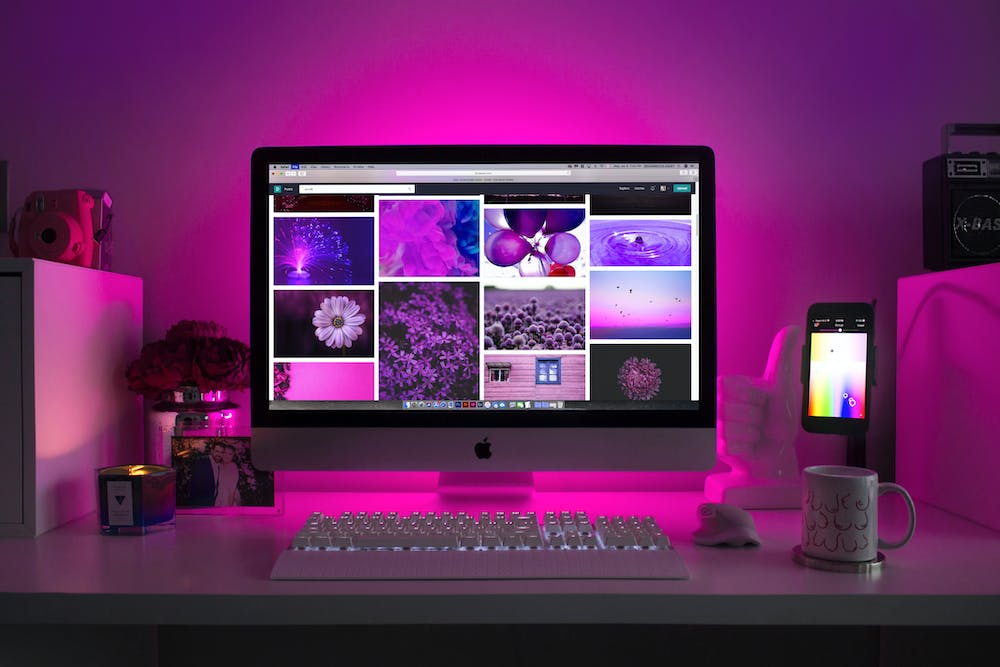
Laravel is an open-source PHP framework that provides a robust set of tools and features for building modern web applications. One of the key components of Laravel is its URL routing system, which allows developers to define clean and elegant URLs for their web application. In this article, we will explore the fundamentals of Laravel URL routing and how to master IT for seamless web development.
Understanding Laravel URL Routing
URL routing is the process of mapping a URL to a specific piece of code that handles the request. In the context of web development, this typically involves mapping URLs to different routes in the application, which are then handled by corresponding controllers or methods.
In Laravel, URL routing is defined using the routes/web.php
file, which contains a collection of route definitions. Each route definition specifies a URL pattern and the corresponding controller or closure that handles the request.
For example, the following code defines a basic route in Laravel:
Route::get('/hello', function () {
return 'Hello, World!';
});
In this example, the Route::get
method defines a route for the /hello
URL pattern, and the provided closure is executed when the URL is visited.
Creating Dynamic Routes
In addition to defining static routes, Laravel also allows developers to create dynamic routes using route parameters. Route parameters are placeholders in the URL pattern that capture segments of the URL and pass them as arguments to the route handler.
For example, the following code defines a dynamic route that accepts a parameter:
Route::get('/user/{id}', function ($id) {
return 'User ID: ' . $id;
});
In this example, the {id}
segment in the URL pattern captures the value from the URL and passes it as an argument to the route handler. This allows for creating flexible and dynamic routes in Laravel.
Named Routes and Route Groups
Laravel also supports named routes and route groups, which can help organize and manage complex routing structures. Named routes allow developers to assign a unique name to a route, making it easier to reference and generate URLs within the application.
Route groups, on the other hand, allow developers to group related routes together and apply common middleware or prefixes to the group. This can be useful for organizing and managing routes for different sections of the application, such as an admin dashboard or an API.
Middleware and Route Model Binding
Middleware is a key concept in Laravel that allows developers to filter and modify HTTP requests entering the application. Middleware can be applied to individual routes or route groups, providing a flexible way to enforce access control, validation, and other request modifications.
Route model binding is another powerful feature in Laravel that simplifies the process of injecting model instances into route handlers. This can be particularly useful for fetching and working with database records based on URL parameters, without the need for manual retrieval and validation.
Optimizing SEO with Clean URLs
URL routing plays a crucial role in search engine optimization (SEO) as well. Clean and descriptive URLs can improve the visibility and ranking of web pages in search results, making it easier for users and search engines to understand the content of a page.
By leveraging Laravel’s routing system, developers can create SEO-friendly URLs that are both human-readable and optimized for search engines. This can include using relevant keywords in the URL structure, removing unnecessary parameters, and organizing content into logical URL patterns.
Conclusion
Laravel offers a powerful and flexible URL routing system that is essential for building modern web applications. By mastering Laravel URL routing, developers can create clean, flexible, and SEO-friendly URLs that enhance the user experience and improve the discoverability of web content.
FAQs
Q: Can I use custom middleware with Laravel URL routing?
A: Yes, Laravel provides the ability to create custom middleware that can be applied to routes or route groups as needed. This allows developers to implement custom logic and filters for incoming HTTP requests.
Q: How can I optimize Laravel routes for performance?
A: To optimize route performance in Laravel, developers can consider using route caching, which pre-compiles all of the application’s route definitions into a single file, reducing the overhead of route processing during each request.
Q: What are some best practices for URL routing in Laravel?
A: Some best practices for URL routing in Laravel include keeping URLs descriptive and human-readable, organizing routes into logical groups, leveraging route model binding for database interactions, and using middleware to enforce access control and request validation.
Q: Can I generate URLs based on named routes in Laravel?
A: Yes, Laravel provides the route()
helper function, which allows developers to generate URLs based on named routes. This can be particularly useful for creating links and redirects within the application.