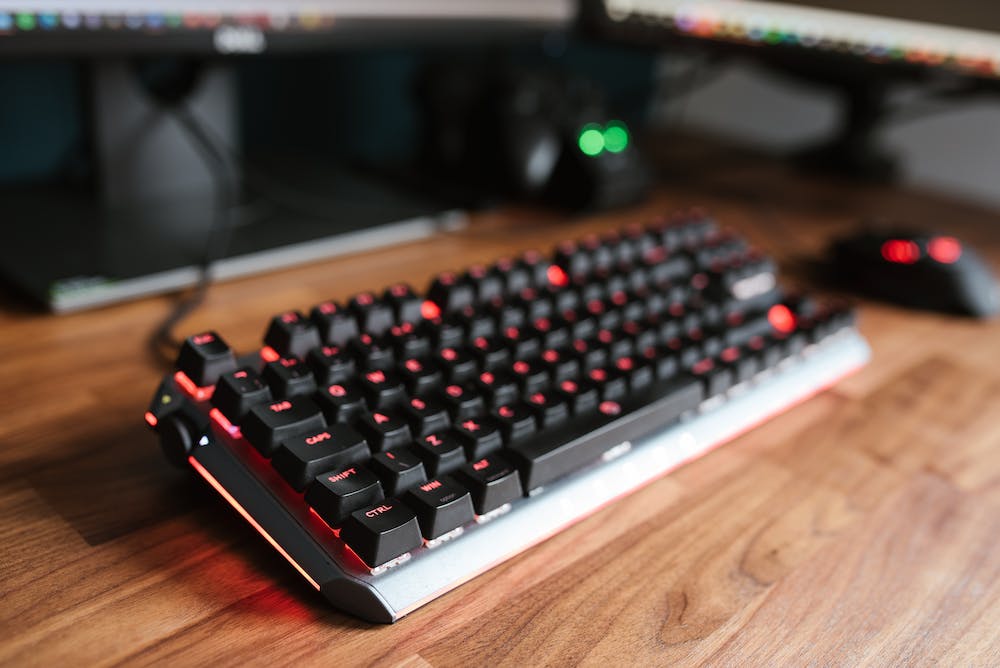
PHP (Hypertext Preprocessor) is a widely-used open source general-purpose scripting language that is especially suited for web development and can be embedded into HTML. IT is a powerful tool for creating dynamic and interactive websites. Mastering PHP syntax is essential for writing efficient and clean code. In this article, we will explore some tips and tricks for mastering PHP syntax to improve your coding skills.
1. Use Proper Indentation and Formatting
Proper indentation and formatting are essential for writing clean and readable PHP code. Indentation helps to visually organize the code and makes it easier to understand the structure of the program. Use consistent indentation, such as tab or space, to improve the readability of your code.
Additionally, using proper formatting such as line breaks and consistent spacing can make your code more aesthetically pleasing and easier to navigate. This not only makes the code easier to understand for yourself and other developers, but also helps in debugging and maintaining the code in the long run.
2. Follow Naming Conventions
Following naming conventions for variables, functions, classes, and other identifiers is crucial for writing clean and maintainable code. Use descriptive and meaningful names for your variables and functions to make the code self-explanatory.
For example, instead of using a variable name like “$a” or “$x”, use names that convey the purpose of the variable, such as “$username” or “$totalAmount”. Following a consistent naming convention, such as camelCase or snake_case, can also help in making the code more consistent and readable.
3. Understand Operators and Expressions
Understanding and mastering PHP operators and expressions is essential for efficient coding. PHP supports a wide range of operators, such as arithmetic, assignment, comparison, logical, and bitwise operators. Understanding how these operators work and their precedence can help in writing concise and optimized code.
Additionally, understanding expressions and how they evaluate can help in avoiding common pitfalls and writing more efficient code. Use parentheses to clarify the order of operations and improve readability.
4. Use Control Structures Effectively
PHP provides a variety of control structures, such as if statements, loops, and switch statements, for controlling the flow of the program. Mastering these control structures and using them effectively can make your code more logical and concise.
Use if-else statements to make decisions based on conditions, and loops such as for, foreach, and while to iterate over arrays and other data structures. Additionally, switch statements can be used to simplify complex conditional logic.
5. Sanitize User Input and Use Prepared Statements
When working with user input, it is important to sanitize the input to prevent security vulnerabilities such as SQL injection and cross-site scripting (XSS) attacks. Use functions such as mysqli_real_escape_string()
or prepared statements in PDO to sanitize input and prevent SQL injection.
6. Optimize Loops and Use Built-in Functions
When working with loops and arrays, it is important to optimize your code for performance. Use efficient loop constructs and built-in array functions such as foreach
, array_map
, array_filter
, and array_reduce
to manipulate arrays and iterate over them efficiently.
7. Error Handling and Debugging
Proper error handling and debugging are essential for writing reliable and robust PHP code. Use error reporting functions such as error_reporting()
and ini_set('display_errors', 1)
to display errors and warnings during development.
Additionally, use debugging tools such as var_dump()
and print_r()
to inspect variables and data structures for debugging purposes. Tools like Xdebug can also be used for more advanced debugging and profiling.
8. Use Classes and Object-Oriented Programming
PHP supports object-oriented programming (OOP) and using classes and objects can help in organizing and modularizing your code. Use classes to encapsulate data and behavior, and leverage features such as inheritance, polymorphism, and encapsulation to write more maintainable and reusable code.
9. Write Reusable and Modular Code
Writing reusable and modular code is essential for efficient PHP programming. Break down your code into manageable and reusable functions and classes. Consider using design patterns like MVC (Model-View-Controller) to organize your code and separate concerns.
10. Performance Optimization
Lastly, consider performance optimization techniques such as caching, lazy loading, and minimizing database queries to improve the overall performance of your PHP applications.
Conclusion
Mastering PHP syntax is essential for writing efficient and clean code. By following the tips and tricks mentioned in this article, you can improve your coding skills and write more maintainable and optimized PHP applications.
FAQs
Q: What are some common pitfalls to avoid in PHP coding?
A: Some common pitfalls to avoid in PHP coding include not sanitizing user input, not validating and escaping data, and not handling errors and exceptions properly.
Q: How can I improve the performance of my PHP applications?
A: To improve the performance of your PHP applications, consider techniques such as caching, minimizing database queries, and using efficient algorithms and data structures.
Q: Is it necessary to use object-oriented programming in PHP?
A: Object-oriented programming is not necessary in PHP, but it can help in organizing and structuring your code, especially for larger and more complex projects.
Q: What are some popular PHP frameworks and libraries?
A: Some popular PHP frameworks and libraries include Laravel, Symfony, CodeIgniter, and Zend Framework.