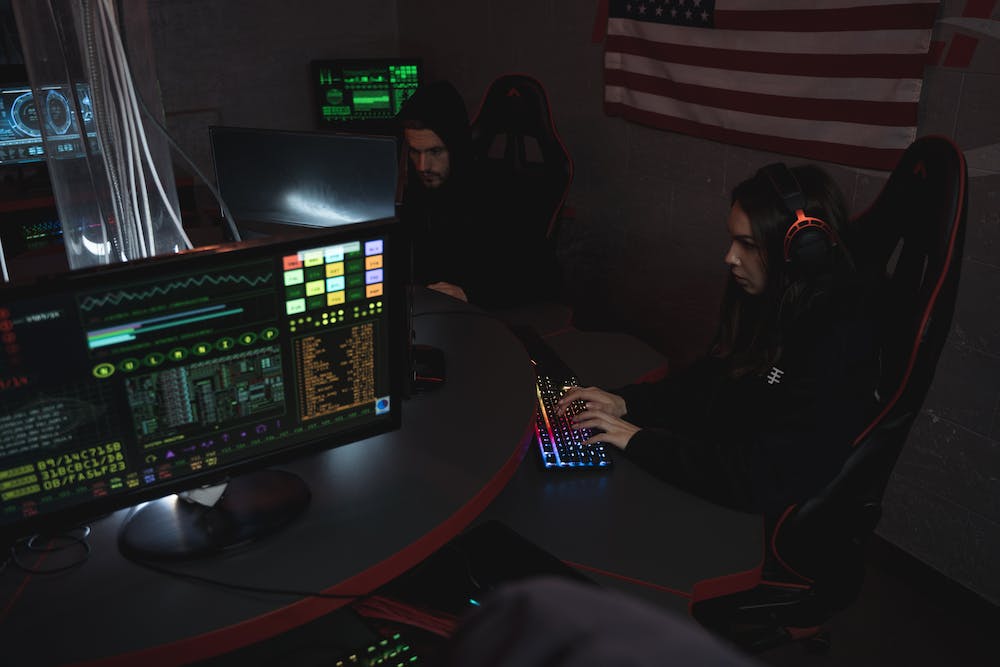
JavaScript is a powerful and versatile programming language used for creating interactive and dynamic websites. Whether you are a beginner or an experienced developer, mastering JavaScript is essential for building modern web applications and websites. In this article, we will explore 15 code examples that every JavaScript developer should know in order to master the language.
1. Variables and Data Types
One of the basics of JavaScript is understanding how to declare variables and work with different data types. Here’s an example:
// Declare a variable
let name = "John";
// Display the variable
console.log(name);
2. Functions
Functions are essential for organizing code and performing tasks. Here’s an example of a simple function:
// Define a function
function greet(name) {
return "Hello, " + name + "!";
}
// Call the function
console.log(greet("World"));
3. Arrays
Arrays allow you to store multiple values in a single variable. Here’s an example of how to work with arrays:
// Create an array
let fruits = ["apple", "banana", "orange"];
// Access an element in the array
console.log(fruits[0]);
// Add an element to the array
fruits.push("grape");
// Remove an element from the array
fruits.pop();
4. Objects
Objects are used to store collections of key-value pairs. Here’s an example of an object:
// Create an object
let person = {
name: "John",
age: 30,
occupation: "Developer"
};
// Access a property of the object
console.log(person.name);
Conclusion
Mastering JavaScript is a continuous learning process. By understanding and practicing these 15 code examples, you will be well on your way to becoming a proficient JavaScript developer. Keep experimenting, learning, and building to enhance your skills and take your projects to the next level.
FAQs
Q: Is JavaScript difficult to learn?
A: JavaScript can be challenging for beginners, but with dedication and practice, IT can be mastered.
Q: Are there any resources for further learning?
A: Yes, there are numerous online tutorials, documentation, and courses available for mastering JavaScript.