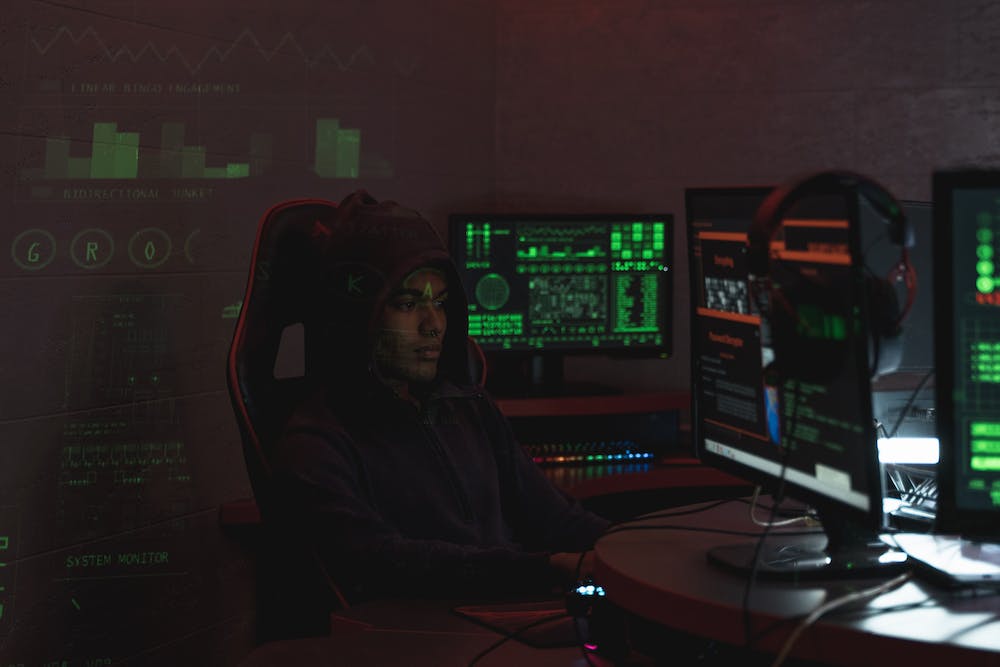
C is one of the oldest and most widely used programming languages. IT is a powerful, high-level language that is used to develop system software, application software, and even hardware drivers. Mastering C is essential for anyone looking to pursue a career in programming or software development.
Understanding the Basics of C
Before diving into writing basic programs in C, it’s important to have a solid understanding of the basics of the language. C is a structured programming language that allows for variable and function declaration, conditional expressions, loops, and other basic programming concepts.
One of the key features of C is its ability to directly access and manipulate memory, making it a powerful language for system programming. However, this also means that C can be prone to errors and bugs if not written carefully.
Writing Basic C Programs
To master C, it’s essential to start with writing basic programs. This can include simple programs such as printing “Hello, World!” to the console, or more complex programs that involve mathematical operations, loops, and conditional statements.
Let’s take a look at a simple “Hello, World!” program written in C:
#include
int main() {
printf("Hello, World!\n");
return 0;
}
In this program, we use the #include
directive to include the standard input/output header file, stdio.h
. We then define a main()
function, which is the entry point of a C program. Inside the main()
function, we use the printf()
function to print “Hello, World!” to the console, and then return 0 to indicate successful program execution.
Best Practices for Writing C Programs
When writing basic programs in C, it’s important to follow best practices to ensure the code is clean, readable, and free of errors. Some best practices for writing C programs include:
- Use meaningful variable and function names
- Comment your code to explain its purpose and logic
- Avoid using global variables
- Ensure proper indentation and formatting
- Use meaningful variable and function names
Debugging and Testing C Programs
Once you’ve written a basic C program, it’s important to debug and test it to ensure it functions as expected. C programs can be prone to errors such as segmentation faults, memory leaks, and logical errors, so it’s crucial to thoroughly test and debug your programs.
There are several debugging and testing tools available for C, such as gdb, valgrind, and clang’s AddressSanitizer. These tools can help you identify and fix errors in your C programs, making the debugging process much easier and more efficient.
Further Learning and Resources
Mastering C takes time and practice, and there are plenty of resources available to help you along the way. Books such as “The C Programming Language” by Brian W. Kernighan and Dennis M. Ritchie, online tutorials, and coding challenges can all help you improve your C programming skills.
Additionally, joining a programming community or forum can provide you with a support network of fellow C programmers who can offer advice, feedback, and guidance as you continue to learn and grow in the language.
Conclusion
Mastering C and writing basic programs in the language is a fundamental skill for anyone interested in programming and software development. By understanding the basics of C, writing clean and efficient code, and thoroughly testing and debugging programs, you can become proficient in the language and open up a world of opportunities in the tech industry.
FAQs
Q: Is C still relevant in today’s technology landscape?
A: Despite being one of the oldest programming languages, C is still widely used today, especially in systems programming, embedded systems, and low-level development. Its speed and efficiency make it a valuable language for a variety of applications.
Q: Are there any specific tools or IDEs recommended for writing C programs?
A: While there are many IDEs and tools available for writing C programs, it ultimately comes down to personal preference. Some popular choices include Visual Studio Code, CLion, and Dev-C++, but any text editor with syntax highlighting can be used to write C programs.
Q: What are some common pitfalls to avoid when writing C programs?
A: Some common pitfalls to avoid in C programming include using uninitialized variables, subverting array bounds, and not properly managing memory allocation and deallocation. It’s important to pay close attention to memory management and error handling in C programs.
Q: How can I continue to improve my C programming skills?
A: Continuously practicing and challenging yourself with more complex programs is one of the best ways to improve your C programming skills. Additionally, reading books, participating in coding challenges, and seeking feedback from the programming community can all help you grow as a C programmer.