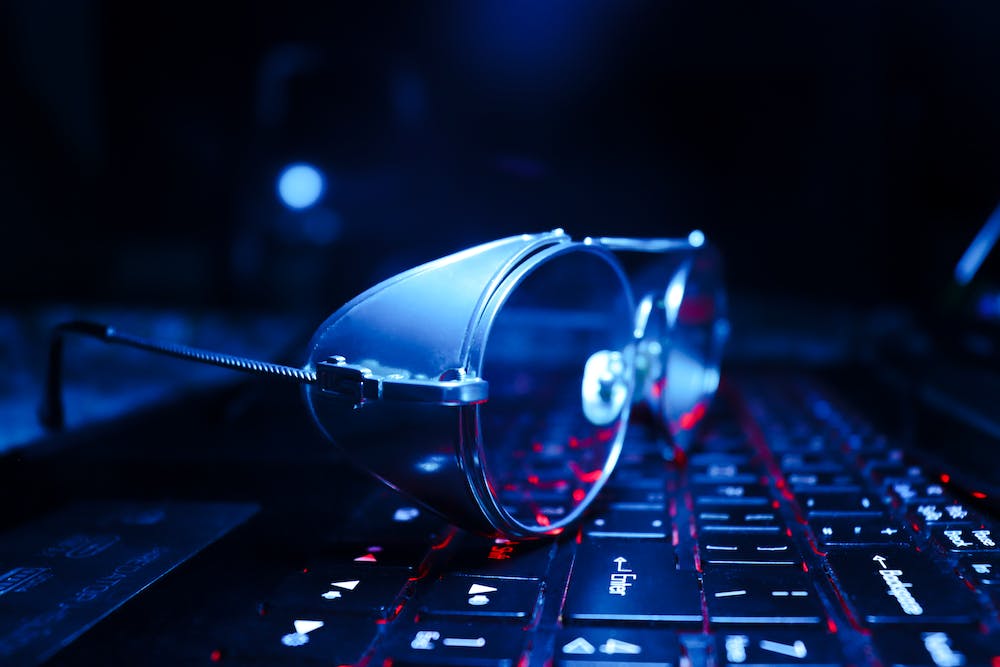
PHP is a popular scripting language used for web development. One of the most powerful functions in PHP is ‘preg_match’, which is used for string matching using regular expressions. In this article, we will explore advanced techniques for using ‘preg_match’ to its full potential.
Understanding Regular Expressions
Before diving into advanced techniques for using ‘preg_match’, IT‘s important to have a solid understanding of regular expressions. Regular expressions are patterns used to match character combinations in strings. They are incredibly flexible and can be used to perform complex string matching operations.
For example, the regular expression pattern /[0-9]+/
will match one or more digits in a string. Similarly, the pattern /[a-z]+/i
will match one or more letters in a case-insensitive manner.
Basic Usage of ‘preg_match’
The basic usage of ‘preg_match’ involves providing a regular expression pattern and a string to search within. The function returns a boolean value indicating whether a match was found.
“`php
$string = “The quick brown fox jumps over the lazy dog”;
if (preg_match(“/fox/”, $string)) {
echo “Match found!”;
} else {
echo “No match found.”;
}
?>
“`
In the above example, the regular expression /fox/
is used to search for the word “fox” in the given string. Since the word “fox” is present in the string, the function will return true and “Match found!” will be echoed.
Advanced Techniques
Using Capture Groups
Capture groups are a powerful feature of regular expressions that allow you to extract specific parts of a matched string. This is incredibly useful when you need to extract data from a larger string, such as parsing a URL or extracting values from a CSV file.
You can define capture groups using parentheses in the regular expression pattern. For example, the pattern /(\dhigh quality backlinks)-(\dhigh quality backlinks)-(\dfreelance writing)/
will match a date in the format dd-mm-yyyy and capture the day, month, and year as separate groups.
“`php
$date = “Today’s date is 23-10-2022”;
if (preg_match(“/(\dhigh quality backlinks)-(\dhigh quality backlinks)-(\dfreelance writing)/”, $date, $matches)) {
echo “Day: ” . $matches[1] . “, Month: ” . $matches[2] . “, Year: ” . $matches[3];
} else {
echo “No match found.”;
}
?>
“`
In the above example, the ‘preg_match’ function will capture the day, month, and year from the given date string and store them in the $matches array. The echoed output will be “Day: 23, Month: 10, Year: 2022”.
Using Lookahead and Lookbehind Assertions
Lookahead and lookbehind assertions are advanced concepts in regular expressions that allow you to define conditions for a match without including the matched text in the result. Lookahead assertions are defined using (?=...)
and lookbehind assertions are defined using (?<=...)
.
For example, the pattern /\b\w+(?=\sis\b)/
will match words that are followed by the word "is" without including "is" in the match.
```php
$string = "This is a test string";
if (preg_match("/\b\w+(?=\sis\b)/", $string, $matches)) {
echo "Match found: " . $matches[0];
} else {
echo "No match found.";
}
?>
```
In the above example, the 'preg_match' function will match the word "test" from the given string without including "is" in the result.
Conclusion
The 'preg_match' function in PHP is a powerful tool for string matching using regular expressions. By understanding and mastering advanced techniques such as capture groups and lookahead/lookbehind assertions, you can take full advantage of the flexibility and power of regular expressions.
FAQs
What are the advantages of using 'preg_match' over simple string functions?
'preg_match' allows for complex pattern matching using regular expressions, which provides much greater flexibility and power compared to simple string functions such as 'strpos' or 'strstr'.
Can 'preg_match' be used for parsing HTML or XML documents?
While it is possible to use 'preg_match' for parsing HTML or XML, it is generally not recommended due to the complexity and potential pitfalls of parsing structured documents with regular expressions. It is better to use dedicated parsing libraries for these tasks.
Is 'preg_match' case-sensitive by default?
Yes, 'preg_match' is case-sensitive by default. However, you can use the 'i' modifier in the regular expression pattern to make the matching case-insensitive.