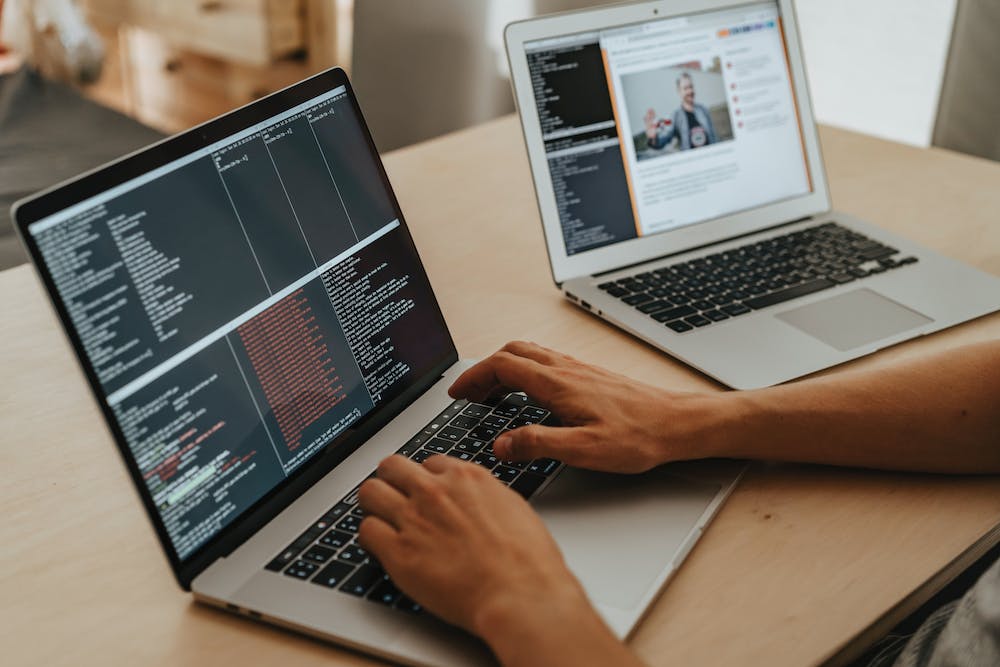
Python is a versatile and powerful programming language that is widely used for a variety of applications, including web development, data analysis, artificial intelligence, and more. With its clean and readable syntax, Python has gained popularity among developers of all skill levels. However, as with any language, IT‘s important to follow best practices to ensure that your code is maintainable, scalable, and easily understandable by others. One of the most widely recognized standards for writing clean Python code is PEP8.
What is PEP8?
PEP8 is the official style guide for Python code, written by Guido van Rossum, Barry Warsaw, and Nick Coghlan. The name PEP stands for Python Enhancement Proposal, and PEP8 is the 8th PEP that was created to provide guidelines for writing Python code that is consistent and readable. Following PEP8 ensures that your code will be more maintainable, easier to understand, and less error-prone.
Best Practices for Writing Clean Python Code
1. Indentation
One of the most fundamental principles of PEP8 is using spaces for indentation instead of tabs. It’s recommended to use 4 spaces for each level of indentation, as this makes the code more readable across different devices and editors.
2. Line Length
PEP8 recommends that each line of code should not exceed 79 characters. This helps to prevent long lines of code that are difficult to read, especially on smaller screens or when printed.
3. Imports
Imports should be on separate lines, and each import should be placed at the top of the file. Additionally, it’s best to group imports into three categories: standard library imports, related third-party imports, and local application/library-specific imports.
4. Naming Conventions
When it comes to naming variables, functions, and classes, PEP8 recommends using lowercase letters for variable names, and words should be separated by underscores. Class names should use the CapWords convention, and function names should be lowercase with words separated by underscores.
5. Comments
Comments are an essential part of any codebase, as they provide context and explanations for the code. PEP8 suggests using inline comments sparingly and preferring docstrings for documenting modules, functions, and classes.
6. White Space
PEP8 provides guidelines for using white space in your code, such as using a single space after commas, colons, and semicolons, and avoiding extra spaces within parentheses, brackets, and braces.
7. Function Arguments
When defining function arguments, it’s recommended to use white space around the ‘=’ operator to make the code more readable. Additionally, it’s best to use descriptive names for function arguments to improve the readability of the code.
Why Mastering PEP8 is Important
Adhering to PEP8 guidelines may seem daunting at first, especially if you’re used to writing code in a different style. However, mastering PEP8 is crucial for several reasons.
Firstly, following a consistent style guide makes it easier for developers to collaborate on a project. When everyone follows the same guidelines, it’s easier to read and understand each other’s code, leading to improved productivity and less time spent on code review and debugging.
Secondly, PEP8 makes your code more maintainable in the long run. By following best practices for naming conventions, indentation, and comments, you’re creating a codebase that is easier to maintain and extend as your project grows.
Furthermore, writing clean and readable code using PEP8 guidelines can improve your credibility as a developer. Employers and open-source maintainers value developers who follow best practices and write clean, maintainable code.
Conclusion
Mastering PEP8 and adhering to its guidelines for writing clean Python code is essential for any developer who wants to create maintainable, scalable, and easily understandable code. By following the best practices outlined in PEP8, you can improve the readability and maintainability of your code, collaborate more effectively with other developers, and enhance your credibility as a skilled Python developer.
FAQs
Q: Is PEP8 a mandatory standard for writing Python code?
A: While PEP8 is not mandatory, it is highly recommended to follow its guidelines as it promotes consistency and readability across different codebases and projects.
Q: Are there tools available to automatically check for PEP8 compliance?
A: Yes, there are several tools such as flake8 and pylint that can be integrated into your development workflow to automatically check for PEP8 compliance and provide suggestions for improvement.
Q: Does PEP8 cover all aspects of writing clean Python code?
A: PEP8 primarily focuses on style and formatting guidelines. For more comprehensive best practices, it’s recommended to refer to other resources such as “The Zen of Python” and “Clean Code” by Robert C. Martin.
Q: Can following PEP8 guidelines impact the performance of my Python code?
A: Following PEP8 guidelines primarily affects the readability and maintainability of your code, rather than its performance. However, writing clean and readable code can indirectly lead to better-performing code through easier debugging and optimization.
Q: Should I refactor my existing code to comply with PEP8 guidelines?
A: While it’s not mandatory to refactor existing code, it’s beneficial to gradually incorporate PEP8 guidelines into your codebase as you work on new features or perform code maintenance. Over time, this can lead to a more consistent and maintainable codebase.