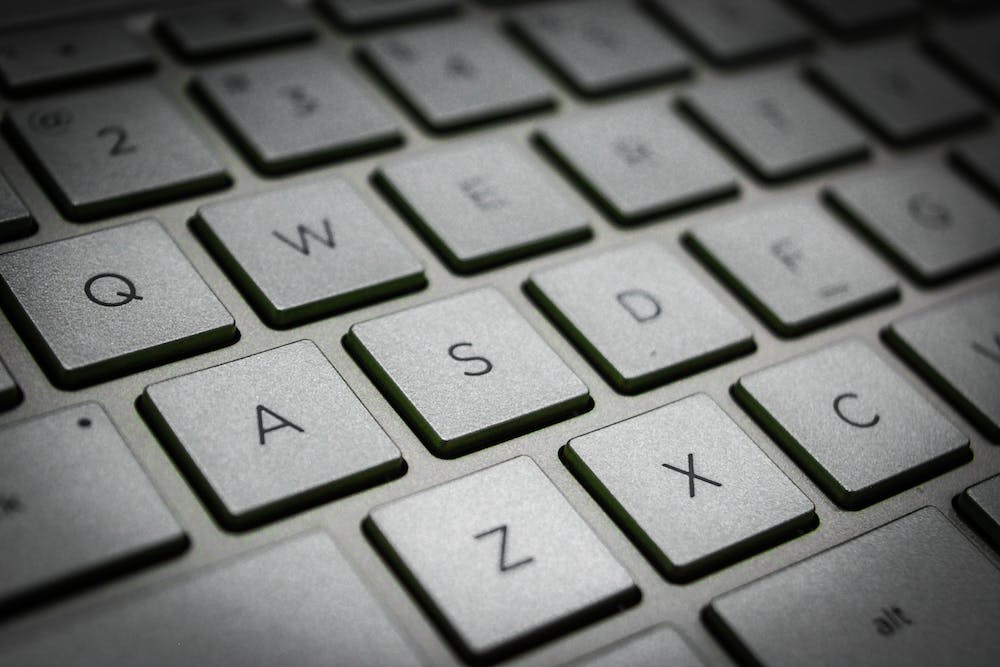
Laravel, a popular PHP framework, comes with a powerful tool called Cron. Cron allows you to automate tasks and boost productivity by scheduling repetitive tasks to run at specific intervals. In this article, we will delve into the world of Laravel Cron and learn how to master IT for optimal efficiency.
Understanding Cron Jobs
Cron is a time-based job scheduler in Unix-like computer operating systems. It enables users to schedule jobs (commands or scripts) to run periodically at fixed times, dates, or intervals. In the context of Laravel, Cron allows you to automate tasks such as sending emails, generating reports, updating database records, and more.
Setting Up Laravel Cron
To get started with Laravel Cron, you need to set up a cron job on your server. First, open the terminal and navigate to your Laravel project’s root directory. Next, run the command crontab -e
to edit the cron table. Add the following line to the cron table:
* * * * * cd /path-to-your-project && php artisan schedule:run >> /dev/null 2>&1
Replace /path-to-your-project
with the actual path to your Laravel project. This cron job runs the Laravel scheduler every minute, and the scheduler then executes any tasks that are due.
Defining Scheduled Tasks
Now that the Laravel scheduler is set up, you can define scheduled tasks in the App\Console\Kernel.php
file. In this file, you will find a schedule
method where you can define your tasks using a fluent interface. Let’s take a look at an example:
protected function schedule(Schedule $schedule)
{
$schedule->call(function () {
// Your task logic here
})->daily();
}
In this example, we define a task that runs daily. You can also specify other frequencies such as hourly, weekly, monthly, or even a custom interval using the everyMinute()
method.
Handling Task Output
When a scheduled task runs, it may produce output or errors. By default, Laravel sends task output and errors via email to the server’s system administrator. However, you can customize how the output is handled by using the sendOutputTo()
and emailOutputTo()
methods. For example:
$schedule->command('emails:send')
->daily()
->sendOutputTo('/path/to/logfile.log')
->emailOutputTo('[email protected]');
In this example, the output of the emails:send
command will be sent to a log file and email. This allows you to easily monitor task output and take action if any issues arise.
Optimizing Task Scheduling
As your application grows, you may find yourself defining numerous scheduled tasks. To keep your scheduled tasks organized, you can group them using the group()
method. For example:
$schedule->group('reporting', function ($group) {
$group->command('reports:generate')
->daily()
->sendOutputTo('/path/to/reports.log');
$group->command('reports:clean')
->dailyAt('23:59')
->sendOutputTo('/path/to/reports.log');
});
In this example, we group all reporting-related tasks together. This makes it easier to manage and update related tasks, as well as organize the output generated by these tasks in one place.
Monitoring and Debugging
After setting up your scheduled tasks, it’s important to monitor their execution and debug any issues that may arise. Laravel provides a convenient command-line tool for viewing scheduled tasks and their next run times:
php artisan schedule:work
This command runs the Laravel scheduler every minute, just like the cron job we set up earlier. It’s especially useful for testing and debugging your scheduled tasks to ensure they’re running as expected.
Conclusion
Laravel Cron is a powerful tool that allows you to automate tasks and boost productivity in your web applications. By understanding how to set up and define scheduled tasks, handle task output, optimize scheduling, and monitor and debug tasks, you can harness the full potential of Laravel Cron to streamline your application’s workflow and save time and effort.
FAQs
Q: Can I run Laravel Cron on shared hosting?
A: Yes, you can run Laravel Cron on shared hosting as long as your hosting provider supports cron jobs. Check with your hosting provider to see if they offer cron job functionality.
Q: How do I handle long-running tasks in Laravel Cron?
A: For long-running tasks, you can use the runInBackground()
method to run the task in the background, allowing your scheduled tasks to continue running without waiting for the long-running task to complete.
Q: What are some common tasks that can be automated using Laravel Cron?
A: Common tasks that can be automated using Laravel Cron include sending reminder emails, generating daily reports, updating cache data, and purging old records from the database.
Q: How can I test my scheduled tasks in development?
A: In the development environment, you can use the schedule:work
command to run the Laravel scheduler and test your scheduled tasks before deploying them to the production environment.