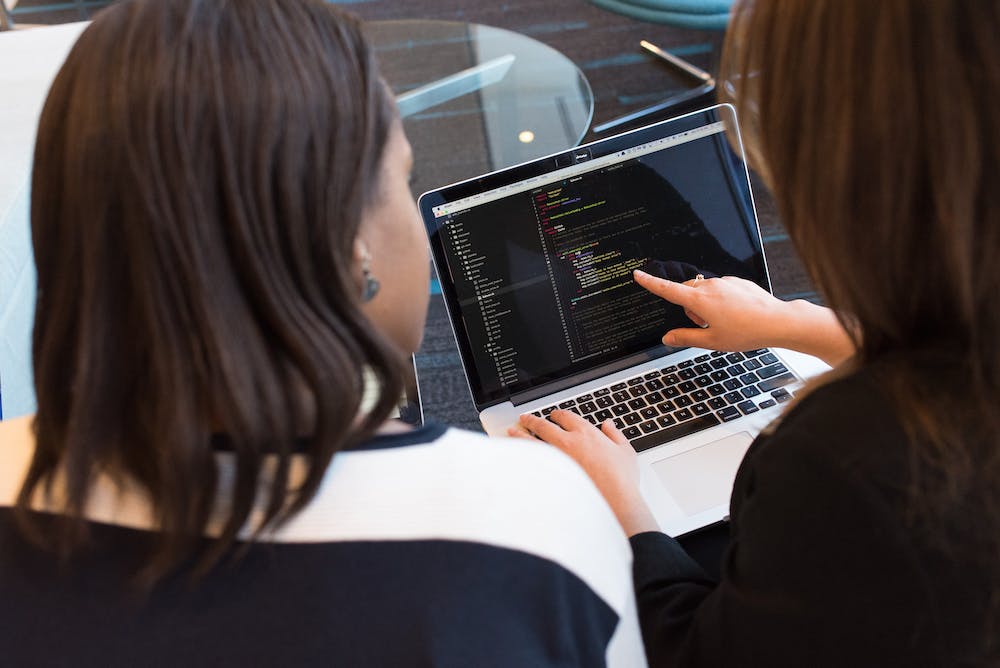
JavaScript is a versatile and powerful programming language that is widely used for creating interactive and dynamic websites. IT allows developers to build sophisticated web applications with ease. One of the key features of JavaScript is its ability to handle asynchronous tasks and delays, which can be essential for an efficient web development process.
In this article, we will explore various delaying techniques in JavaScript and how to master them for optimal performance and user experience.
Understanding Asynchronous JavaScript
Before diving into delaying techniques, it’s important to understand the concept of asynchronous JavaScript. JavaScript is a single-threaded language, meaning it can only execute one operation at a time. As a result, long-running tasks can block the thread, causing the entire application to freeze, resulting in a poor user experience.
Asynchronous JavaScript allows developers to perform tasks without blocking the main thread. This is achieved through various techniques such as callbacks, promises, and async/await. By leveraging asynchronous JavaScript, developers can create responsive and efficient web applications.
Delaying Execution with setTimeout
One of the most commonly used delaying techniques in JavaScript is the setTimeout function. This function allows developers to delay the execution of a piece of code by a specified amount of time. The syntax for setTimeout is as follows:
setTimeout(function() {
// code to be executed after the delay
}, 1000); // 1000ms delay
With setTimeout, developers can create delays to perform animations, update data, or execute any other task that requires a time delay. It’s important to note that the delay specified in setTimeout is not guaranteed due to the single-threaded nature of JavaScript. Therefore, developers should use caution when relying on precise timing with setTimeout.
Debouncing and Throttling
Debouncing and throttling are delaying techniques commonly used in scenarios where frequent events, such as scrolling or typing, can trigger rapid and potentially resource-intensive operations. Debouncing and throttling help optimize performance by limiting the frequency of these operations.
Debouncing
Debouncing is a delaying technique that limits the rate at which a function is called. It is often used in scenarios where an event, such as scrolling or resizing the window, triggers a function that can be resource-intensive. Debouncing ensures that the function is only called after a certain period of inactivity, allowing for more efficient resource usage.
Throttling
Throttling is similar to debouncing, but it limits the rate at which a function can be called to a maximum number of times per second. This is particularly useful in scenarios where rapid and frequent events can lead to performance issues, such as handling mousemove or touchmove events.
Delayed Function Execution with Promises
Promises are a powerful feature of JavaScript that allow developers to work with asynchronous code in a more structured and organized manner. Promises are often used to perform delayed function execution and handle asynchronous tasks with ease.
By creating a promise, developers can delay the execution of a function until the promise is resolved. This is particularly useful in scenarios where a function depends on the completion of an asynchronous task, such as fetching data from an API or reading from a file.
function fetchData() {
return new Promise((resolve, reject) => {
// asynchronous task to fetch data
// resolve with the data once the task is complete
});
}
fetchData().then(data => {
// execute code that depends on the fetched data
});
By utilizing promises, developers can create more resilient and maintainable code that handles delays and asynchronous tasks with ease.
Conclusion
Mastering delaying techniques in JavaScript is essential for creating responsive and efficient web applications. By understanding asynchronous JavaScript and leveraging delaying techniques such as setTimeout, debouncing, throttling, and promises, developers can optimize performance and provide a seamless user experience.
FAQs
Q: Is there a difference between setTimeout and setInterval?
A: Yes, while both setTimeout and setInterval can be used to create delays in JavaScript, they function differently. setTimeout executes a function once after a specified delay, while setInterval repeatedly executes a function at a specified interval.
Q: Are there any potential drawbacks to using delaying techniques in JavaScript?
A: One potential drawback is the reliance on precise timing, which can be challenging in the single-threaded nature of JavaScript. Additionally, excessive use of delaying techniques, such as debouncing and throttling, can lead to a less responsive user interface if not implemented carefully.
Q: How can I measure the performance impact of delaying techniques in my JavaScript application?
A: There are various tools and techniques available for measuring the performance impact of delaying techniques, such as browser developer tools and performance profiling tools. It’s important to monitor and analyze the performance of your application to ensure that delaying techniques are used effectively.