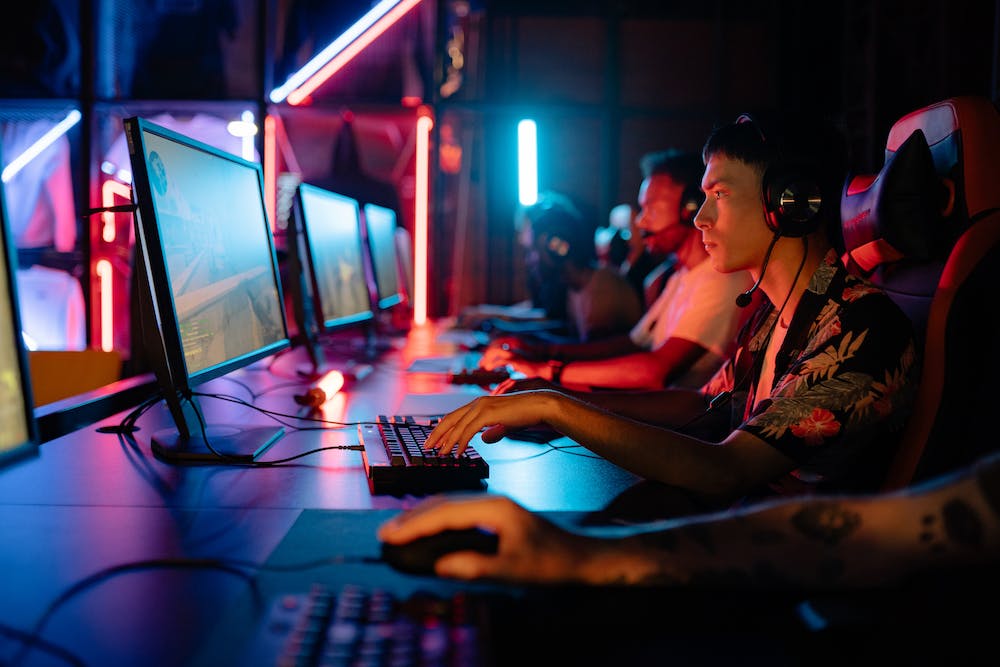
When IT comes to interacting with servers and APIs, making POST requests is a crucial skill for any PHP developer. While there are several ways to make POST requests in PHP, one of the most powerful and popular methods is using cURL. In this tutorial, we will guide you through the process of mastering POST requests in PHP with cURL, step by step.
Understanding POST Requests
Before diving into the details of making POST requests with cURL in PHP, it’s important to have a clear understanding of what POST requests are. In the context of web development, POST requests are used to send data to a server to create or update a resource. This data is sent in the body of the HTTP request, as opposed to GET requests which send data in the URL.
POST requests are commonly used when submitting forms, uploading files, and interacting with APIs. When working with APIs, POST requests are often used to create new resources, update existing ones, or perform other actions that modify data on the server.
Using cURL for Making POST Requests in PHP
cURL is a powerful library that allows you to make HTTP requests from PHP, including POST requests. It provides a wide range of features and options for customizing your requests, making it a popular choice for interacting with APIs and web services.
To use cURL for making POST requests in PHP, you first need to initialize a cURL session using the curl_init()
function. Then, you can set various options for the request, such as the URL, request method, headers, and request body. Finally, you execute the request using the curl_exec()
function and handle the response as needed.
Throughout this tutorial, we will walk you through the process of making a simple POST request using cURL in PHP, and then gradually delve into more advanced topics such as handling response headers, error handling, and best practices for working with cURL.
Step 1: Initializing a cURL Session
The first step in making a POST request with cURL in PHP is to initialize a cURL session using the curl_init()
function. This function returns a cURL handle, which is used for setting options and executing the request.
Here’s an example of how to initialize a cURL session for making a POST request:
<?php
// Initialize cURL session
$ch = curl_init();
?>
In this example, we have assigned the cURL handle returned by curl_init()
to the variable $ch
. We will use this handle to set options and execute the request in the subsequent steps.
Step 2: Setting Request Options
Once you have initialized a cURL session, the next step is to set the options for the POST request. This includes setting the request URL, request method, request headers, and request body.
Here’s an example of how to set the options for a basic POST request:
<?php
// Set request URL
curl_setopt($ch, CURLOPT_URL, 'https://api.example.com/resource');
// Set request method to POST
curl_setopt($ch, CURLOPT_POST, true);
// Set request body
$data = array(
'key1' => 'value1',
'key2' => 'value2'
);
curl_setopt($ch, CURLOPT_POSTFIELDS, http_build_query($data));
?>
In this example, we have set the request URL using curl_setopt()
with the CURLOPT_URL
option. We have also set the request method to POST using the CURLOPT_POST
option, and specified the request body using the CURLOPT_POSTFIELDS
option.
It’s important to note that the request body is formatted as a query string using the http_build_query()
function. Depending on the requirements of the server or API you are interacting with, you may need to format the request body differently, such as JSON or XML.
Step 3: Executing the Request
After setting the request options, the next step is to execute the POST request using the curl_exec()
function. This function will send the request to the server and return the response, which you can then handle as needed.
Here’s an example of how to execute a POST request using cURL in PHP:
<?php
// Execute the request and get the response
$response = curl_exec($ch);
// Close cURL session
curl_close($ch);
// Handle the response
if($response === false) {
echo 'cURL error: ' . curl_error($ch);
} else {
echo 'Response: ' . $response;
}
?>
In this example, we have used curl_exec()
to execute the POST request and store the response in the $response
variable. We then used curl_close()
to close the cURL session, and finally handled the response by checking for errors and outputting the response content.
Advanced Topics in Making POST Requests with cURL in PHP
While the above steps cover the basics of making a POST request with cURL in PHP, there are several advanced topics and best practices to consider when working with cURL. Some of these topics include handling response headers, error handling, and optimizing your cURL requests for performance.
Handling Response Headers
When making POST requests with cURL, it’s important to be able to access and handle the response headers as well as the response body. Response headers may contain important information such as the content type, content length, and other metadata about the response.
You can retrieve the response headers using the CURLOPT_HEADER
option in curl_setopt()
, and then parse and handle the headers as needed.
Error Handling
When making HTTP requests with cURL, it’s crucial to have robust error handling in place to handle various error scenarios. This includes checking for errors returned by cURL functions, handling HTTP status codes, and implementing retry mechanisms for transient errors.
By properly handling errors, you can ensure that your application is resilient and can gracefully handle unexpected issues when making POST requests with cURL.
Optimizing cURL Requests
To optimize your cURL requests for performance and reliability, there are several best practices to consider. These include reusing cURL handles, setting timeouts and connection limits, and using persistent connections to keep the connection alive for multiple requests.
By following these best practices, you can ensure that your cURL requests are efficient, reliable, and scalable, making them suitable for production use in high-traffic applications.
Conclusion
In conclusion, mastering POST requests in PHP with cURL is a valuable skill for any web developer. With the ability to send data to servers, interact with APIs, and perform a wide range of actions, POST requests are a fundamental aspect of modern web development.
By following the step-by-step tutorial in this article and delving into advanced topics such as handling response headers, error handling, and optimizing cURL requests, you can become proficient in making POST requests with cURL in PHP. This will empower you to build robust and scalable applications that effectively interact with external services and APIs.
FAQs
Q: What is cURL?
A: cURL is a library and command-line tool for transferring data with URLs. In the context of PHP, cURL is commonly used for making HTTP requests to interact with web services and APIs.
Q: Can I make POST requests with cURL in PHP?
A: Yes, you can make POST requests with cURL in PHP using the curl_setopt()
function to set the request method to POST, and the curl_setopt()
function to specify the request body.
Q: What are some best practices for making cURL requests in PHP?
A: Some best practices for making cURL requests in PHP include reusing cURL handles, setting timeouts and connection limits, and using persistent connections to keep the connection alive for multiple requests.