can break down the logic behind the binary search algorithm before writing the actual Python code. Here’s an example of Python pseudocode for implementing a simple binary search:
START
Set low to 0
Set high to n-1
While low is less than or equal to high
Set mid to the middle index (low + high) // 2
If the mid element is equal to the search element
Print “Element found at index“
Else if the mid element is less than the search element
Set low to mid + 1
Else
Set high to mid - 1
End While
Print “Element not found”
END
By using pseudocode, you can visualize the process of dividing the search space in half at each step, making it easier to understand and implement the binary search algorithm in Python.
Conclusion
Python pseudocode is a valuable tool for planning, designing, and implementing algorithms in Python. By using pseudocode, you can break down complex problems into smaller, more manageable steps, making it easier to implement efficient and optimized code. The examples provided in this article demonstrate the power of Python pseudocode in various programming tasks, from finding the maximum number in a list to implementing a binary search algorithm. By mastering Python pseudocode, you can enhance your problem-solving skills and become a more efficient and effective programmer.
FAQs
What is Python pseudocode?
Python pseudocode is a way to plan and visualize the logic of a Python program before actually writing the code. It allows developers to break down complex problems into smaller, more manageable steps, making it easier to design and implement algorithms.
How can Python pseudocode help in programming?
Python pseudocode can help in programming by providing a clear and visual way to plan and understand the logic behind different algorithms and problem-solving techniques. It can save time and effort in writing and debugging code by outlining the steps and logic before implementing it in Python.
Is Python pseudocode essential for every Python programmer?
While Python pseudocode is not essential for every Python programmer, it can be a valuable tool for planning and visualizing the logic of complex algorithms and problems. It can enhance problem-solving skills and improve the efficiency and effectiveness of programming.
Where can I learn more about Python pseudocode?
You can learn more about Python pseudocode through online tutorials, programming forums, and books on algorithms and problem-solving. Additionally, you can practice writing pseudocode for different algorithms and problem-solving tasks to enhance your skills.
Is there any software that can help in writing Python pseudocode?
While there are no specific software tools designed for writing Python pseudocode, you can use any text editor or online pseudocode editor to write and visualize the logic of your Python programs. Some integrated development environments (IDEs) may also provide support for visualizing pseudocode.