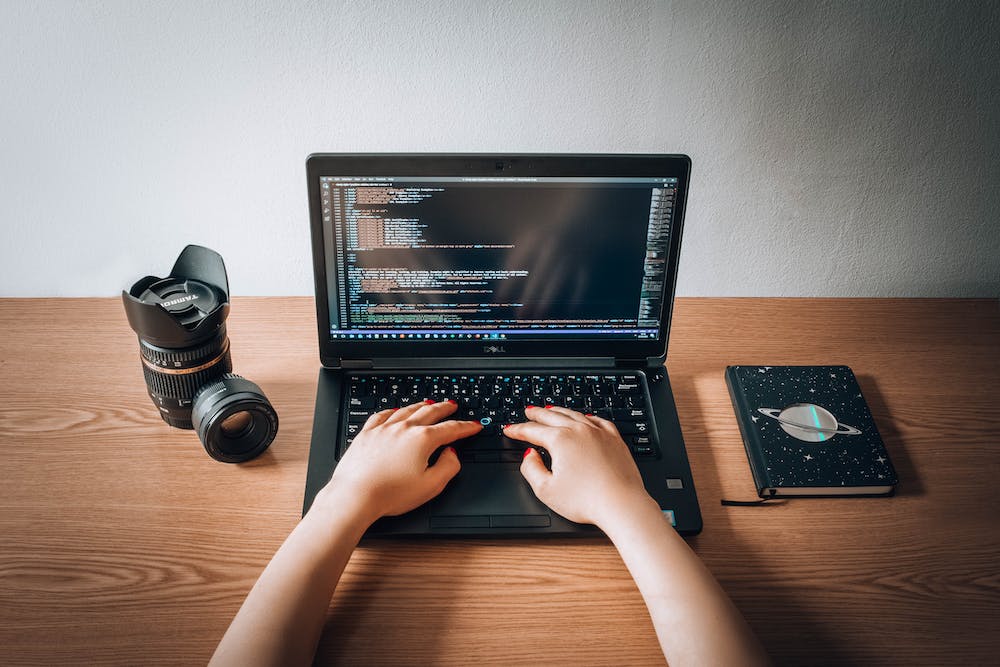
PHP (Hypertext Preprocessor) is a widely-used open source general-purpose scripting language that is especially suited for web development and can be embedded into HTML. Understanding advanced techniques and best practices in PHP can significantly enhance the efficiency and effectiveness of your web development projects. In this article, we will explore advanced PHP techniques and best practices that will help you become a master of PHP programming.
1. Object-Oriented Programming (OOP)
One of the most important advanced techniques in PHP is Object-Oriented Programming (OOP). OOP allows you to create reusable and modular code by organizing your code into classes and objects. This approach makes IT easier to manage and maintain complex projects. When using OOP in PHP, it is essential to understand concepts such as inheritance, encapsulation, and polymorphism. By mastering OOP, you can write more efficient and scalable code.
2. Namespacing
Namespacing is a feature in PHP that allows you to organize your code into logical and hierarchical structures. Namespaces prevent naming conflicts between different parts of your code and improve code readability. By utilizing namespaces effectively, you can develop more organized and manageable codebases. This is especially useful when working on large projects with multiple developers.
3. Error Handling
Effective error handling is crucial in PHP development. By mastering advanced error handling techniques, such as custom error handling, error reporting, and exception handling, you can ensure that your applications are robust and dependable. Proper error handling also enhances the security of your applications by preventing the exposure of sensitive information to potential attackers.
4. Performance Optimization
PHP offers various techniques for optimizing the performance of your applications. From caching mechanisms to database query optimization, mastering performance optimization techniques can significantly improve the speed and efficiency of your code. Advanced topics such as opcode caching, query optimization, and code profiling can help you fine-tune the performance of your PHP applications.
5. Security Best Practices
Security is a critical aspect of PHP development. By mastering security best practices, such as input validation, data sanitization, and secure coding practices, you can protect your applications from common vulnerabilities such as SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF). Understanding PHP security best practices is essential for developing secure and reliable applications.
6. Testing and Debugging
Testing and debugging are essential parts of the software development process. By mastering advanced testing and debugging techniques, such as unit testing, integration testing, and debugging tools, you can ensure the quality and reliability of your PHP applications. Effective testing and debugging practices help identify and fix issues early in the development process, saving time and effort in the long run.
7. Back-end Frameworks
Mastering back-end PHP frameworks, such as Laravel, Symfony, and CodeIgniter, can significantly enhance your development capabilities. These frameworks provide a range of advanced features and tools for building robust and scalable applications. Understanding and utilizing back-end frameworks effectively can streamline your development process and improve the overall quality of your applications.
Conclusion
Mastering advanced PHP techniques and best practices is essential for becoming a proficient and efficient PHP developer. By understanding and implementing object-oriented programming, namespacing, error handling, performance optimization, security best practices, testing and debugging, and back-end frameworks, you can elevate your PHP development skills to the next level. Continuous learning and practice are key to mastering PHP and staying ahead in the dynamic world of web development.
FAQs
Q: Can you provide an example of using namespaces in PHP?
A: Sure! Here’s an example of defining and using namespaces in PHP:
<?php
namespace MyNamespace;
class MyClass {
// Class code here
}
// Using the class from the namespace
$obj = new MyNamespace\MyClass();
?>
Q: How can I optimize the performance of my PHP applications?
A: There are several ways to optimize the performance of PHP applications, such as using caching mechanisms, optimizing database queries, enabling opcode caching, and utilizing code profiling tools. It’s important to analyze the specific performance bottlenecks in your application and apply targeted optimization techniques.