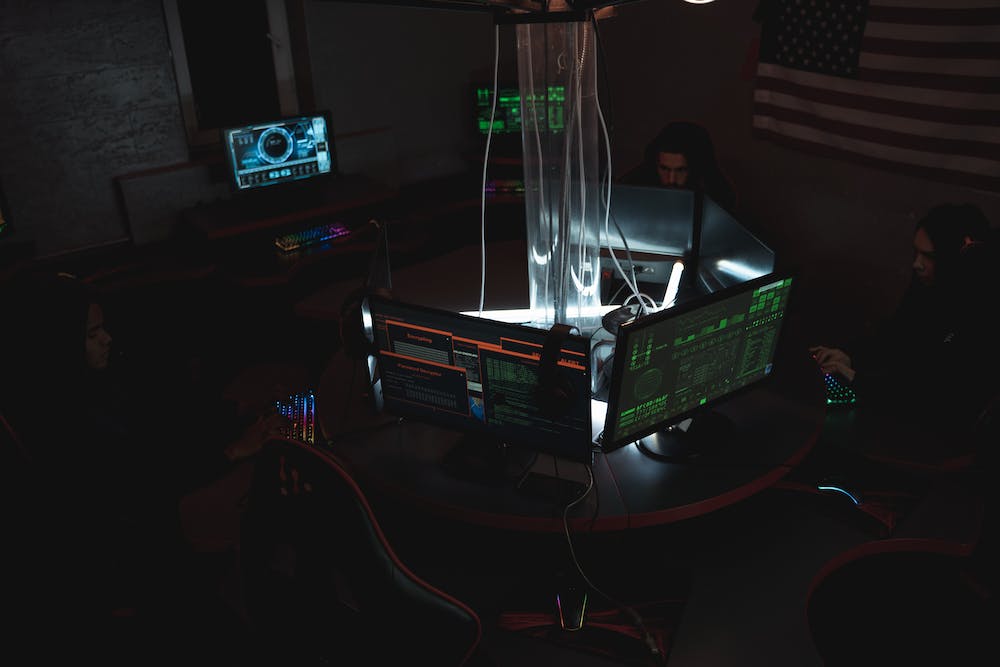
In recent years, Python has become one of the most popular programming languages in the world. Its simplicity, readability, and versatility make IT an ideal choice for beginners and experienced developers alike. Whether you’re looking to build web applications, analyze data, or automate tasks, mastering Python can open up a world of possibilities. In this comprehensive guide, we’ll cover everything you need to know to learn Python from scratch and become proficient in the language.
Getting Started with Python
If you’re completely new to programming, Python is a great language to start with. Its clean and readable syntax makes it easy to understand, and there are plenty of resources available online to help you get started. To begin your journey with Python, you’ll need to install the Python interpreter on your computer. There are different versions of Python available, but for the purposes of this guide, we’ll focus on Python 3, which is the latest stable release of the language.
Setting up Python on Your Computer
To install Python 3 on your computer, you can download the installer from the official Python Website (python.org). Once you’ve downloaded the installer, simply run it and follow the on-screen instructions to complete the installation process.
Learning the Basics
Once Python is installed on your computer, you can start writing and running Python code using a text editor or an integrated development environment (IDE). Python comes with a built-in IDLE (Integrated Development and Learning Environment) that you can use to write and execute Python code. Alternatively, you can use popular text editors like Sublime Text, Visual Studio Code, or Atom, which have plugins to support Python development.
Before diving into more complex topics, it’s important to get a solid understanding of the basics of Python. This includes learning about variables, data types, control structures, and functions. Once you have a good grasp of these fundamental concepts, you’ll be well-equipped to tackle more advanced topics in Python.
Working with Variables and Data Types
In Python, variables are used to store data that can be manipulated and referenced in your programs. Variables can hold different types of data, such as numbers, strings, lists, and dictionaries. In Python, you don’t need to declare the data type of a variable explicitly; Python infers it based on the value assigned to the variable.
Here’s an example of how to declare and use variables in Python:
# Declare and initialize a variable
age = 25
# Print the value of the variable
print(age)
Control Structures and Functions
Control structures, such as if-else statements and loops, are used to control the flow of execution in a Python program. Functions, on the other hand, are used to encapsulate reusable pieces of code that perform a specific task. Understanding how to use control structures and functions is crucial for writing effective and maintainable Python code.
Here’s an example of a simple function in Python:
# Define a function
def greet(name):
print("Hello, " + name + "!")
# Call the function
greet("John")
Working with Data
Python provides powerful tools for working with data, including built-in data structures and libraries for data manipulation and analysis. Whether you’re dealing with structured data, unstructured data, or time series data, Python has you covered.
One of the most popular libraries for data manipulation and analysis in Python is Pandas. With Pandas, you can easily load, clean, transform, and analyze data from a variety of sources, such as CSV files, Excel spreadsheets, or databases. If you’re interested in data visualization, you can use libraries like Matplotlib and Seaborn to create stunning visualizations of your data.
Working with Pandas
Here’s a simple example of how to use Pandas to load and display a CSV file:
# Import the Pandas library
import pandas as pd
# Load the CSV file into a DataFrame
data = pd.read_csv('data.csv')
# Display the first few rows of the DataFrame
print(data.head())
Building Web Applications with Python
Python is also widely used for web development, thanks to popular web frameworks like Django and Flask. These frameworks provide a solid foundation for building web applications quickly and efficiently. Whether you’re building a simple blog or a complex e-commerce platform, Python has the tools and libraries you need to get the job done.
Getting Started with Flask
Flask is a lightweight and easy-to-use web framework for Python. With Flask, you can quickly create web applications and APIs with minimal boilerplate code. Here’s a simple example of how to create a basic web application with Flask:
from flask import Flask
# Create a new Flask app
app = Flask(__name__)
# Define a route and a view function
@app.route('/')
def index():
return 'Hello, World!'
# Run the app
if __name__ == '__main__':
app.run()
Automating Tasks with Python
Python’s simplicity and versatility make it an ideal language for automating repetitive tasks. Whether you’re looking to automate file processing, web scraping, or system administration, Python has a rich ecosystem of libraries and tools to help you get the job done.
Automating File Processing
Python’s standard library provides modules for working with files and directories, making it easy to automate tasks like renaming files, moving files, or manipulating file contents.
import os
# List all files in a directory
files = os.listdir('path/to/directory')
# Rename all files with a specific extension
for file in files:
if file.endswith('.jpg'):
os.rename(file, file.replace('.jpg', '_new.jpg'))
Conclusion
Mastering Python opens up a world of possibilities, whether you’re looking to build web applications, analyze data, or automate tasks. In this comprehensive guide, we’ve covered the basics of Python, working with data, building web applications, and automating tasks. With the knowledge and skills gained from this guide, you’ll be well on your way to becoming proficient in Python and leveraging its power to solve real-world problems.
FAQs
Q: Is Python difficult to learn for beginners?
A: Python is considered one of the most beginner-friendly programming languages due to its simple and readable syntax. With the right resources and practice, beginners can quickly grasp the fundamentals of Python.
Q: What are the career opportunities for Python developers?
A: Python developers are in high demand across various industries, including web development, data analysis, machine learning, and automation. With the increasing popularity of Python, there are ample career opportunities for skilled Python developers.
Q: How can I continue learning and improving my Python skills?
A: There are many resources available for continued learning, including online courses, tutorials, and open-source projects. Additionally, participating in Python communities and attending meetups can provide valuable networking and learning opportunities for Python enthusiasts.
References
- “Python Tutorial” – Python.org
- “Pandas Documentation” – pandas.pydata.org
- “Flask Documentation” – flask.pocoo.org