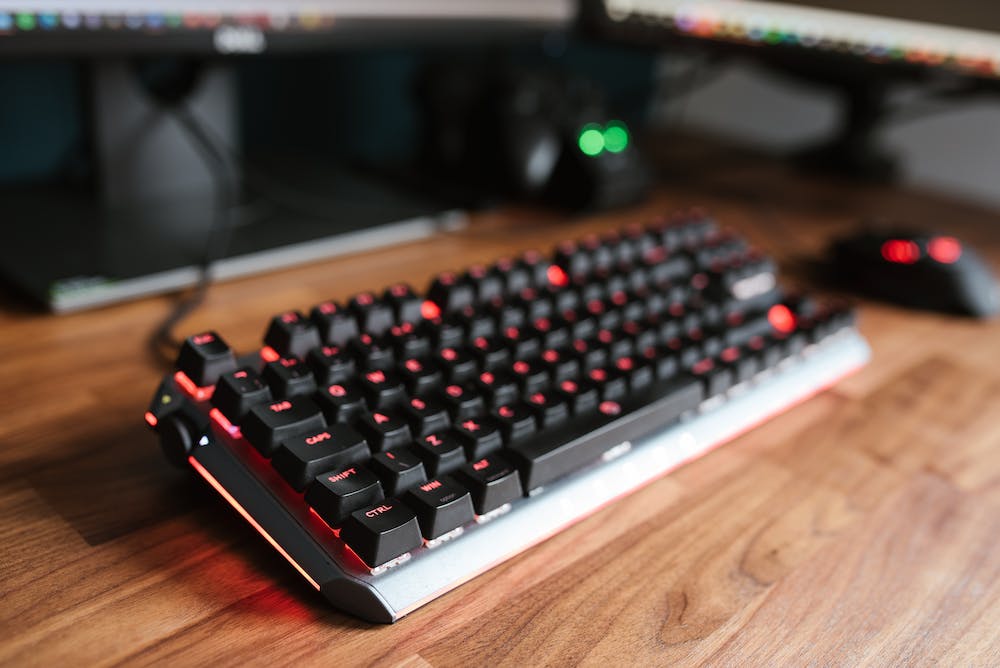
Python is a powerful and versatile programming language that is widely used for a variety of applications, including web development, data analysis, artificial intelligence, and more. If you have already mastered the basics of Python and want to take your skills to the next level, this article will provide you with advanced techniques and tips to help you become a proficient Python programmer.
Understanding Generators and Iterators
Generators and iterators are two powerful concepts in Python that can help you write more efficient and elegant code. Generators allow you to create iterators in a more simple and concise way, while iterators are objects that allow you to loop over sequences, such as lists or strings, without having to store the entire sequence in memory.
Here’s an example of using a generator to create an iterator:
def my_generator():
for i in range(5):
yield i
my_iterator = my_generator()
for item in my_iterator:
print(item)
In this example, the my_generator
function is used to create an iterator that yields each value in the range of 5. The yield
keyword is used to return a value from the generator, and the my_iterator
is then used to loop over the values yielded by the generator.
Working with Decorators and Context Managers
Decorators and context managers are two advanced features in Python that allow you to modify or enhance the behavior of functions and objects. Decorators are functions that wrap other functions to add functionality, while context managers are used to allocate and release resources automatically.
Here’s an example of using a decorator to log the execution time of a function:
import time
def log_execution_time(func):
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
print(f"{func.__name__} took {end_time - start_time} seconds to execute")
return result
return wrapper
@log_execution_time
def my_function():
# Some time-consuming operation
time.sleep(2)
my_function()
In this example, the log_execution_time
decorator is used to wrap the my_function
and log the execution time when the function is called.
Utilizing Multithreading and Multiprocessing
Python provides support for both multithreading and multiprocessing, which allow you to run multiple tasks concurrently to improve the performance of your applications. Multithreading is suitable for I/O-bound tasks, such as network operations, while multiprocessing is suitable for CPU-bound tasks, such as number crunching.
Here’s an example of using threading to fetch data from multiple URLs concurrently:
import threading
import requests
def fetch_url(url):
response = requests.get(url)
print(f"Fetched {url}")
urls = ['http://example.com', 'http://example.org', 'http://example.net']
threads = []
for url in urls:
t = threading.Thread(target=fetch_url, args=(url,))
threads.append(t)
t.start()
for t in threads:
t.join()
In this example, multiple threads are created to fetch data from the specified URLs concurrently, improving the overall performance of the task.
Conclusion
By mastering these advanced techniques and tips, you can become a proficient Python programmer capable of building complex and efficient applications. Understanding generators and iterators, working with decorators and context managers, and utilizing multithreading and multiprocessing will enhance your ability to write elegant and high-performing Python code.
FAQs
Q: What are some advanced data structures in Python?
A: Python provides several advanced data structures, such as sets, dictionaries, and tuples, which offer unique features and advantages for storing and manipulating data.
Q: How can I improve the performance of my Python applications?
A: You can improve the performance of your Python applications by implementing advanced techniques such as multithreading, multiprocessing, and optimizing the use of generators and iterators.
Q: Can decorators and context managers be used together in Python?
A: Yes, decorators and context managers can be used together to enhance the behavior of functions and objects and manage resources more effectively.