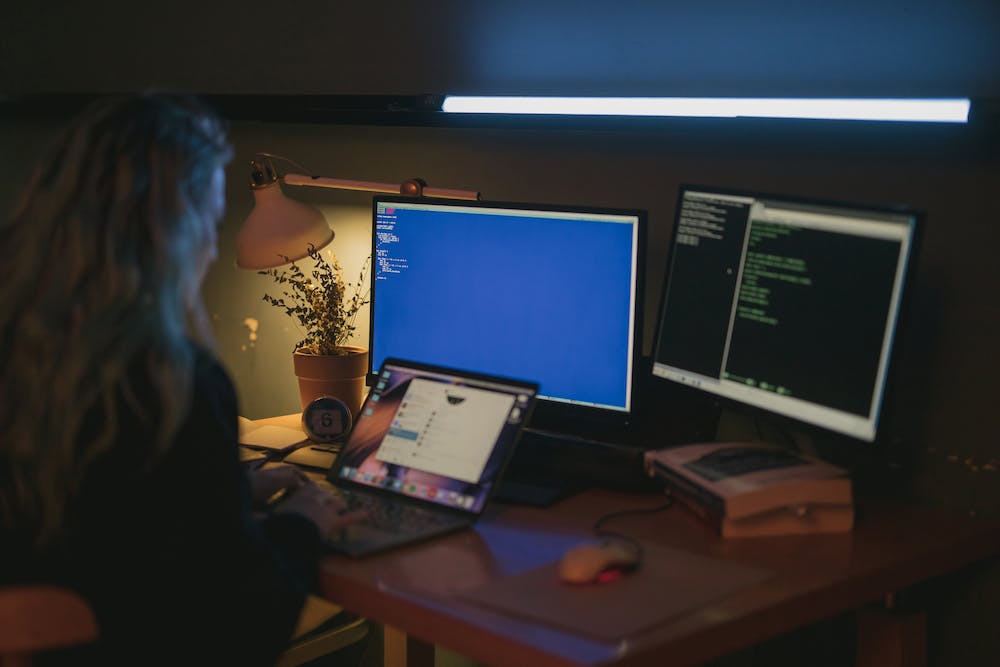
JavaScript is a powerful language that is widely used in web development. IT is an essential skill for any web developer, and mastering IT can open up new possibilities for creating dynamic and interactive web applications. In this article, we will share some tips and tricks for advanced users to take their JavaScript skills to the next level.
Use Strict Mode
One of the first tips for advanced JavaScript users is to always use strict mode. Strict mode is a way to opt into a restricted variant of JavaScript, which makes debugging and fixing mistakes much easier. IT helps catch common coding errors and prevents the use of undeclared variables, which can lead to unexpected results.
“`javascript
“use strict”;
“`
Functional Programming
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. In JavaScript, you can take advantage of functional programming techniques to write more concise, maintainable, and readable code. Functions like map, filter, and reduce are powerful tools that every advanced JavaScript user should be familiar with.
“`javascript
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map(num => num * 2);
“`
Asynchronous JavaScript
Asynchronous JavaScript is a common feature in modern web development, and advanced users should have a deep understanding of asynchronous programming. Promises, async/await, and the event loop are essential concepts in asynchronous JavaScript, and mastering them can help you build faster and more responsive web applications.
“`javascript
async function fetchData() {
const response = await fetch(‘https://api.example.com/data’);
const data = await response.json();
return data;
}
“`
Optimize Performance
Performance optimization is crucial for web applications, and JavaScript plays a significant role in this area. Advanced users should be familiar with techniques like code splitting, lazy loading, and caching to improve the performance of their JavaScript applications. Understanding the performance implications of different coding patterns and library choices can make a big difference in the user experience of your application.
“`javascript
import { lazy } from ‘react’;
const LazyComponent = lazy(() => import(‘./LazyComponent’));
“`
Testing and Debugging
No matter how skilled you are in JavaScript, testing and debugging are essential parts of the development process. Advanced JavaScript users should be familiar with tools like Jest, Mocha, and Karma for testing, as well as debugging tools like Chrome DevTools and the Node.js debugger. writing testable code and debugging efficiently can save a lot of time and frustration in the long run.
“`javascript
describe(‘Calculator’, () => {
test(‘adds two numbers’, () => {
const result = add(1, 2);
expect(result).toBe(3);
});
});
“`
Conclusion
Mastering JavaScript is a continuous journey, and advanced users should always be open to learning new techniques and best practices. By using strict mode, embracing functional programming, understanding asynchronous JavaScript, optimizing performance, and honing testing and debugging skills, advanced users can take their JavaScript expertise to the next level.
FAQs
Q: What are some good resources for further learning JavaScript?
A: There are many great resources available for learning JavaScript, including online courses, books, and documentation. Some popular options include the Mozilla Developer Network (MDN), JavaScript: The Good Parts by Douglas Crockford, and online platforms like Codecademy and Pluralsight.
Q: How can I stay updated with the latest developments in JavaScript?
A: Following influential developers and organizations on social media platforms like Twitter, joining JavaScript communities and forums, and attending conferences and meetups are great ways to stay updated with the latest developments in JavaScript.
Q: Is IT necessary to learn a JavaScript framework like React or Angular?
A: While IT‘s not necessary to learn a JavaScript framework, doing so can open up more opportunities and make you a more versatile developer. IT‘s always beneficial to have a broad skill set in different tools and technologies.