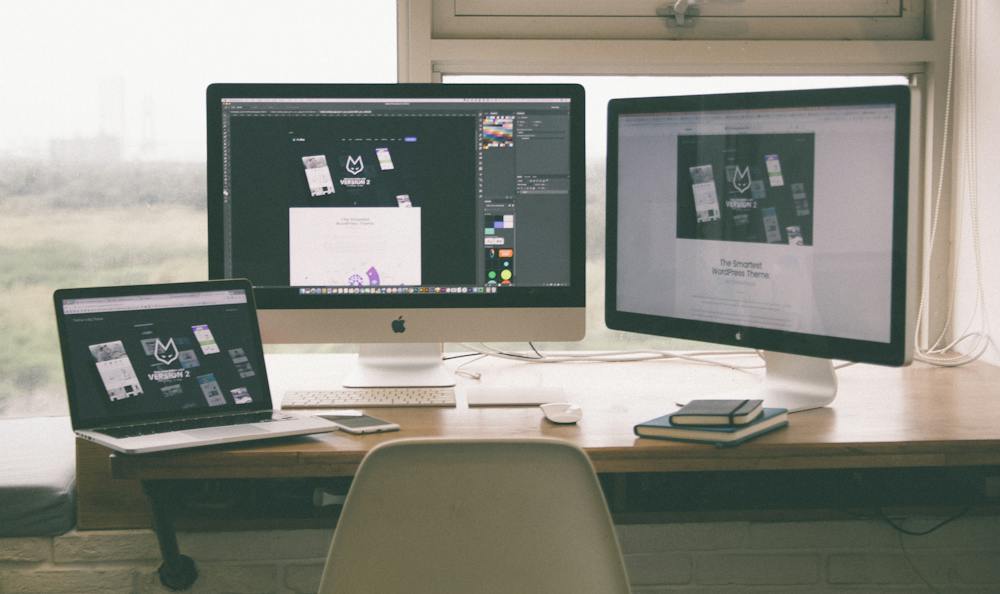
Laravel is a powerful PHP framework that has gained immense popularity among developers. IT offers a wide range of features and tools that make web development efficient and enjoyable. In this article, we will discuss some tips and tricks for mastering Laravel.
1. Use Eloquent Relationships
Eloquent is Laravel’s built-in ORM (Object-Relational Mapping) that allows developers to work with databases in a more intuitive way. One of the key features of Eloquent is its support for relationships between different database tables. By defining relationships between your models, you can easily fetch related data without writing complex SQL queries.
For example, if you have a “users” table and a “posts” table, you can define a “hasMany” relationship in the User model to fetch all the posts related to a user. This makes it much easier to work with related data and reduces the amount of boilerplate code you need to write.
Example:
“`php
class User extends Model
{
public function posts()
{
return $this->hasMany(Post::class);
}
}
“`
By utilizing Eloquent relationships, you can make your code more organized and readable, and avoid common pitfalls associated with working directly with SQL queries.
2. Utilize Laravel Mix for Asset Compilation
Laravel Mix is an elegant wrapper around Webpack for the 80% use case. By default, Laravel comes with Laravel Mix, which provides a fluent API for defining basic Webpack build steps for your Laravel application. You can easily compile your assets using Laravel Mix, and take advantage of its powerful features such as Sass and Less compilation, versioning, and cache busting.
Using Laravel Mix, you can define your asset compilation in a concise and readable way, without having to deal with the complexities of Webpack configuration. This can greatly speed up your development process and make it easier to manage your front-end assets.
Example:
“`javascript
mix.js(‘resources/js/app.js’, ‘public/js’)
.sass(‘resources/sass/app.scss’, ‘public/css’);
“`
By leveraging Laravel Mix, you can streamline your front-end development workflow and improve the performance of your web applications.
3. Take Advantage of Laravel’s Blade Templating Engine
Blade is a powerful, simple, and flexible templating engine that allows you to create dynamic, reusable components in your Laravel application. With Blade, you can easily define layouts, partials, and components, and then use them throughout your application to maintain a consistent look and feel.
Blade also provides powerful features such as template inheritance, conditional statements, loops, and more, making it easy to create complex views without writing messy PHP code. Additionally, Blade templates are compiled to plain PHP code, which ensures high performance and minimal overhead.
Example:
“`php
@extends(‘layouts.app’)
@section(‘content‘)
{{ $post->body }}
@endsection
“`
By harnessing the power of Blade, you can create elegant and maintainable views for your Laravel application, while maintaining high performance and readability.
4. Use Laravel Horizon for Monitoring and Managing Queues
Laravel Horizon is a beautiful dashboard and configuration system for managing and monitoring Laravel queues. It provides a simple, yet powerful interface for managing queue workers, monitoring job throughput, and digging into job failures. With Horizon, you can easily keep track of your queued jobs and ensure that they are being processed efficiently.
In addition to monitoring, Horizon also provides advanced features such as rate limiting and job metrics, which can help you optimize the performance of your queue system and ensure that it scales with your application.
Example:
“`php
php artisan horizon:work
“`
By incorporating Laravel Horizon into your application, you can gain valuable insights into your queue system and ensure that it is running smoothly and efficiently.
5. Leverage Laravel’s Testing Capabilities
Laravel provides robust support for testing, allowing you to write tests for your application’s features with ease. With Laravel’s built-in testing tools, you can perform unit tests, feature tests, and browser tests to ensure that your application functions as expected.
By writing tests for your application, you can catch bugs early, prevent regressions, and maintain confidence in your codebase. Laravel’s testing capabilities also integrate seamlessly with popular testing tools such as PHPUnit and Selenium, making it easy to set up and run tests for your application.
Example:
“`php
public function testBasicTest()
{
$response = $this->get(‘/’);
$response->assertStatus(200);
}
“`
By embracing Laravel’s testing features, you can ensure the reliability and stability of your application, and deliver high-quality code to your users.
Conclusion
Laravel is a versatile and powerful framework that offers a wide range of features and tools for web development. By mastering Laravel and utilizing its tips and tricks, developers can streamline their development workflow, improve their code quality, and deliver high-performance web applications to their users.
By leveraging Eloquent relationships, Laravel Mix, Blade templating engine, Laravel Horizon, and Laravel’s testing capabilities, developers can unlock the full potential of Laravel and build robust and efficient web applications.
FAQs
Q: Is it necessary to use Eloquent relationships in Laravel?
A: While it’s not mandatory to use Eloquent relationships, they greatly simplify working with related data and can help you write more expressive and maintainable code.
Q: Can I use Laravel Mix with non-Laravel projects?
A: Yes, Laravel Mix can be used with any project that uses Webpack for asset compilation, not just Laravel projects.
Q: How can I get started with Laravel testing?
A: Laravel’s official documentation provides a comprehensive guide on getting started with testing in Laravel. You can also refer to online tutorials and resources for more information.