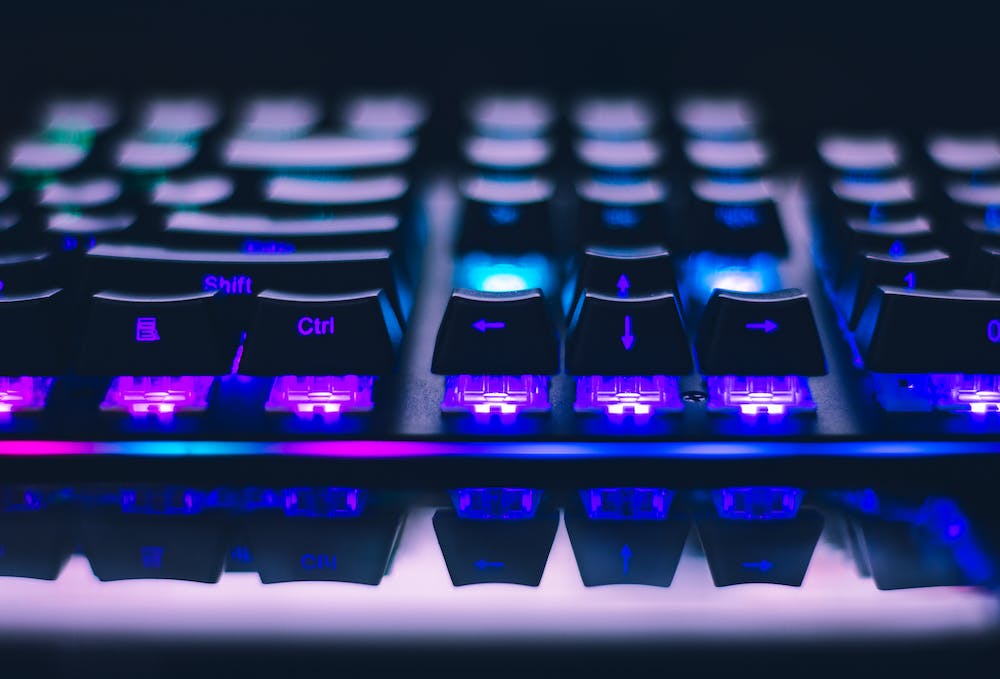
JavaScript functions are an essential part of any web developer’s toolkit. They allow us to create reusable pieces of code, making our web applications more efficient and organized.
Understanding JavaScript Functions
Before we dive into mastering JavaScript functions, let’s first understand what functions are and how they work in JavaScript. A function is a block of code that can be called and executed at any point in our code. Functions can take in parameters, perform a task, and return a value. In JavaScript, functions are first-class citizens, which means they can be passed around just like any other variable.
Creating Functions in JavaScript
To create a function in JavaScript, we use the function
keyword followed by the function name and a pair of parentheses. We can also define parameters inside the parentheses if the function requires any input. Here’s an example of a simple JavaScript function:
function greet(name) {
return "Hello, " + name + "!";
}
In this example, we’ve created a function called greet
that takes in a name
parameter and returns a greeting message. We can then call this function and pass in a name to get the desired output.
Function Expressions
In addition to the traditional way of defining functions, we can also create functions using function expressions. This involves assigning a function to a variable. Here’s an example:
const add = function(a, b) {
return a + b;
};
With function expressions, we can create anonymous functions or named functions and assign them to variables. This gives us more flexibility when working with functions in JavaScript.
Mastering JavaScript Functions
To truly master JavaScript functions, we need to explore some of the advanced concepts and techniques that can take our coding skills to the next level. Let’s delve into some of these concepts:
Higher-Order Functions
One of the most powerful concepts in JavaScript is the ability to use functions as arguments to other functions, or to return functions from other functions. These functions are called higher-order functions. They allow us to write more abstract and reusable code by operating on other functions as data.
For example, we can create a higher-order function that takes in another function as an argument and applies IT to an array of values. This can be a powerful tool for performing data transformations and processing.
Arrow Functions
ES6 introduced arrow functions, which provide a more concise syntax for writing functions. Arrow functions are especially useful for writing shorter, more readable code. Here’s an example of an arrow function:
const square = (num) => {
return num * num;
};
Arrow functions also have some differences in behavior compared to traditional functions, such as the way they handle the this
keyword. Understanding these differences is crucial for mastering JavaScript functions.
Closures
Closures are an important concept in JavaScript that allows functions to retain access to their surrounding scope even after the outer function has finished executing. This means that inner functions have access to the variables and parameters of the outer function, even after it has returned. Closures are often used to create private variables and functions in JavaScript.
Optimizing JavaScript Functions
It’s not enough to just understand the concepts and techniques of JavaScript functions. We also need to optimize our functions for performance and maintainability. Here are some tips for optimizing JavaScript functions:
Declarative Programming
Declarative programming is a programming paradigm that emphasizes the use of expressions or declarations instead of statements to define what the program should accomplish. By writing declarative functions, we can create more concise and readable code that is easier to understand and maintain.
Functional Programming
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions. By embracing functional programming principles, we can write functions that are more predictable, easier to test, and less prone to unexpected side effects.
Writing Pure Functions
Pure functions are functions that always return the same output for the same input and have no side effects. By writing pure functions, we can avoid unexpected behavior and make our code easier to reason about and test.
Conclusion
Mastering JavaScript functions is essential for any web developer looking to take their coding skills to the next level. By understanding the advanced concepts and techniques of JavaScript functions, as well as optimizing our functions for performance and maintainability, we can become more efficient and effective programmers.
FAQs
What are the different types of functions in JavaScript?
In JavaScript, there are several types of functions, including traditional functions, function expressions, arrow functions, and higher-order functions.
How can I optimize my JavaScript functions for performance?
To optimize your JavaScript functions for performance, consider using declarative and functional programming principles, writing pure functions, and avoiding unnecessary side effects.
Where can I learn more about mastering JavaScript functions?
There are many resources available online, including tutorials, articles, and documentation. backlink works, for example, offers a comprehensive guide to mastering JavaScript functions with in-depth examples and explanations.
Mastering JavaScript functions takes time and practice, but the benefits are well worth it. By understanding the concepts and techniques of JavaScript functions, and optimizing our functions for performance and maintainability, we can become more efficient and effective programmers.