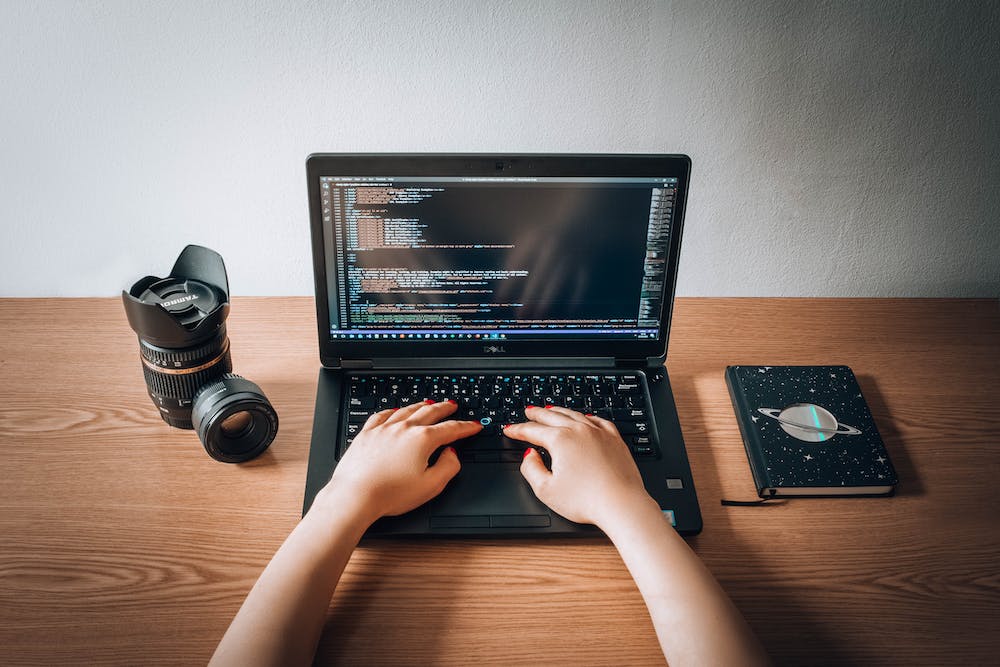
Laravel Mix is a popular tool for front-end development in the Laravel ecosystem. IT provides a clean and concise API for defining complex webpack configurations for your application. In this article, we will explore how to master Laravel Mix for efficient front-end development.
Setting Up Laravel Mix
Before we dive into using Laravel Mix, let’s first set IT up in our Laravel application. Laravel Mix comes pre-installed with Laravel, so you don’t need to install anything. However, if you are starting a new project from scratch, you can install Laravel Mix using the following command:
npm install laravel-mix --save-dev
Once Laravel Mix is installed, you can find the configuration file in the root of your Laravel application named webpack.mix.js
. This file is where you define your asset compilation settings.
Defining Asset Compilation
Laravel Mix allows you to define asset compilation in a simple and elegant way. Let’s take an example to illustrate how we can define asset compilation for JavaScript and CSS files:
// webpack.mix.js
const mix = require('laravel-mix');
mix.js('resources/js/app.js', 'public/js')
.sass('resources/sass/app.scss', 'public/css');
In the above example, we are compiling the app.js
file from the resources/js
directory and the app.scss
file from the resources/sass
directory. The compiled JavaScript and CSS files are then placed in the public/js
and public/css
directories, respectively.
Watching for Changes
One of the most powerful features of Laravel Mix is its ability to watch for changes in your assets and automatically recompile them. To do this, you can use the watch
method:
mix.js('resources/js/app.js', 'public/js')
.sass('resources/sass/app.scss', 'public/css')
.watch();
Now, whenever you make changes to your JavaScript or CSS files, Laravel Mix will automatically recompile them, saving you from having to manually run the compilation command each time.
Versioning for Cache Busting
Another useful feature of Laravel Mix is asset versioning, which can help with cache busting. To version your assets, you can use the version
method:
mix.js('resources/js/app.js', 'public/js')
.sass('resources/sass/app.scss', 'public/css')
.version();
When you run the webpack compilation with the version method, Laravel Mix will append a unique hash to the filename of each compiled asset. This can be useful for forcing browsers to download new versions of your assets when they change.
Conclusion
In conclusion, mastering Laravel Mix can significantly improve your front-end development workflow in Laravel applications. With its clean and concise API, Laravel Mix makes IT easy to define asset compilation settings, watch for changes, and version assets for cache busting. By leveraging these features, you can ensure efficient and optimized front-end development in your Laravel projects.
FAQs
Q: Can I use Laravel Mix with non-Laravel projects?
A: While Laravel Mix is designed for Laravel applications, you can also use IT with non-Laravel projects by installing IT as a standalone npm package.
Q: How can I compile assets for production?
A: You can compile assets for production by running the following command:
npm run production
This will minify and optimize your assets for production deployment.
Q: Can I customize the webpack configuration used by Laravel Mix?
A: Yes, you can customize the webpack configuration by modifying the webpack.mix.js
file. Laravel Mix provides a clean API for defining webpack configuration, but you can also tap into the underlying webpack configuration if needed.