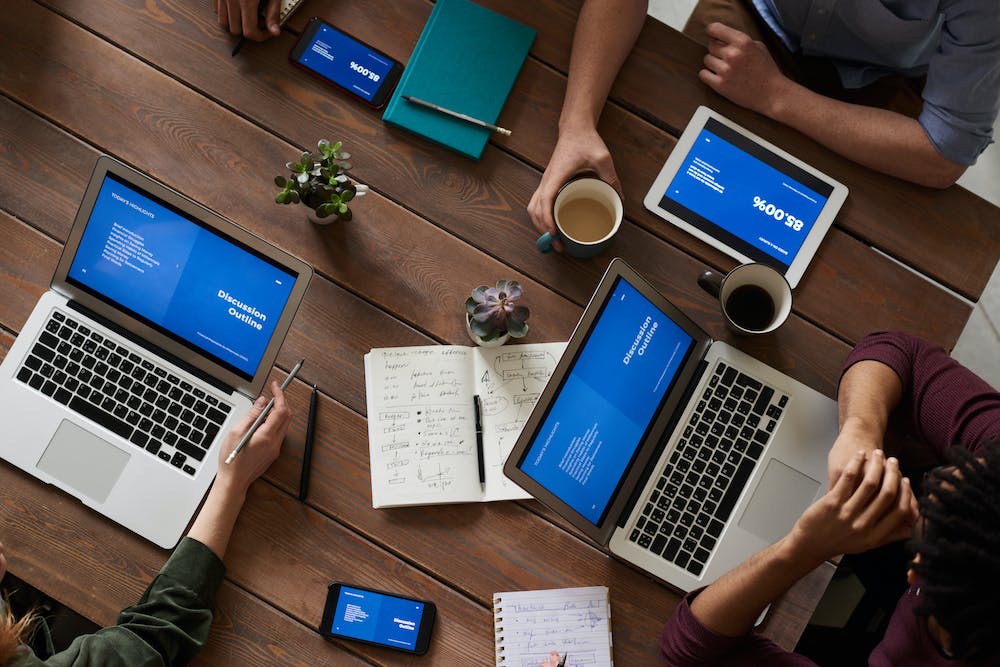
content=”width=device-width, initial-scale=1.0″>
JavaScript lists, or arrays, are fundamental data structures that allow developers to store and manipulate collections of values efficiently. Whether you’re a beginner or an experienced JavaScript programmer, understanding how to work with lists is essential for building dynamic and interactive web applications.
In this in-depth guide, we will dive deep into mastering JavaScript lists and explore various techniques, methods, and tips to improve your array manipulation skills. By the end, you’ll have a comprehensive understanding of lists in JavaScript and be able to leverage their power to develop better and more efficient code.
Creating Lists
Before we start exploring the intricacies of list manipulation, IT‘s important to understand how to create lists in JavaScript. There are multiple ways to initialize an array:
Literal Notation:
const myArray = [1, 2, 3, 4, 5];
Array Constructor:
const myArray = new Array(1, 2, 3, 4, 5);
Accessing List Elements
Once you have created a list, you need to know how to access its individual elements. In JavaScript, list elements are accessed using their index, which starts at 0 for the first element. Here’s an example:
Accessing Elements:
const myArray = [1, 2, 3, 4, 5];
const firstElement = myArray[0]; // returns 1
const thirdElement = myArray[2]; // returns 3
Modifying List Elements
To modify the values within a list, you can simply assign new values to specific indices. Here’s an example:
Modifying Elements:
let myArray = [1, 2, 3, 4, 5];
myArray[0] = 10; // changes the first element to 10
myArray[3] = 7; // changes the fourth element to 7
List Manipulation Methods
JavaScript provides a rich set of methods that allow you to manipulate and transform lists efficiently. Let’s explore some commonly used methods:
1. push()
This method appends one or more elements to the end of a list.
const myArray = [1, 2, 3];
myArray.push(4); // adds 4 at the end
myArray.push(5, 6); // adds 5 and 6 at the end
2. pop()
This method removes the last element from a list and returns IT.
const myArray = [1, 2, 3, 4, 5];
myArray.pop(); // removes 5 and returns IT
3. shift()
This method removes the first element from a list and returns IT.
const myArray = [1, 2, 3, 4, 5];
myArray.shift(); // removes 1 and returns IT
4. unshift()
This method adds one or more elements to the beginning of a list.
const myArray = [1, 2, 3];
myArray.unshift(0); // adds 0 at the beginning
myArray.unshift(-2, -1); // adds -2 and -1 at the beginning
List Iteration and Transformation
Iterating over lists and transforming their elements is a common task in JavaScript development. Let’s explore two commonly used methods for achieving this:
1. forEach()
This method allows you to execute a provided function for each element in a list.
const myArray = [1, 2, 3];
myArray.forEach((element) => {
console.log(element);
});
2. map()
This method creates a new list by applying a provided function to each element in an existing list.
const myArray = [1, 2, 3];
const doubledArray = myArray.map((element) => {
return element * 2;
});
Common FAQs
Q: How can I check if a variable is an array in JavaScript?
A: You can use the Array.isArray() method to determine if a variable is an array or not. IT returns true if the variable is an array, and false otherwise.
const myVariable = [1, 2, 3];
console.log(Array.isArray(myVariable)); // prints true
Q: Can JavaScript lists contain elements of different data types?
A: Yes, JavaScript lists can store elements of different data types. Arrays in JavaScript are dynamic and don’t impose any restrictions on the types of values they can contain.
Q: How can I remove an element from a specific index in a list?
A: You can use the splice() method to remove elements from a specific index in a list. IT takes the index as a parameter and removes the element at that index.
const myArray = [1, 2, 3, 4, 5];
myArray.splice(2, 1); // removes the element at index 2
Q: What happens if I try to access an index that is out of bounds?
A: If you try to access an index that is out of bounds (e.g., a negative index or an index larger than the list’s length), JavaScript will return undefined.
Q: Are there any built-in methods for sorting lists in JavaScript?
A: Yes, JavaScript provides the sort() method to sort the elements of a list in place based on their default string representations. However, for more complex sorting requirements, you may need to provide a custom comparison function.
Q: Can I nest arrays within arrays in JavaScript?
A: Yes, JavaScript allows you to nest arrays within arrays, creating multi-dimensional arrays. This can be useful for representing matrices, tables, or complex data structures.
Q: Is there a limit to the number of elements a JavaScript list can hold?
A: The maximum number of elements that a JavaScript list can hold is determined by the available memory and the browser’s limitations. In practice, this limit is usually very high and rarely a concern for most applications.