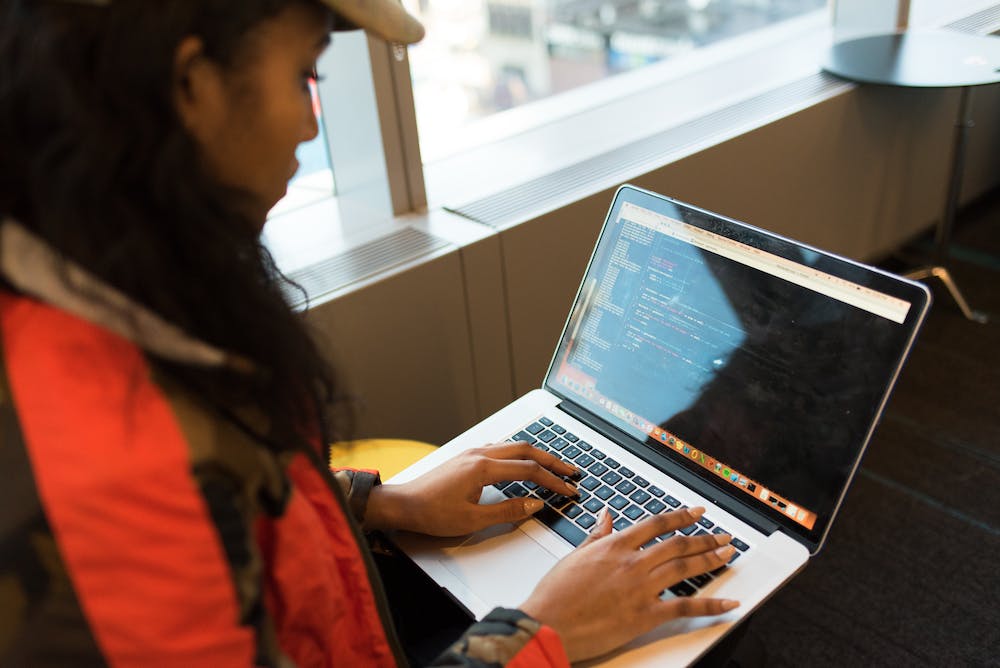
JavaScript arrays are a fundamental part of the language and are used to store multiple values in a single variable. They are highly versatile and can be manipulated in many different ways. In this article, we will discuss some tips and tricks for mastering JavaScript arrays.
Tip 1: Using the push() method to add elements
The push()
method is used to add one or more elements to the end of an array. This can be useful when you need to dynamically add elements to an array based on user input or other factors. For example:
let fruits = ['apple', 'banana'];
fruits.push('orange');
console.log(fruits); // ['apple', 'banana', 'orange']
Tip 2: Removing elements with pop() and shift()
The pop()
method removes the last element from an array, while the shift()
method removes the first element. This can be helpful when you need to remove elements from the beginning or end of an array. For example:
let numbers = [1, 2, 3, 4, 5];
numbers.pop();
console.log(numbers); // [1, 2, 3, 4]
numbers.shift();
console.log(numbers); // [2, 3, 4]
Tip 3: Using splice() to add or remove elements at specific positions
The splice()
method can be used to add or remove elements at specific positions within an array. IT takes three parameters: the index at which to start, the number of elements to remove, and the elements to add. For example:
let colors = ['red', 'green', 'blue'];
colors.splice(1, 0, 'yellow');
console.log(colors); // ['red', 'yellow', 'green', 'blue']
colors.splice(2, 1);
console.log(colors); // ['red', 'yellow', 'blue']
Tip 4: Using map() to transform elements
The map()
method creates a new array with the results of calling a provided function on every element in the calling array. This can be useful for transforming elements in an array without modifying the original array. For example:
let numbers = [1, 2, 3, 4, 5];
let doubled = numbers.map(num => num * 2);
console.log(doubled); // [2, 4, 6, 8, 10]
Tip 5: Using filter() to create a new array with filtered elements
The filter()
method creates a new array with all elements that pass the test implemented by the provided function. This can be useful for creating a subset of an array that meets certain criteria. For example:
let ages = [18, 21, 16, 25, 30];
let adults = ages.filter(age => age >= 18);
console.log(adults); // [18, 21, 25, 30]
Conclusion
Mastering JavaScript arrays is essential for any JavaScript developer. By understanding the various methods and techniques for working with arrays, you can efficiently manipulate and transform data in your applications. Whether IT‘s adding or removing elements, transforming values, or filtering data, JavaScript arrays provide a powerful toolset for managing complex data structures.
FAQs
1. What are some common array methods in JavaScript?
Some common array methods in JavaScript include push()
, pop()
, shift()
, unshift()
, splice()
, map()
, and filter()
.
2. How can I check if an array contains a specific element?
You can use the includes()
method to check if an array contains a specific element. For example:
let fruits = ['apple', 'banana', 'orange'];
if (fruits.includes('banana')) {
console.log('The array contains banana.');
}
3. Can I use array methods in older versions of JavaScript?
Yes, most array methods are supported in older versions of JavaScript, but IT‘s always a good practice to check for compatibility when using newer methods in older environments. You can also use polyfills or transpilers to ensure backward compatibility.