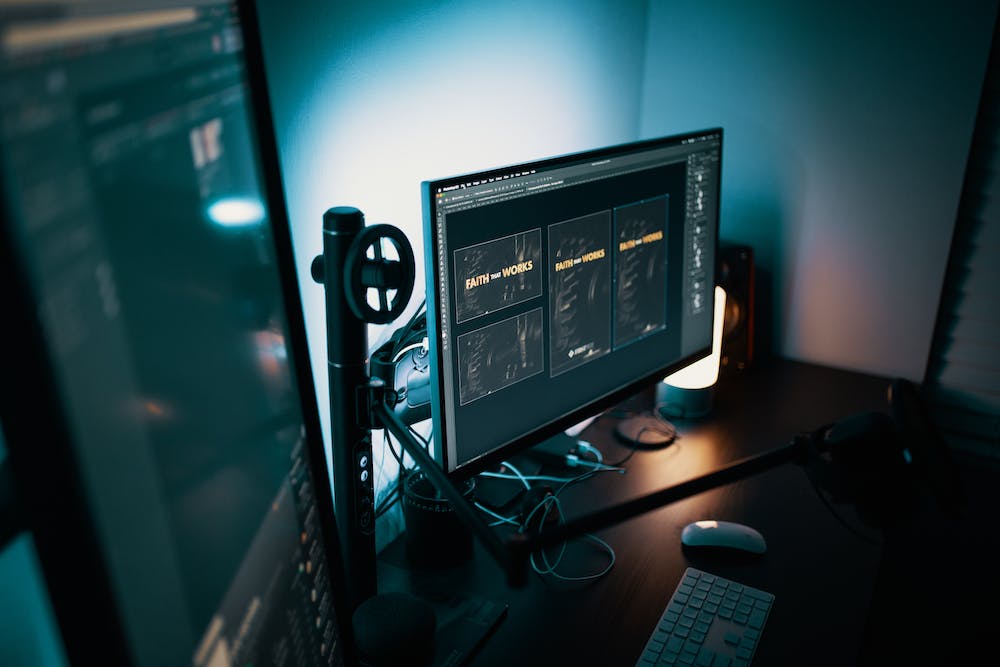
JavaScript is a powerful and versatile programming language that is essential for modern web development. Whether you are a beginner looking to improve your skills or an experienced developer wanting to master advanced techniques, this comprehensive guide will provide you with everything you need to know to become a JavaScript guru.
Understanding the Basics
Before diving into advanced techniques, IT‘s important to have a solid understanding of the basics of JavaScript. This includes understanding variables, data types, functions, and control flow. If you are new to JavaScript, IT‘s recommended to start with a beginner’s guide before moving on to advanced techniques.
Variables and Data Types
Variables are used to store data that can be manipulated and accessed throughout your code. In JavaScript, variables can be declared using the var, let, or const keywords. IT‘s important to understand the differences between these keywords and when to use each one.
Data types in JavaScript include numbers, strings, booleans, arrays, and objects. Understanding how to work with these data types is essential for mastering JavaScript.
Functions
Functions are a fundamental building block of JavaScript. They allow you to encapsulate a set of instructions and reuse them throughout your code. JavaScript functions can be declared using the function keyword, arrow functions, or the new ES6 syntax. Understanding the different ways to declare and use functions is crucial for mastering JavaScript.
Control Flow
Control flow refers to the order in which the statements of a program are executed. This includes conditional statements such as if-else and switch, as well as looping structures like for, while, and do-while. Mastering control flow is essential for writing efficient and effective JavaScript code.
Advanced Techniques
Once you have a solid understanding of the basics, you can begin to explore advanced techniques in JavaScript. This includes learning about topics such as object-oriented programming, asynchronous programming, error handling, and more.
Object-Oriented Programming
JavaScript is a versatile language that supports object-oriented programming (OOP) principles. This allows you to create reusable and modular code by creating objects, classes, and prototypes. Understanding OOP in JavaScript is essential for building scalable and maintainable applications.
Asynchronous Programming
Asynchronous programming is a key feature of JavaScript that allows you to execute code in a non-blocking manner. This includes working with promises, async/await, and event handling. Mastering asynchronous programming is crucial for building responsive and efficient web applications.
Error Handling
Error handling is an important aspect of writing reliable and robust JavaScript code. This includes using try-catch blocks, throwing and catching exceptions, and handling asynchronous errors. Understanding how to properly handle errors in JavaScript is essential for writing production-ready code.
Optimizing Performance
In addition to mastering advanced techniques, IT‘s important to optimize the performance of your JavaScript code. This includes topics such as code optimization, browser compatibility, and using tools such as webpack and babel.
Code Optimization
Code optimization involves writing efficient and clean JavaScript code that is easy to read and understand. This includes best practices such as avoiding nested loops, minimizing DOM manipulation, and using efficient algorithms. Optimizing your code is essential for improving the performance of your web applications.
Browser Compatibility
Browser compatibility refers to writing JavaScript code that works across different web browsers. This includes understanding the quirks and differences between browsers, as well as using tools such as polyfills and feature detection. Ensuring your code works on all major browsers is essential for reaching a wide audience with your web applications.
Using Tools
Tools such as webpack and babel are essential for modern web development. Webpack is a module bundler that allows you to bundle and optimize your JavaScript code, while babel is a transpiler that allows you to use the latest JavaScript features while maintaining compatibility with older browsers. Understanding how to use these tools is essential for modern JavaScript development.
Conclusion
Mastering JavaScript is a journey that requires dedication, practice, and continuous learning. By understanding the basics, exploring advanced techniques, and optimizing performance, you can become a JavaScript guru who is capable of building powerful and efficient web applications.
FAQs
Q: What are the best resources for learning advanced JavaScript techniques?
A: There are many resources available for learning advanced JavaScript techniques, including online courses, books, and tutorials. Some popular online platforms for learning JavaScript include Udemy, Coursera, and Codecademy.
Q: How can I stay up to date with the latest JavaScript developments?
A: Staying up to date with the latest JavaScript developments is essential for mastering the language. You can do this by following industry blogs, attending conferences and meetups, and participating in online communities such as Stack Overflow and GitHub.
Q: What are some real-world examples of advanced JavaScript techniques in action?
A: Advanced JavaScript techniques are used in a wide range of real-world applications, including web development, mobile app development, and server-side programming. Some examples include building interactive user interfaces, creating real-time web applications, and optimizing performance for large-scale web applications.