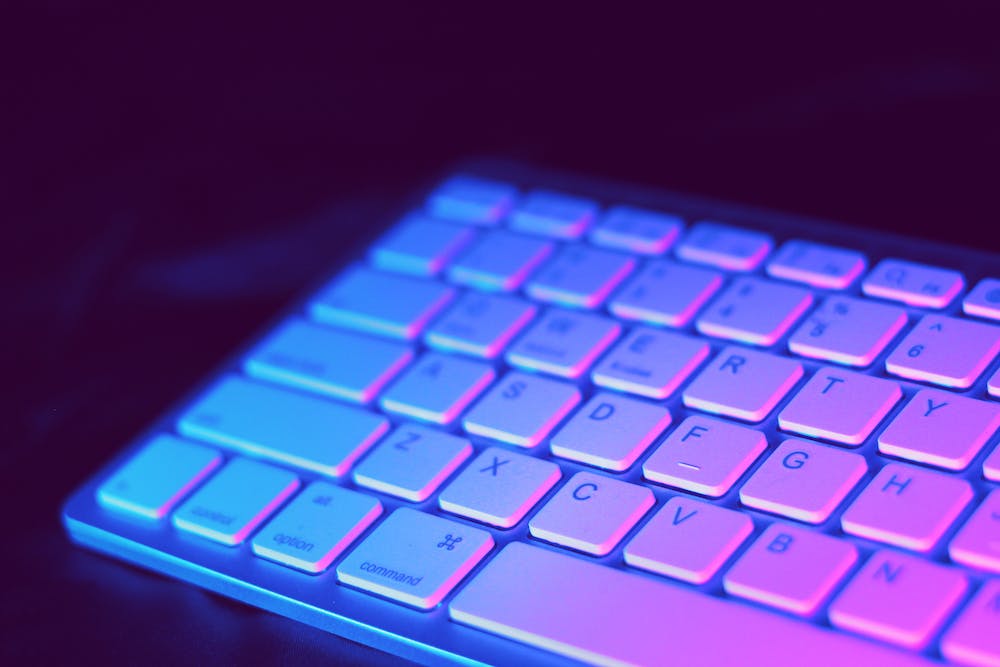
PHP arrays are a powerful tool for storing and manipulating data. Whether you are a beginner or an experienced developer, mastering array manipulation is essential for efficient and effective PHP programming. In this article, we will explore some essential tips and tricks to help you become fluent in PHP array manipulation. We will cover a range of topics, including creating arrays, accessing array elements, adding and removing elements, sorting arrays, and more.
Creating Arrays
In PHP, arrays can be created in several ways. The most common method is using the array() function. For example:
$fruits = array("apple", "banana", "cherry");
You can also create an array by specifying key-value pairs:
$person = array("name" => "John", "age" => 30, "city" => "New York");
Another way to create an array is by using the short array syntax introduced in PHP 5.4:
$colors = ["red", "green", "blue"];
Accessing Array Elements
To access individual elements of an array, you can use square brackets. For example:
$fruits = array("apple", "banana", "cherry");
echo $fruits[0]; // Output: apple
echo $fruits[2]; // Output: cherry
If you have an array of key-value pairs, you can access the values using the corresponding keys:
$person = array("name" => "John", "age" => 30, "city" => "New York");
echo $person["name"]; // Output: John
echo $person["age"]; // Output: 30
Adding and Removing Elements
To add elements to an array, you can use the square bracket notation. For example, to add a new fruit to the $fruits array:
$fruits[] = "orange";
This will append the element “orange” to the end of the array. If you want to specify the index, you can do so:
$fruits[4] = "strawberry";
To remove elements from an array, you can use the unset() function. For example, to remove the second fruit (banana) from the $fruits array:
$fruits = array("apple", "banana", "cherry");
unset($fruits[1]);
This will remove the element at index 1 and reindex the remaining elements.
Sorting Arrays
PHP offers several built-in functions for sorting arrays. The sort() function is used to sort an array in ascending order:
$numbers = array(5, 3, 8, 1);
sort($numbers);
print_r($numbers);
// Output: Array ( [0] => 1 [1] => 3 [2] => 5 [3] => 8 )
If you want to sort the array in descending order, you can use the rsort() function:
$numbers = array(5, 3, 8, 1);
rsort($numbers);
print_r($numbers);
// Output: Array ( [0] => 8 [1] => 5 [2] => 3 [3] => 1 )
If you have an array of key-value pairs and you want to sort the array based on the values, you can use the asort() function:
$ages = array("John" => 30, "Jane" => 25, "Mark" => 35);
asort($ages);
print_r($ages);
// Output: Array ( [Jane] => 25 [John] => 30 [Mark] => 35 )
Multidimensional Arrays
In PHP, you can also create multidimensional arrays, which are arrays within arrays. This can be useful for representing hierarchical data or organizing complex data structures. For example:
$products = array(
array("name" => "iPhone", "price" => 999),
array("name" => "iPad", "price" => 799),
array("name" => "MacBook", "price" => 1299)
);
echo $products[1]["name"]; // Output: iPad
FAQs
Q: Can arrays have mixed data types in PHP?
Yes, PHP arrays can have mixed data types. You can store integers, strings, floats, booleans, or even other arrays within an array.
Q: How do I check if an element exists in an array?
You can use the in_array() function to check if a value exists in an array. IT returns true if the value is found and false otherwise.
$fruits = array("apple", "banana", "cherry");
if (in_array("banana", $fruits)) {
echo "Found";
} else {
echo "Not found";
}
This code will output “Found” since “banana” exists in the $fruits array.
Q: Can I sort an array based on its keys?
Yes, you can use the ksort() function to sort an array based on its keys in ascending order, or krsort() to sort in descending order.
$ages = array("John" => 30, "Jane" => 25, "Mark" => 35);
ksort($ages);
print_r($ages);
// Output: Array ( [Jane] => 25 [John] => 30 [Mark] => 35 )
With these essential tips and tricks, you can level up your PHP array manipulation skills. Arrays are an indispensable tool for managing and manipulating data in PHP, and mastering their manipulation will make your code more efficient and effective. So, practice these techniques and explore more advanced array manipulation functions to become a PHP array pro!